
Why does my code produce a "Make error" when calling method on wave of waves (Make/WAVE)?
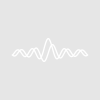
DJackson
driver_batch()
, so I have not included the gigantic monstrosity that is the the functions called in it here, just abbreviated versions. Thus, the problem seems to be in the way that I am creating and using Make/WAVE
. The specific error message in the debugger is Make error: "Can't convert a wave reference wave to or from another type." I'd really appreciate it if someone could explain what this means and why it is happening.My understanding of this is predicated on my understanding of what happens when you do
Make/N=(0, 0, 0)
and add to the second an third dimensions, which is as follows. Say you add a point to the first dimension several times times, it looks like a 3D stick or line made of cubes. Then, if you add a couple of data points to each wave in the second and third dimensions, the stick becomes a pine tree that only grows its horizontal branches in two directions. Is this accurate?#pragma rtGlobals=3 // Use modern global access method and strict wave access Function driver_batch()// use of *_batch here will allow us to use preexisting code for running script on all waves in experiment file //for spont, do something like below for stepDataAnalysis() Make /O/D/N=(0, 0, 0)/WAVE cStepCurves//dim=0: Cell number dim=1: FI dim=2: IV see display curves below for explanation stepDataAnalysis(cStepCurves)//changes sStepData DisplayCurves(cStepCurves) end //my explanation in comments below may not be particularly relevant. Function stepDataAnalysis(cStepCurves) wave cStepCurves ...//add information to cStepCurves wave. by way of Insert/M=1 /M=2 end Function DisplayCurves(cStepCurves) wave cStepCurves ...//I call Display on the second and third dimensions, separately, (which are wave objects if I understand correctly?) as each wave //represents an IV curve and FI curve (like in electrical engineering/physics or patch clamping neurons) end
I wanted to call Display on waves, which is why I am bothering with Make/Wave in the first place. Many, many thanks to you all.
/D and /WAVE are not compatible. The first creates a double precision wave, the latter a wave of references.
Does "cStepCurves" contain one wave, i.e., one data set, or several waves,i.e., a set of data sets?
In the first case, the /WAVE flag is wrong
In the second case the "wave" statements are missing the /WAVE flag and the Make operation has an extra /D:
Adding data points to a dimension alters the size of the "cuboid" shaped data set -- no branches.
HJ
Caffeine helps reading:
My guess is that you want
This would address two waves (y-dimension) for each experiment (x-dimension)
Experiment IV-curve FI-curve
1§ 4DF6 357A 4242 666F
2 946B CDF6 FD23 23A0*
....
§calculated index
*wave references
January 4, 2016 at 01:21 am - Permalink
January 4, 2016 at 11:25 am - Permalink
Make /WAVE /N=(0,2)
how can I insert points into the y-dimension for each of the two waves in the y-dimension? My best guess is something likeJanuary 4, 2016 at 09:13 pm - Permalink
Your x (actually it's "p") value is an index for your experiments. The y (actually "q") 'selects' the wave reference (q=0: FI q=1: IV)
Long story short x for the experiment, y for the waveS (sic).
HJ
January 4, 2016 at 03:03 pm - Permalink
It does not compile.
January 5, 2016 at 03:24 pm - Permalink
X refers to the rows dimension of a 1D or greater wave.
Y refers to the columns dimension of a 2D or greater wave.
The contents of any element is called the "data value".
A 2D wave has X indices, Y indices, and data values.
Sometimes with XY pairs, we call the data value of the Y wave the "Y value", but when talking about multi-dimensional waves, stick to the terminology above.
Often it is best to create a simplified, self-contained example that anyone can easily understand and execute. Here is an simplified, self-contained example that may help:
What if you don't know how many rows you will eventually have in your waves matrix and want to add to it incrementally. You might try this, which does not work:
This does not work because, when you make a wave with 0 rows and 0 columns, it is a zero-dimensional wave, not a 2D wave. To see this, execute:
This prints 0, not 2 as you might expect. The reason for this is that Igor determines the dimensionality of a wave by the highest dimension with a non-zero number of elements.
If you call InsertPoints on test, it becomes a 1D wave, not a 2D wave as you wanted.
You can fix this by creating the waves matrix with 0 rows and 2 columns:
This prints 2.
Which brings us to this solution for incrementally adding rows to the waves matrix:
January 5, 2016 at 02:59 pm - Permalink
January 5, 2016 at 03:40 pm - Permalink
You must start with a non-zero number of columns in order for the wave to be 2D.
If that does not solve the problem, post a simplified, self-contained example illustrating what you are trying to do.
January 5, 2016 at 03:45 pm - Permalink
Make/O/WAVE/N=(0,2) waves
, then doingInsertPoints/M=1 1, 1, waves
does not tell me which column I am adding to, since theN=(0,2)
means there are two columns for each row index. Or am I adding to both columns?Edit: let me get together a self-contained example. Sorry I haven't been doing that.
Edit: In the documentation for Igor 6.37, the first parameter is said to be beforeElement, which marks the point before where the numElements points are added, which seems different from what you said. The dimension is determined by the /M flag. Is the /M flag what you meant by the first parameter, or are referring to beforeElement? What you said seems to conflict directly with the documentation for Igor 6.37.
I edited your example to demonstrate the slightly different thing I am trying to do, most specifically in the :
January 5, 2016 at 05:26 pm - Permalink
It should have been
I'm still unclear whether you want a fixed number of rows and a variable number of columns or if both are to be variable. Consequently I have written the following demo to do both.
I also wrote it so that you can start with a zero-dimension wave (Make/N=(0,0)). The demo uses Redimension to make the wave 2D the first time you add a row or column.
The demo uses a numeric wave but the adding of rows and columns works with any type of wave.
The DoUpdate calls are just so you can step through this in the Igor debugger and see what the wave looks like after each added row or column. Set a breakpoint on the first DoUpdate and step through the function to the end. This will show you what happens at each step.
January 5, 2016 at 06:08 pm - Permalink
I'm still unclear whether you want a fixed number of rows and a variable number of columns or if both are to be variable. Consequently I have written the following demo to do both.
Please run the following command ONE BY ONE and have a look what happens.
You will see, that adding a new row also creates the cell in the second column once it is "reasonable".
Hope this helps,
HJ
January 6, 2016 at 01:47 am - Permalink