
RGB to HSV and HSV to RGB conversion
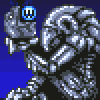
chozo
It took me way too long to figure these out, so I post them here. For RGB <> HSL you can use ImageTransform instead. Both functions use the multiple-return syntax and do not check the correctness of the input. Can be directly used with Igor R,G,B colors which have a 16 bit range. If you have 8 bit colors, simply multiply with 257 first.
// assumes R,G,B values in the range [0..65535] Function [variable H, variable S, variable V] RGB2HSV(int R, int G, int B) variable Mx = max(R,G,B), Mn = min(R,G,B) H = acos((R-G/2-B/2)/sqrt(R^2+G^2+B^2-R*G-R*B-G*B))*180/pi H = G>=B ? 360-H : H; H = numtype(H)!=0 ? 0 : H S = Mx==0 ? 0 : (1-Mn/Mx) V = Mx/65535 return [H,S,V] // outputs H = [0..360], S,V = [0..1] End // assumes a H value in the range [0..360] and S,V values in the range [0..1] Function [int R, int G, int B] HSV2RGB(variable H, variable S, variable V) variable C = V*65535, M = C*(1-S), X = (C-M)*(1-abs(mod(H/60,2)-1)) if (H >= 0 && H < 60) R = C; G = M; B = X+M; elseif (H >= 60 && H < 120) R = X+M; G = M; B = C; elseif (H >= 120 && H < 180) R = M; G = X+M; B = C; elseif (H >= 180 && H < 240) R = M; G = C; B = X+M; elseif (H >= 240 && H < 300) R = X+M; G = C; B = M; elseif (H >= 300 && H <= 360) R = C; G = X+M; B = M; endif return [R,G,B] // outputs R,G,B = [0..65535] End
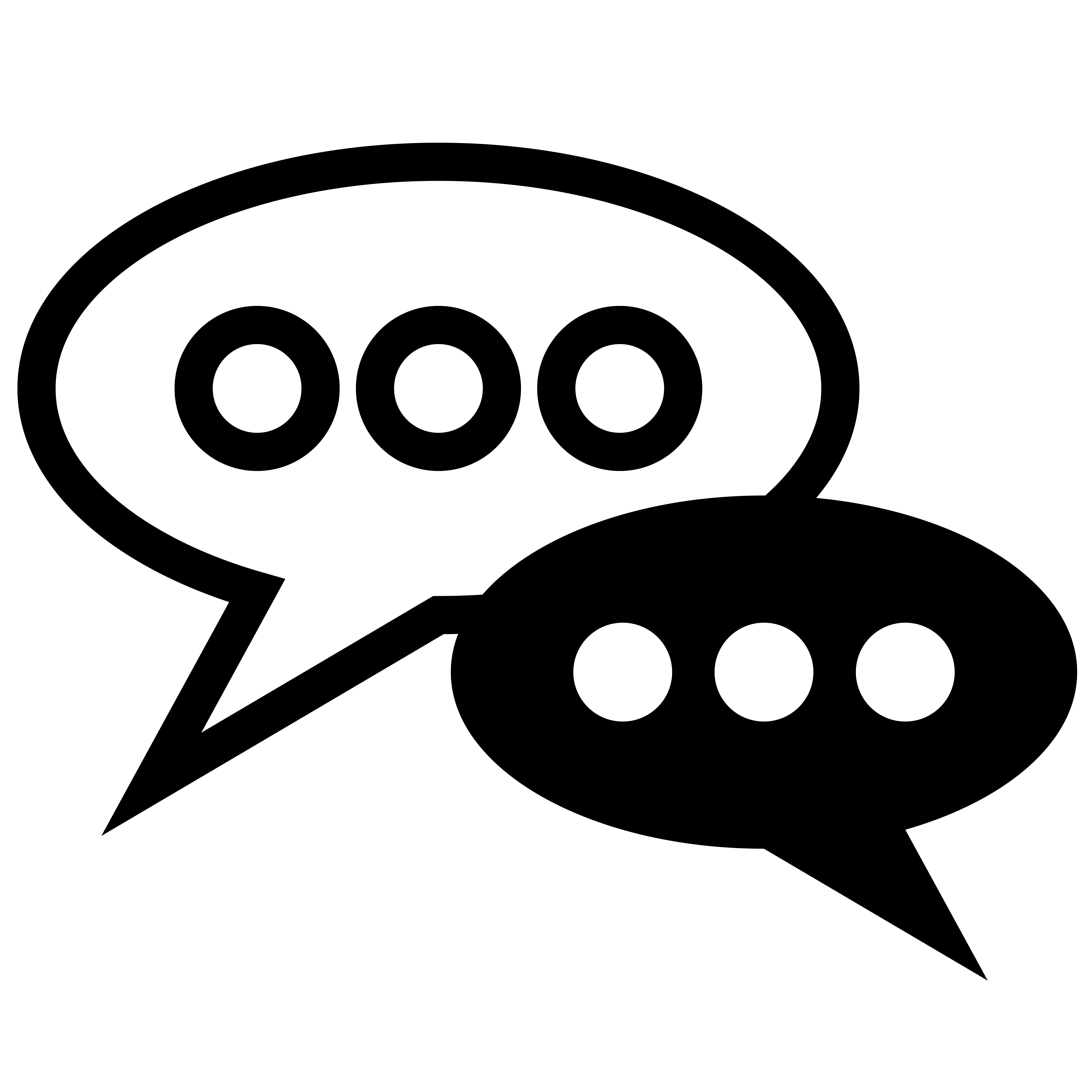
Forum
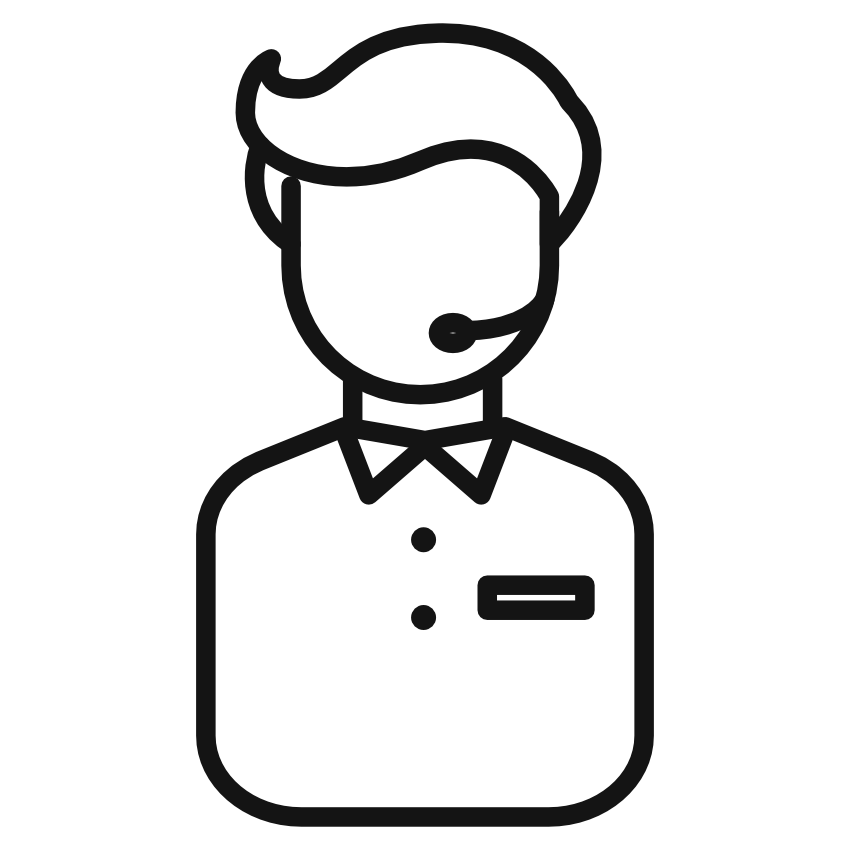
Support
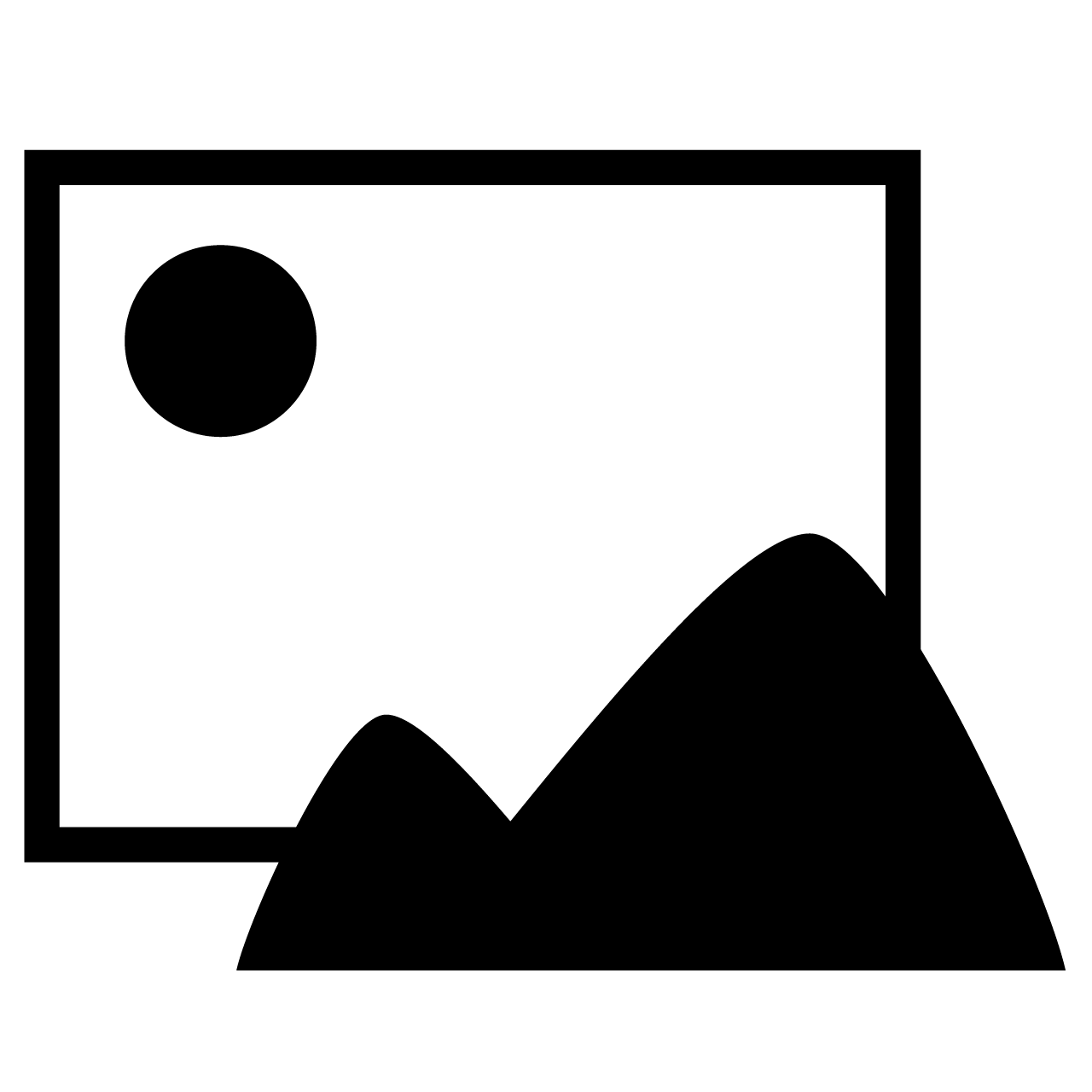
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More