
Function to get an Axis Label
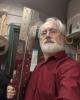
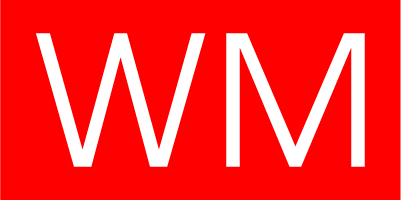
johnweeks
This function, given the name of graph ("" uses the top graph window) and the name of an axis, returns the axis label.
Function/S GetAxisLabel(gname, axisname) String gname, axisname String grecreation = WinRecreation(gname, 0) String oneLine String labelStr = "" String part1, part2 Variable i=0 do oneLine = StringFromList(i, grecreation, "\r") if (stringmatch(oneLine, "\tLabel*")) String regexp = "^\\tLabel "+axisname+" \\\"(.*)\\\"" SplitString/E=regexp oneLine, labelStr break; endif i += 1 while(strlen(oneLine) > 0) return labelStr end
The regular expression works like this:
- ^
- match the beginning of the string
- \\t
- match a tab character. The double backslash is because Igor interprets the backslash first, so to get a single backslash all the way through to SplitString, you need two of them.
- Label
- matches the literal string "Label "
- +axisname+
- matches the axis name. We hope there are no special characters in the axis name! Note that the space after the next quote mark matches the space between the axis name and the beginning of the quoted string
- \\\"
- match the opening quote on the axis label itself
- (.*)
- match all characters, and the () make it a substring which will be pulled out and put into the string variable labelStr
- \\\"
- match the closing quote
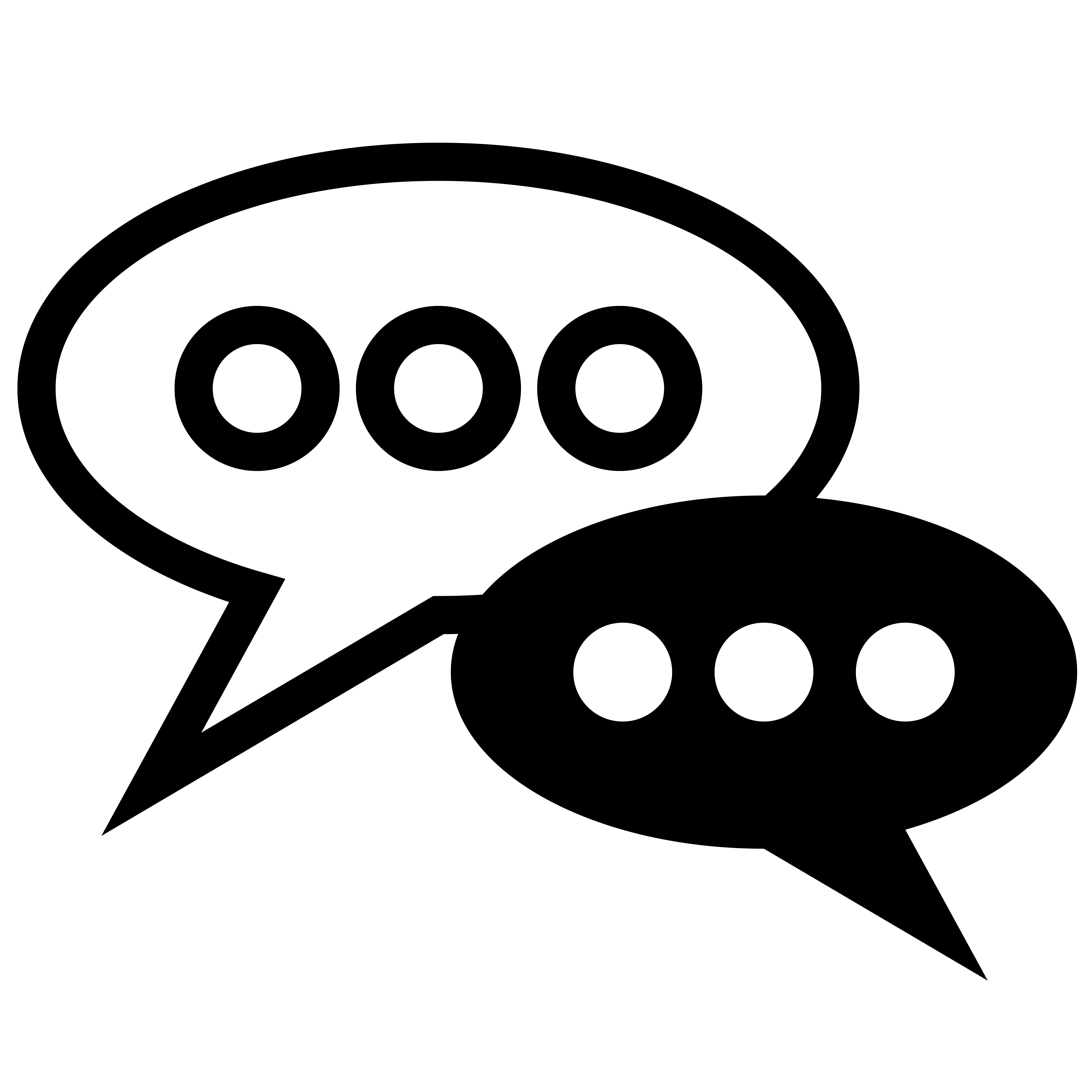
Forum
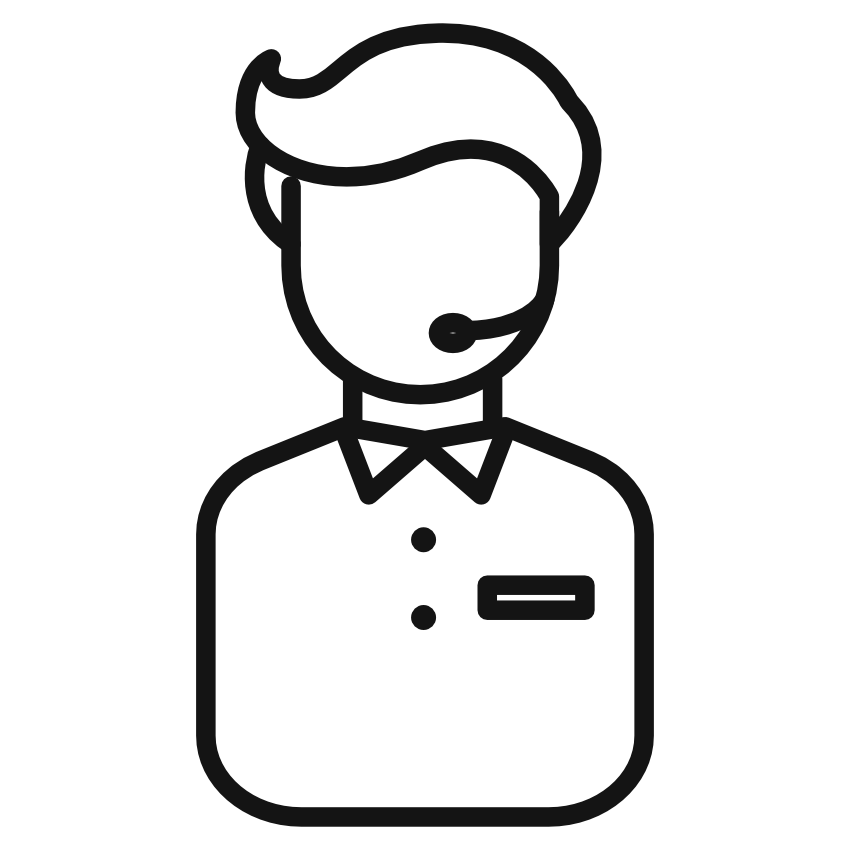
Support
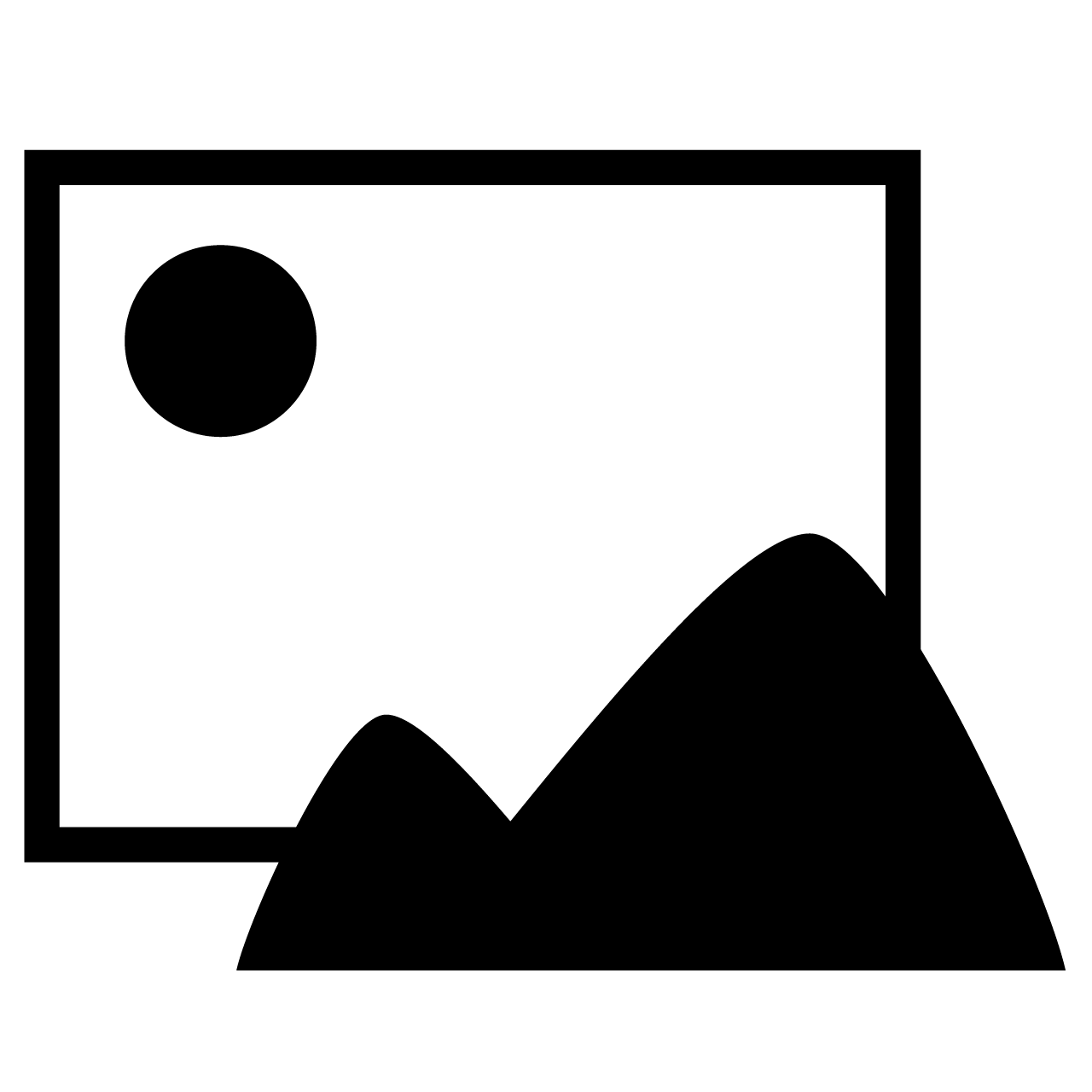
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
thanks for the hint here. I don't know why but I had to change the stringmatch line to
otherwise the returned labelStr was empty if querying the bottom axis.
Maybe this has something to do with the multiline axis labels from Igor 6.1 which I'm using?
October 7, 2009 at 06:59 am - Permalink
•make junk=x;display junk
•Label bottom "one line\ranother line"
•print GetAxisLabel("graph0", "bottom")
one line\ranother line
•display/B=bottomAxis junk
•Label bottomAxis "one line\ranother line"
•print GetAxisLabel("graph1", "bottomAxis")
one line\ranother line
I used the code I posted above without modification; as you can see, it worked correctly. If you would like, send a copy of your experiment file to support@wavemetrics.com and I'll try to figure out why the code failed on your graph.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
October 12, 2009 at 04:35 pm - Permalink
Here is an alternative that dispenses with the StringMatch altogether:
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
May 25, 2011 at 09:12 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
May 25, 2011 at 02:50 pm - Permalink
works fine for a graph with multiple axis labels (though I see it fails with labelled subgraphs) and it doesn't loop through all the lines:
Example:
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
May 26, 2011 at 08:33 am - Permalink
Needed this now for IP8 and IP9, this should be shorter:
It is not idiot proof, there are clearly many cases when it may fail.
I am really confused why there is no Igor function which would return this.
December 26, 2020 at 09:46 am - Permalink
There is an AxisLabelText user function already in
#include <Axis Utilities>
But Igor 9 will (soon) have an AxisLabel function.
December 28, 2020 at 04:46 pm - Permalink
@ilavsky: The clipboard exchange looks scary. Grep can also query text waves.
January 12, 2021 at 06:51 am - Permalink