
Prefix and/or suffix for loaded wave names in dialogs
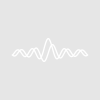
ikonen
These days I find myself using a lot of different legacy code programs that generate text output in files named "Card N" (yeah...as in legacy punch card code) with a variety of formats. If I need to run a few runs with different inputs and want to bring the data into Igor, it's just quicker and easier to use a GUI loader than writing my own function from scratch each time. Sometimes these data formats even have column headers, and I can let Igor auto-name the data, but then each data file will produce teh same names and Igor will complain about overwriting. Thus the relatively simple solution of adding a prefix or a suffix to the automatic wave names I can use to distinguish data sets. Even without column headers, adding a prefix would mean Wave0 would contain the same type of data for all my data sets. I realize many users use data folders for this, and that's always on option, but my own preference is to just work in one data folder if there aren't too many waves. It just makes all the other GUI dialogs (plotting, fitting, sorting, etc) a little quicker if all the waves are in one folder.
If you decide to put multiple data sets in one data folder, you can add a prefix or suffix to the names of freshly-loaded data by writing a procedure that takes as a parameter a semicolon-separated list of wave names and a prefix and a suffix. Pass to this function the S_waveName string generated by LoadWave and other file loaders.
Here is an untested function for doing this. Left as an exercise for the reader is the issue of dealing with name conflicts:
May 15, 2009 at 03:43 pm - Permalink
Whether or not this solution is actually easier for you to code or not is up for you to decide.
One advantage of using data folders that you might not be aware of is that Igor provides the very useful ReplaceWave operation that, if you use the allInCDF keyword, will replace all waves in a certain graph with waves with an identical name but which are in a different data folder. Depending on your workflow, this might allow you to create a graph with one set of data, and then you could duplicate that graph, and replace the data in the graph with a different set of data.
May 16, 2009 at 09:06 am - Permalink
I did not know about ReplaceWave...in the past I've achieved similar effects by saving and editing recreation macros, so that's a nice tip.
But I'll tell you why I still don't always prefer data folders. Lets say I want to plot 5 simulated spectra in the same graph. The simulations were generated in an external program which gives me 5 data files each with labeled columns "Intensity" and "Wavelength". If I load each file into 5 different data folders, and put them all in one graph, the trace names will be "Intensity, Intensity#1, Intensity#2, Intensity#3, Intensity#4". OTOH, if they are named "Intensity_He, Intensity_Ne, Intensity_Ar, etc" the trace names will match the wave names, making both command line and GUI-based trace modifications easier. Similarly, if I want to compare the data in a single table, I'll just get 5 columns labeled "Intensity".
In this particular example, I would probably just manually append the label suffix as I loaded each data set. But I was recently inspired to make this request when I was loading data from a simulation that generated 18 columns of data. I didn't need all 18 columns, but would have gladly loaded them all in order to skip the step where I edited the names. It really is just a matter of convenience.
May 16, 2009 at 12:23 pm - Permalink