
Window focus with window hook
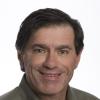
hegedus
I have an experiment for manual data review and I am trying to keep the user interaction to a minimum. The idea is to use keyboard entry only to keep their hand motion to a minimum. To that end I am using a window hook to drive the actions. I have four keys defined plus some cursor movement controlled by the arrow keys.
The issue is for one of line feed (return key) key the associated functions execute but the focus then shifts to the command window. The other keys 1, 2, or 3 work as expected but do not shift the window focus. What should I look for that is causing the shift in window focus. There is a work around if I keep the command window closed, but since I am deploying to users, I would like to avoid forcing that.
Function MyWindowHook(s) STRUCT WMWinHookStruct &s Variable hookResult = 0 // 0 if we do not handle event, 1 if we handle it. switch(s.eventCode) case 11: // Keyboard event switch (s.keycode) case 49: //1 this keeps focus definePad() setcursor(0) hookResult = 1 break case 50: //2 this keeps focus marqueeDefinePad() setCursor(0) hookResult = 1 break case 51://3 this keeps focus setCursor(1) hookResult = 1 break case 13://Line Feed this shifts focus to command window (if open) retains if closed CR_Button() hookResult = 1 break endswitch break endswitch return hookResult // If non-zero, we handled event and Igor will ignore it. End
definePad() does some edgestats and draws a boundary box on the image.
marqueeDefinePad() uses a marquee to define the drawn boundary box.
CR_Button is a little bit more involved depending on state either loading the image or recording some data and changing the axis ranges and cursor position and sets a few flags.
Any guidance?
Thanks - Andy
September 24, 2015 at 05:19 am - Permalink
I have another experiment using very similar code and the focus does not shift, so I am puzzled.
Andy
September 24, 2015 at 06:19 am - Permalink
September 24, 2015 at 06:30 am - Permalink
Andy
September 24, 2015 at 07:05 am - Permalink
what do you see? Do you by any chance get keycode 12?
Or, on Macintosh, if I do that (and comment out the call to CR_Button(), which doesn't work in my test) when I press the keypad Enter I get keycode 3. You may go crazy trying to track down all the keycodes you need to handle...
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
September 24, 2015 at 09:22 am - Permalink
My suggestion was only to comment out the call to your function not the entire case. The idea is to verify that something in your function isn't the cause of the focus shift.
September 24, 2015 at 10:26 am - Permalink
I changed the hook per your request:
Made changes: Now the "enter" does execute the function but still gives the command window focus. If I comment out the Branch with case 13:, I see the the not handled message and 13 printed to history. Other non-trapped keys show the message and key code value in history and additionally are printed to the command line.
September 24, 2015 at 12:10 pm - Permalink
Try adding case 12: to your code. It may be that you're getting carriage returns.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
September 24, 2015 at 05:13 pm - Permalink
Gave a try but no difference. Here is another bit of trouble shooting:
If I modify the case to include a print statement either before or after the CR_Button() call, I get different results
For this code: If I have the window focus on the graph and hit return. "xxxx" gets printed, the next image is loaded as intended and then the focus is shifted to the command window.
case 13://Line Feed
print "xxxx"
CR_Button()
hookResult = 1
break
If I move the print statement to after the CR_Button() call, and again with the focus on the graph and press return, the next image gets loaded, but 'xxxx" is NOT printed to history and the focus is shifted to the command window.
case 13://Line Feed
CR_Button()
print "xxxx"
hookResult = 1
break
I think I have another clue, the CR_Button function has a branched structure that can include an abort. Perhaps the abort is spanning across the the CR_Button() function to the window hook procedure. Is that possible?
September 24, 2015 at 06:03 pm - Permalink
There was an abort command indebted in a function that was called on some of the branches of CR_Button(). Abort spans all the function calls as I have now learned.
I rewrote the CR_Button() function to remove abort and now it works as intended.
Thank you for all your help.
Andy
September 25, 2015 at 02:01 pm - Permalink
I came across this thread while searching for a solution to an annoyance I have, and this background reflects exactly my feeling about what should happen: since you rarely do keyboard input in a graph, why should graph windows get the keyboard focus?
Except the feature in the quote is not what happens when you display a graph from the command window. The keyboard focus is placed in the new graph window, which is useless and counterproductive.
So I decided to comment here with my desired behavior. Maybe I should put this in the "Wish list," but it just fits so well with the quoted text above....
My opinion is that when typing in the command window, the focus should remain there as much as possible so that you can continue to type commands.
Example: displaying multiple graphs.
display wave1 vs wave0 append wave3 vs wave2 append wave5 vs wave 4 ...
Currently, you have to re-click in the command windows between each command, but that shouldn't be necessary.
October 21, 2015 at 08:08 am - Permalink
On Windows you can press CTRL+J for bringing back the focus to the command line.
October 21, 2015 at 08:15 am - Permalink
Well, when you create a new window it becomes the active window. That's how windows work.
The behavior where typing in a graph switches to the command line is, for Igor 6, a Macintosh-only feature. In Igor 7 it works on Windows, too.
In my trial, the 'append' commands do not cause the graph to be activated.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
October 21, 2015 at 12:43 pm - Permalink