
Updating SetVariable Control
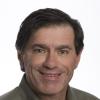
hegedus
I am using controlinfo to get the S_recreation and trying to extract the limits field. The WhichListItem requires me to know the exact string which of course is what I am trying to find. It does not allow wildcards. What is the solution I am missing? Do I just loop through the recreation string doing a stringmatch?
Andy
Or, write the settings to a userdata string attached to the panel itself, then read them back as needed?
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
March 11, 2016 at 03:23 pm - Permalink
Andy
March 11, 2016 at 05:14 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
March 14, 2016 at 05:18 pm - Permalink
March 14, 2016 at 11:49 pm - Permalink
March 15, 2016 at 02:54 pm - Permalink
March 15, 2016 at 06:41 pm - Permalink
maybe?
March 16, 2016 at 03:29 pm - Permalink
Thank you for the other options. They highlighted commands I didn't even think of.
All good options.
One last thing I also keep in mind when I code especially since this is for a client is that it needs to clear to the person maintaining the code. Or in this case the machine since it is part of the interface for positioning stage. Some while I am regexp aware but not proficient, I tend to avoid using it if a clear albeit longer method is available.
Andy
March 16, 2016 at 05:34 pm - Permalink
If other people will maintain the code, this should definitly be taken into account. If I look at your solution (as not the original coder) I would have liked to see the limit extraction functionality extracted into its own function with documentation. And most important error checking. I also woiuld have liked to see the use of new style GUI procedures as these don't rely on the top window being the panel with the control.
March 17, 2016 at 05:12 am - Permalink
Good input.
Andy
March 17, 2016 at 06:53 am - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
March 17, 2016 at 11:42 am - Permalink