
Help Parsing out a return message from a stage controller
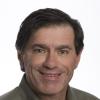
hegedus
I am attempting to interface an IAI stage system with IP7. It communicates on serial and needs a convoluted (IMHO) messaging string to communicate. In general it communicates in hex but with varying field widths and then the first character is thrown in just to mess you up.
Example: Stage move
Sprintf input, "!99234%02X%04X%04X%04X%08X",axispattern(ctrlName),Accel,decel,vel,VarNum*1000
This part works now I am trying to figure out how to parse the response specifically I want to know when it has stop moving.
The response format is
Header "#" width of 1 byte
Station "XX(h)" width of 2 bytes
Mess ID "212(h)" width of 3 bytes
Axis Pattern "XX(h)" width of 2 bytes
Axis Status "XX(h)" width of 2 bytes
... to carriage return and line feed.
I need to look at bit 0 of the Axis status field to see it is still moving. So in principle I should know where to expect the data.
Do date I have tried just getting the whole message as a text string but I am getting a bunch of unknown characters which show up as diamonds with question marks. Any suggestions?
Andy
If I look at the VTDReadHex2 it is looking for either 4 or 8 bytes. So how should I approach this?
Redimsion/E=1
?November 15, 2016 at 10:18 am - Permalink
Andy
November 15, 2016 at 12:16 pm - Permalink
November 15, 2016 at 01:13 pm - Permalink
To see each byte's value expressed as decimal and hex, execute:
November 15, 2016 at 03:53 pm - Permalink
Typically when I do serial communications I have always taken the strings or numbers as a group ending with the terminators and I guess that works for the vast majority of cases. For this case where I need to pick a particular value out of the message can I (and how would) just get the piece I need and throw away the rest. For example in the message I need bytes 9 and 10 taken as a hex number and then I can do a test for the value either in bit 0 or bit 4. So in this case would this work?
Read 8 bytes using VDTreadbinary /s=8 dummyVariable // throw away
Read 2 bytes using VDTreadHex2 realVariable
Read the rest using VDTread2 /T="\n\r" dummyString //Throw away and clear out the rest of the message
Andy
November 16, 2016 at 07:14 am - Permalink
* read the entire line to the EoL character (storage is cheap, r/w is slow, and who knows what you might need later vs now)
* parse out the required content from the line in a clever HEX or BYTE or BIT or ... space.
As for the second step, I might wonder if you could use a bit/byte-mask and do bit/byte-operation-math. I think for example
Mostly I wonder if, with some clever coding in HEX or BYTE or whatever space, you can avoid doing some other complicated or back-and-forth conversions just to test the input value.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
November 16, 2016 at 08:18 am - Permalink
It would if bytes 8 and 9 were a two-digit hex number. But I see nothing in http://www.igorexchange.com/files/screenshot.png to indicate that is the case. My guess is that it is a two-byte binary number.
The "4C" that appears in the Data Browser looks like a two-byte hex number, but it is not at byte position 8 and 9.
November 16, 2016 at 09:55 am - Permalink
I did a quick test. I had an earlier version of my experiment that was developed using IP6.3 I talk to the stage controller and get responses from it. The response are all text without any missing characters. See attached screenshots and the IAI Response field.
Is there a bug in the VDT2 code for IP7?
Andy
December 1, 2016 at 08:33 am - Permalink
Possibly but this would not be my first suspicion.
First, separate the VDT2 issue from the IAI Response control issue as follows:
1. Try setting the IAI Response field using a SetVariable command from the command line to what you think it should say and see if you can get it to misbehave. If so, post the simplest possible complete example.
2. Write the simplest possible function that gets the response from the instrument and print it to the history. If it misbehaves then print the response to the history as hexadecimal using the Test function that I posed on November 15. Then post the output from the Test function.
Since Igor7 uses Unicode to store text, if the instrument is returning non-ASCII text, you will need to convert it to UTF-8 using ConvertTextEncoding.
December 1, 2016 at 09:03 am - Permalink
The system controller has a test call feature where it just returns the same message you send. Previously when I was using IP6.3 this worked as expected.
I issue this command to the controller Input
!992003563429147
The response without the ConvertTextEncoding command
#N�0�5Ӣ2N�7�j
With it the response variable is empty (0 length)
December 1, 2016 at 09:37 am - Permalink
Now, if you will run the Test function posted above, we can see what bytes those erroneous characters represent.
I would also like to see the dump from a simpler test string, such as "ABC".
December 1, 2016 at 10:25 am - Permalink
The serial communications had been switched to 2 stop bits instead of 1. It is still odd to me that communication to the controller worked fine, but not back. I am far from an expert in serial communications.
Andy
December 5, 2016 at 08:10 pm - Permalink
The serial communications had been switched to 2 stop bits instead of 1. It is still odd to me that communication to the controller worked fine, but not back. I am far from an expert in serial communications.
Andy
December 5, 2016 at 08:10 pm - Permalink