
Return a Date|Time Stamp
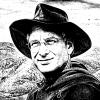
jjweimer
Function/S DateTimeStamp([sep]) string sep string a, b, c, dstr, tstr string gExp if (ParamIsDefault(sep)) sep = "" endif gExp = "([0-9]+):([0-9]+)" SplitString/E=(gExp) secs2time(datetime,2), a, b tstr = a + b gExp = "([0-9]+)/([0-9]+)/([0-9]+)" SplitString/E=(gExp) secs2date(datetime,-1), a, b, c dstr = c[2,3] + b + a return (dstr + sep + tstr) end
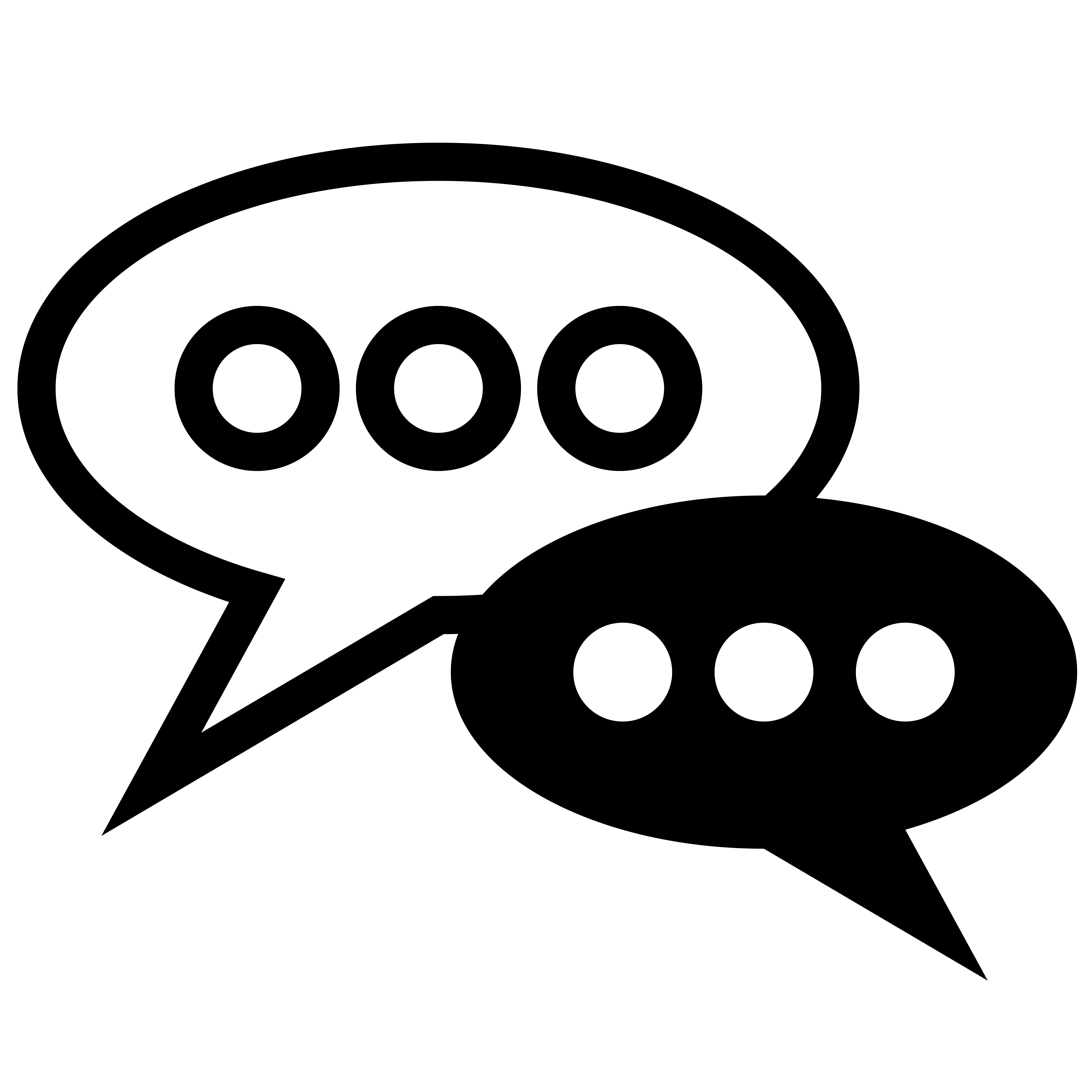
Forum
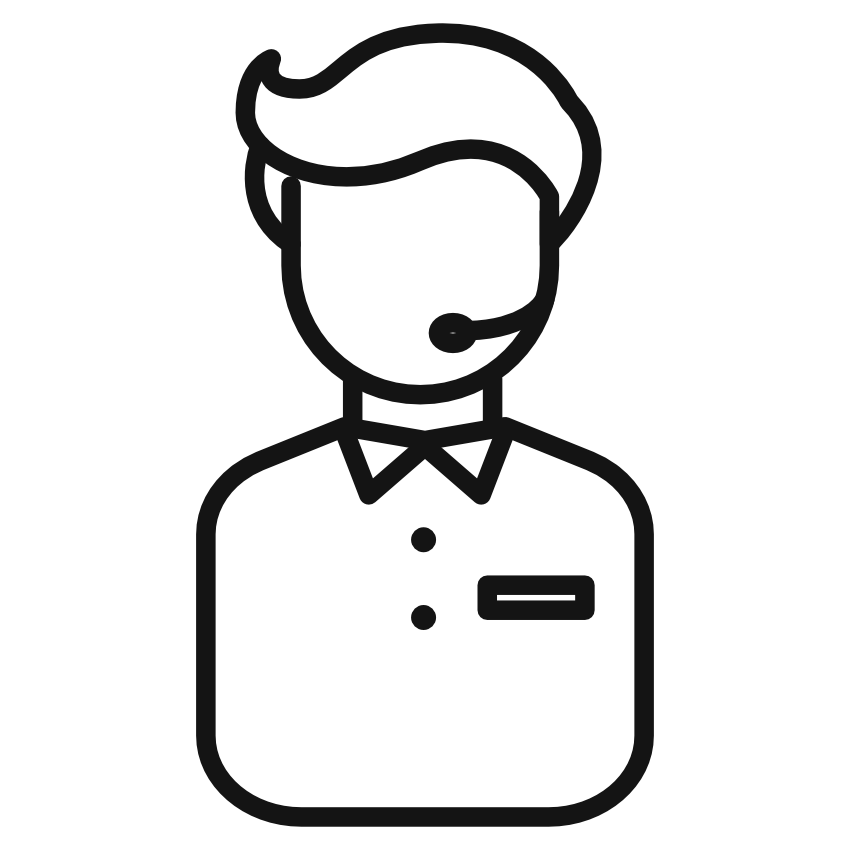
Support
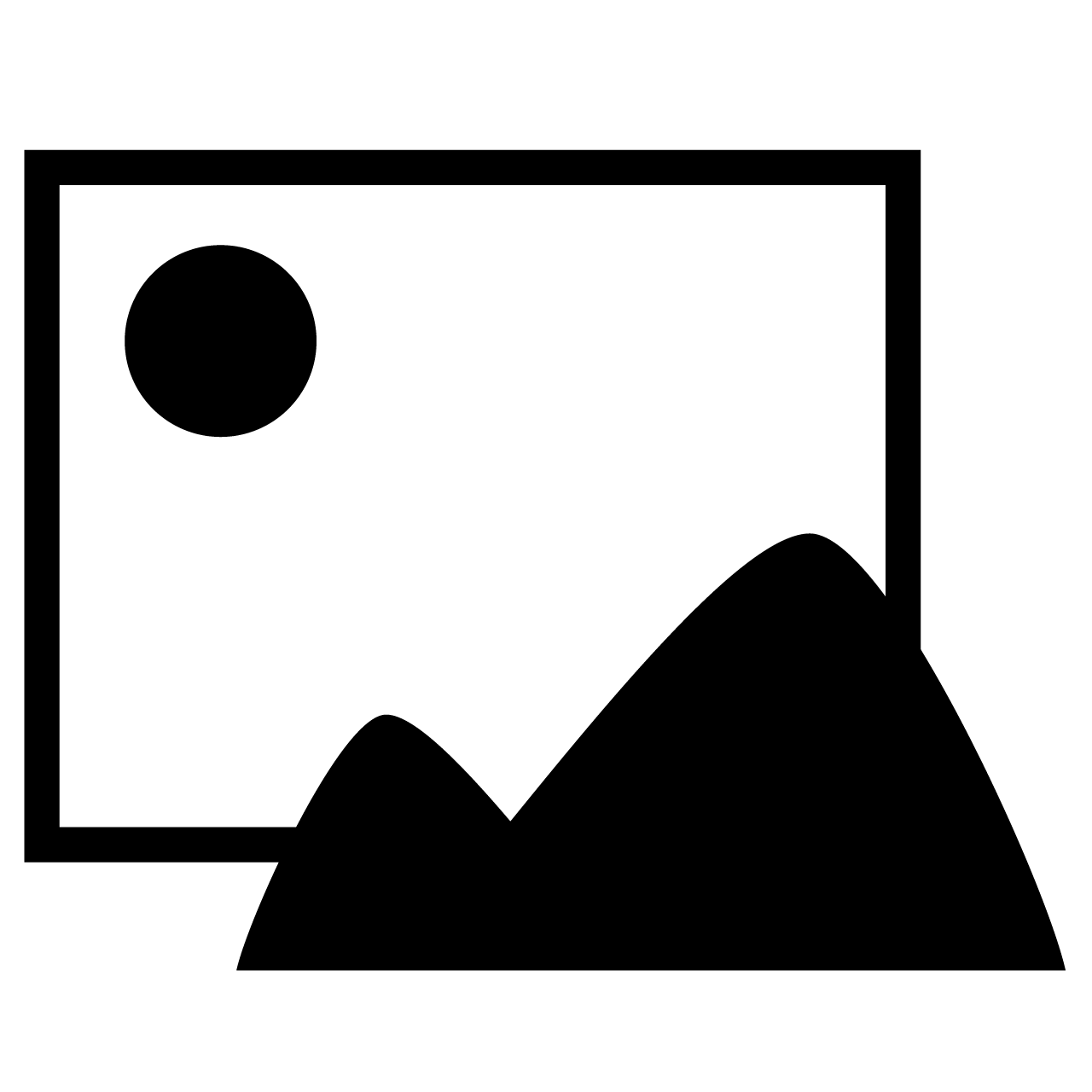
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
For computer logging (not the best visually the best way of doing it) is:
091207203350 OR yearmonthdayhourminutesecond
This is because the time/date stamp can be sorted numerically. Also, since the string is a fixed length the individual parts can be obtained with a substring, i.e.
string year = mydatestring[0,1]
December 7, 2009 at 01:39 am - Permalink
A feature request for Igor Pro is to have functions such as year(), month(), day([inyear/inmonth]), hours([24/12]), minutes(), and seconds() that return respective string or numerical values independent of platform and operating system.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
December 7, 2009 at 07:19 am - Permalink
It has the advantage of working using any time/date system settings, though the disadvantage is that the month strings are in English.
For the various year(), month(), etc. functions, you could use these:
I think if we were to improve the ability to format date and time strings, instead of creating new year(), month(), etc. built in functions, it would be better to create a function like PHP's date() function, which, while complicates, allows you to format a date/time in pretty much any way imaginable.
December 7, 2009 at 09:30 am - Permalink
Thanks.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
December 7, 2009 at 10:06 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
December 8, 2009 at 08:42 am - Permalink