
How to properly use GetRTError?
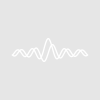
thomas_braun
yesterday I fixed a nasty bug regarding stale run time errors (RTE).
Consider the following example:
#pragma rtFunctionErrors=1 Function DoStuff() DebuggerOptions enable=0 variable a = str2num("a") a = str2num("123") print GetRTErrMessage() variable err = GetRTError(1) print err End
which gives
•dostuff() Str2num;expected number 1001
Due to "rtFunctionErrors" the first str2num generates an RTE. The next str2num suceeds, but not clears the RTE.
The RTE seems to not be cleared by a successfull operations.
This greatly "uglyfies" error handling as I have to do:
// clear err = GetRTError(1) // call operation a = str2num("str") if (GetRTError(1)) // handle error endif
My example is contrived but if error generation and error checking have 10 function calls depth in between it is not so obvious.
Is the ugly approach really the only correct one?
You can also use the AbortOnRTE keyword within a try...catch block. For example:
March 3, 2017 at 07:50 am - Permalink
March 3, 2017 at 02:41 pm - Permalink