
Loading and renaming
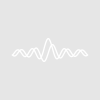
Dolbash
Moke_PermalloyWires__4_27_17_PermalloyWires1_1e_Ch1 .
Right now my code just names them wave0, wave1, etc..
I would like it to extract part of this flie name, namely anthying past the date and before the " _ch1". Mainly in this case im interested in any combniation of permalloywires1_1e but most interested in the "1e" part, since from run to run, this value changes when i take data( 1e,1f,1g, 1h, 2a, 2b,2c....). I am having a really frustrating time doing this. Part of the problem is that all of my files have the first 2 ROWS of the file occupied by non numeric data"....see below.
Experiment_SampleType _Date_ RunName Time Constant (Ms) Sensitivity Coupling Reserve Ch1 Output Ch2 Output Reference Freq (Hz) Step Size(A) Power(mw) Notes 1Avg
Moke_PermalloyWires_ _5-2-17_ SNR_1 300 50.000000 AC Low Noise X Y 2449 0.100000 3 Theta=0.doublet, Phi=2, Allwood . CW. Vary Power. SNR intent 1.000000
-2.000000 3.140259
-1.900000 3.475952
-1.800000 3.038025
-1.700000 3.666687
-1.600000 3.129578
-1.500000 3.172302
-1.400000 3.054810
From this file i only want the second column of numbers. the first column and first two rows are reference stuff that I don't need immediately.I have used the loadwave function to extract the part of the data I want, but I am having a really hard time figuring out this renaming procedure.
#pragma rtGlobals=3 // Use modern global access method and strict wave access. #include <Waves Average> function loaderOdd() //initialize loop variable variable i=0 string wname,fname //wave names and file name, respectively //Ask the user to identify a folder on the computer getfilefolderinfo/D //Store the folder that the user has selected as a new symbolic path in IGOR newpath/O DataAnalysis S_path //Create a list of all files that are .txt files in the folder. -1 parameter addresses all files. string filelist= indexedfile(DataAnalysis,-1,"????") //Begin processing the list do //store the ith name in the list into fname = stringfromlist(i,filelist) If (stringmatch(fname ,".DS_Store")) //skip mac file sorting file i=i+1 Fname = stringfromlist(i,filelist) endif variable length length = strlen(fname)-5 String extname= fname[25,length] //arbitrary name that cuts off the _ch1 part of name but probably maintains the important parts //String columnInfoStr = "" //columnInfoStr += "N= extname;"....tried a /b flag, but i think iwas implementing it improperly, nothing changed //LoadWave/A/B=columninfostr/D/j/L={0,2,0,1,0}/N=/O /P=DataAnalysis stringfromlist(i,filelist) LoadWave/j/D/A=wave/P=DataAnalysis/O/L={0,2,0,1,0} stringfromlist(i,filelist)//obviusly this is where im stuck with wave0,wave1.... Print "Loaded" + S_filename i =i+2 //move to next file while(i<itemsinlist(filelist)) //end when all files are processed. end
thank you in advance
It takes in a string like the one you show, and returns the part that is just before "_Ch", backing up to the second underscore before that. Note that this makes some assumptions about what's in the string!
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
May 4, 2017 at 12:56 pm - Permalink
If you use /B, omit /W.
Here is an example that loads just one column:
http://www.igorexchange.com/node/7171
To specify names for multiple columns, the columnInfoStr would need to be something like:
"N=<name for column 0>;N=<name for column 1>;"
If the wave names are liberal, you need single quotes:
"N='<liberal name for column 0>';N='<liberal name for column 1>';"
You can skip a column like this:
"N='_skip_';N='<liberal name for column 1>';"
Here is an example that programmatically builds up the columnInfoStr parameter:
http://www.igorexchange.com/node/6409
When you try this, start with simple names, like:
After you get that to work, customize it like this:
where baseName is extracted from your file name and GetColumnName is a function that you write which knows your naming convention.
May 4, 2017 at 01:10 pm - Permalink
May 8, 2017 at 07:21 am - Permalink
An alternative is to let Igor name the wave however it likes, then rename the wave afterwards, especially since you are only loading one column. When LoadWave completes, it generates the list string S_wavenames that you can use for the rename. Since S_waveNames is technically a list, it contains a semicolon even if it only contains one item, so you can use the function StringFromList to pull out just the string you want.
In your code:
You may want to use some of the tricks others mentioned to get the right string into "extname", and be aware that Igor can't handle very long wave names. Another useful function is PossiblyQuoteName, which will add single quotes to your string if needed.
May 8, 2017 at 08:35 am - Permalink
Yes. For example:
May 8, 2017 at 09:31 am - Permalink