
Appending strings to a textwave??
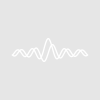
Dolbash
I have this program that, pretty much, fills a wave full of numbers that are each associated with a wave in root:. Ex. I have 20 waves in root, i generate 20 new numbers, each associated with a wave in root:. the way I do this is Not too tricky but the coode is a mess to look at so I don't want to put it here :(. In common language, I use a do-while loop to iterate with a counter and each time the counter runs, the next slot in the destination wave is filled, corresponding to the counter, using wave[i] = sourcewave[0], where imax corresponds to the number of source waves.
to the meat of the question though.
as this fills a master wave up with numerical values, I also want to fill a text wave up with the names of the source waves so I will have one wave that looks like this.
masterWave
3423
545
346
734
57746
8356
373
and a corresponding text wave that looks like this
master textwave
name0
name1
name2
name3
name4
name5
name6
The problem I am running in to is that frmulating text waves is not the same as doing so for normal waves and it seems like I must know what is going to be in all of the spots and can't simply make a wave and fill it with each iteration in a do-while loop. Any input on this would be greatly appreciated. I can certainly answer questions to clarify, and I can also put my ugly code up, but that's hopefully last resort :D
thanks in advance
cory
That's untested but you should get the idea from that.
June 13, 2017 at 11:55 am - Permalink
June 13, 2017 at 12:23 pm - Permalink
You can then use
NameOfWave(MyWaves[i])
to get the names at any point later on, if you for any reason need the names.June 13, 2017 at 11:30 pm - Permalink
June 14, 2017 at 06:59 am - Permalink