
number casting to type
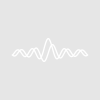
_sk
I needed to cast numbers to specific types (i.e. int16, uint16, etc.) so I wrote the following code, which may be an indication of a bug or an oversight on my behalf. Could you please verify either way?
structure struct_type char byte uchar ubyte int16 int16 uint16 uint16 int32 int int32 int32 uint32 uint uint32 uint32 float float float flt16 double double double flt32 endstructure function cast(var, type) variable var string type struct struct_type r strswitch(type) case "byte": r.byte = var; var = r.byte break case "ubyte": r.ubyte = var; var = r.ubyte break case "int16": r.int16 = var; var = r.int16 break case "uint16": r.uint16 = var; var = r.uint16 break case "int": case "int32": r.int32 = var; var = r.int32 break case "uint": case "uint32": r.uint32 = var; var = r.uint32 break case "flt16": case "float": r.float = var; var = r.float break case "flt32": case "double": r.double = var; var = r.double break endswitch return var end
And compare the results of:
•variable a •a = cast(-5, "uint16"); print a 65531 •a = cast(-5, "uint32"); print a 0
I would expect the second call (
a = cast(-5, "uint32")
) to set variable a
to: 4294967291
, the 2 complement of -5.
matrixop
January 17, 2018 at 01:24 pm - Permalink
Thanks for letting me know about this feature in IP7. It is way more pleasant to the eye like that.
While we are on the topic, does the uint32 struct field also return/ store 0 in the case of -5 in IP7 as well?
best,
_sk
January 18, 2018 at 02:34 am - Permalink
January 18, 2018 at 03:26 am - Permalink