
Checking memory usage by Igor
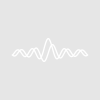
euaruksakul
Hello
We are using Igor for analysis of hyperspectral data, each set being about 300-400 MB in size. After loading many scans, the size of an Igor experiment can easily go up to a few GB, and that can cause problems with the computer sometimes. Is there a command in Igor to check for the current size of Igor experiment, so that our function automatically warns users when it becomes larger than a set limit?
We have a function to 'delete raw data' to lighten the RAM usage after finishing data reduction of each scan, but that has to be done manually (after users decide the data reduction is successful).
Thank you!
Best
Chanan
It would be helpful to know what version of Igor you are using. My guess is Igor Pro 6.xx, 32-bit version. If you were running the 64-bit version of Igor Pro 8, you would probably not need to do any memory checking.
The only reliable way to check this is to try to create a wave of a certain size. For example:
November 25, 2018 at 04:36 am - Permalink
I would check the free memory at good points, and if that is too low do your cleanup. I've posted https://www.wavemetrics.com/code-snippet/get-free-memory-gb for getting the free memory.
November 26, 2018 at 08:31 am - Permalink
In Igor 7 and later, the string returned by WaveInfo contains the size of the wave in the SIZEINBYTES keyword. You could walk your data tree to calculate the total amount of data used by waves in your experiment. Or, if the experiment is saved on disk, you could look at the size of the file itself with GetFileFolderInfo. Igor experiments don't compress the data contained in the experiment, so the size of an experiment file should roughly correlate with the amount of memory used when an experiment is loaded.
November 26, 2018 at 09:13 am - Permalink
With SIZEINBYTES keyword, I made a code for folder size calculations.
February 12, 2021 at 11:38 am - Permalink