
Removing rows with missing values
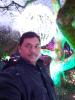
op1111shukla
Hello All, so I have a data file with 100 columns and 2000 rows. In some rows some of the cells don't have values and are blank. Is there an easy way to delete these rows?
Thanks
To remove NaNs from 1D numeric waves you can do
WaveTransform zapnans yourWave
It could be that this task is a bit more complicated though - can't tell from your question. E.g. if it's one 2D wave or if you want to evaluate if the whole row is blank before deleting...
December 27, 2018 at 12:28 pm - Permalink
In reply to To remove NaNs from 1D… by sjr51
So some of the columns have missing values in some cells and I want to remove all such rows with a missing value. See the attached table.
December 27, 2018 at 12:41 pm - Permalink
OK so at the end the columns need to be the same length. The way I would approach this is to make a 2D wave with all the columns in. Then do
This will give a 1D wave that has NaNs for every row where there was a NaN in the matrix (because summing with a NaN gives a NaN). You can then use this to set all points each row where there is a NaN to NaN. You can test for NaN with Numtype == 2.
MatA[][] = (NumType(NaNLocWave[p]) == 2) ? NaN : MatA[p][q]
Next you can use SplitWave to break the matrix into columns. Run WaveTransform ZapNans on each 1D wave (do this in a loop) and then concatenate it back together.
December 27, 2018 at 01:30 pm - Permalink
The OP also asked this question of support@wavemetrics.com, sending in an example file.
I assumed he loaded the file as separate waves (all the same length) using Load Delimited Text into a table.
This code was presented as a solution:
December 31, 2018 at 09:58 am - Permalink