
Help topic for using assertions(?) to replace simple loops?
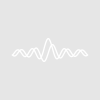
igorisfun
I think it's possible to replace a simple for loop like this:
w[0] = 1 for(i=0;i<numpnts(w);i+=1) w[i] = w[i-1] +1 endfor
using "?" and ":" but I can't seem to find the correct help topic. A simple example in the programming help file may exist but I can't seem to find it...
Or am I wrong and this is not possible? Is p indexing the correct way to this instead?
For constantly increasing (or decreasing) values, the p function would be a better choice, i.e.
However, depending on what you want to do you may want to look at wave scaling in case you just need "x-values":
Otherwise you were probably looking for:
April 29, 2019 at 02:49 am - Permalink
w[1,*] means from point 1 to the end (*).
w[3,10] would mean from point 3 to point 10, etc...
w[0,*] would be the same as writing just w[], which means all points.
April 29, 2019 at 03:12 am - Permalink
I probably should have mentioned that I tried p indexing using
but that doesn't evaluate over the whole range of wave w for some reason. If numpnts(w) = 3 then the above statements in the function evaluate to
w = [2,1,1] and w = [1,2,1], respectively (assuming w[0] = 1 statement from above).
I now see that the correct answer seems to be
w = p+1
for some reason it's not intuitive to me to think about p simply being the index number automatically. Thanks for the help!
April 29, 2019 at 03:20 am - Permalink
In reply to w[0]=1 w[1,*]=w[p-1]+1 w[1,… by olelytken
That's better than my w = p+1 answer, thanks!
April 29, 2019 at 03:22 am - Permalink
Your original loop will fail at i = 0 and is also very inefficient. For instructional purposes, here is a better way to write the loop:
But even then it is much more efficient to write this as a wave assignment statement so that the looping is done internally in Igor and not in Igor procedure code. That would also allow you to add the MultiThread keyword to the wave assignment statement if you wished to. The equivalent wave assignment statement is simply
w = p + 1
olelytken's response of
will give you the same end result, but it is less efficient because of the wave value lookup on the RHS of the assignment (the w[p-1] part).
April 29, 2019 at 08:02 am - Permalink