
Loading JSON File
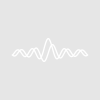
IgorRaven
I am trying to use the JSON XOP - my first time trying to use an XOP - but having problems with the instructions. The instructions are clear after loading the JSON file into a string.
Question: How do I load the JSON file to a JSON string to then use JSONXOP_GetValue to pass the data into waves?
Thank you!
Best,
Loading an arbitrary file is not exactly straightforward. Something like the following should do the trick:
This returns NaN on error or a valid jsonID in the sucess case.
December 7, 2020 at 06:40 am - Permalink
In reply to Loading an arbitrary file is… by thomas_braun
You might want to
Close refNum
before return? :)
December 7, 2020 at 09:13 am - Permalink
@tony: Thanks. Done.
December 7, 2020 at 11:50 am - Permalink
Great! Thanks, Thomas and Tony!
Best,
December 8, 2020 at 05:32 am - Permalink
JSON_Parse appears to be available on IGOR 8. I am still on 6. Perhaps is time to upgrade!
December 8, 2020 at 05:37 am - Permalink
Thoughts?
December 8, 2020 at 08:15 am - Permalink
Yes I would definitly upgrade. IP8 is so much nicer to use!
December 8, 2020 at 08:42 am - Permalink
Hi
I have a large json file (see attachment). I want to have the values put in igor waves. How can I use the function above in order to do that? Where do I load the file? How can I get this to run?
Cheers
Silvan
April 15, 2021 at 02:14 am - Permalink
@Silvan:
You need the JSON-XOP from https://docs.byte-physics.de/json-xop/. Install it.
And then you can use the below code as a starting point:
This is not a pure JSON file, it is in JSONLines format, https://jsonlines.org/, where each line is its own JSON document.
Whoever produced that file did also not create a standards-compliant file, as you can not use NaN when a number is expected. I've added some hacky fix for that.
April 15, 2021 at 04:04 am - Permalink
In reply to @Silvan: You need the JSON… by thomas_braun
@ Thomas_braun Genius!!!, thanks a lot. It works, I'm so happy
April 15, 2021 at 04:31 am - Permalink
Sorry Gents, I couldn't find the delete post...
December 31, 2023 at 09:53 pm - Permalink