
Using ButtonControl function inside another function
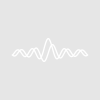
ankit7540
Hello all,
Is it possible to use a ButtonControl function, in a standalone way, inside another function. For example, below is a function linked to a button in a panel.
Function SimpleButtonFunc (s): ButtonControl String s SVAR pa = root:parameter_a SVAR pb = root:parameter_b print pa + pb end
We wonder if it can be used inside another function as,
Function AnotherFunction () // some calculations here SimpleButtonFunc() // does not work // or string tmp SimpleButtonFunc( tmp ) // does not work ! end
Above example does not work. Is there another way to have such functionality.
Thanks.
First, I cannot at all recommend that you call such a control function somewhere else in your code. This is not the way to do it. Instead, you would want to write a dedicated function which does what you want and then call this function from both your button control and somewhere else in your code, e.g.:
I also recommend to use the newer structure-based control functions:
But if you absolutely have to, you can call a simple (not structure-based) control function just fine by passing nothing for the string input (this obviously does not work if the control name is used in you button function):
SimpleButtonFunc("")
Your above example does not work simply because your input string 'tmp' is not properly initialized:
May 23, 2023 at 08:29 pm - Permalink
In reply to First, I cannot at all… by chozo
Thank you for the quick reply and suggestions.
May 23, 2023 at 09:15 pm - Permalink