
How fast is SOCKIT?
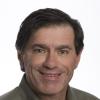
hegedus
Keithley provides an Eclipse based script builder(TSP - Test Script Builder) to program the unit and for some testing.
I have built the test script and executed in both Eclipse and Igor. The basic sweeps take about 10 sec, but it seems that in Igor getting the data out of the unit takes about 5 sec versus instantaneous in Eclipse.
In Eclipse the code to return the data is:
printbuffer(1, smua.nvbuffer.n,smua.nvbuffer1) ( which means print the buffer from point 1 to the last point (.n) of non-volatile buffer 1.)
which prints to Elcipse's version of the command window.
In Igor I do
SOCKITsendrecv socknum, "printbuffer(1, smua.nvbuffer.n,smua.nvbuffer1)", output
where output is a string that I parse into 4 waves. The total amount of data in the string is about 8500 characters.
Both environments are communicating via TCP/IP to the same IP address over the same network. I also did a bit of testing with Telnet and it also seems to be very fast. Any clues as to what I should look at? I am using Igor Pro 6.20 on a Windows box running XP.
Using sockitsendnrecv is a good first approach. However, when you use this option, you need to be aware of the /TIME flag.
Ok, so what you could do is use a small /TIME value, e.g. /TIME=0.1. If it's fast enough, then stop. However, there will probably be a faster way.
But the approach you take will depend on whether you know the maximum size of the message, or the structure of the message. How do you know when the message has finished? When you use telnet it doesn't matter, it just stops printing then waits around. If you used sockitsendmsg, with the output being printed to the history area I'm willing to bet the output would be presented just as fast.
I would use the following:
Start the socket using the /NOID flag in SOCKITopenconnection. This means that you are responsible for emptying the incoming buffer with the SOCKITpeek function.
e.g.
This will be a lot faster.
There will be other ways, but I would need to know more information regarding the incoming message.
BTW, you can use SOCKITstringtowave to directly convert the numbers in to a wave, but the data would have to be binary representations of floats/doubles, etc, not ascii. I'm willing to bet that that's even faster.
November 4, 2010 at 10:10 pm - Permalink