
3D data set to stereo lithography input
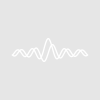
jtigor
I need to get data describing a 3D surface (from AFM data... it's just z values sampled over a uniform xy grid) to a stereo lithography machine. Has anyone tackled this before?
Apparently, an stl file is a standard input file for these devices. This file describes the surface via a mesh of triangles along with the normal to each triangle. The file then consists of an xyz triplet for each vertex of each triangle along with the normal. I haven't found anything (yet) in Igor to create the mesh. Image transform seems to be the likely suspect, but this hasn't panned out, unless I'm missing something.
I'm hopeful this post will generate some ideas.
Thanks,
Jeff
ImageInterpolate
operation with the Voronoi method. This requires an x,y,z triplet source wave, so you will have to convert your gridded data to a big triplet wave. Then use the /STW flag to save the Delauney trangulation data.July 16, 2012 at 06:59 am - Permalink
Indeed ImageTransform has a well-hidden feature called ccsubdivision. However, the Catmull-Clark subdivision is probably more appropriate for Pixar-type applications then for what you are doing. It is designed to create a higher density mesh so you can describe smoother surfaces.
If your original data represent samples on a regular rectangular grid then the actual triangulation is trivial, though non-unique as for each rectangle there are two choices of triangle representation. IP7 Gizmo does this calculation internally, i.e., for each rectangle create two triangles and compute the normals. The calculation of the normals is straightforward: pick a vertex of the triangle and compute the cross-product of the two vectors from the selected vertex to the other two triangle vertices.
Feel free to contact me directly through support@wavemetrics.com and I'll help you with that.
A.G.
WaveMetrics, Inc.
July 16, 2012 at 11:17 am - Permalink
AG -- I will follow up privately and post my results later.
Thanks,
Jeff
July 16, 2012 at 12:45 pm - Permalink
I thought it was obvious that Steve is correct and that you would get the triangulation for your data using ImageInterpolate Voronoi.
The down-side of this approach is that you are taking data that are essentially already triangulated and you run a very complex calculation, O(N^2), just to obtain a triangulation which you already know. Also, regardless of how you obtain the triangulation, you still need to generate the normals in a consistent manner. The latter, in my view, is the more interesting part of the problem.
AG
July 16, 2012 at 01:47 pm - Permalink
The output of ImageInterpolate for Voronoi with the /STW flag was a single column wave (W_TriangulationData). Are the components of each vertex on sequential rows in this wave?
At this point, it's all interesting for me.
Thanks,
Jeff
July 16, 2012 at 02:46 pm - Permalink
If you just want the "edges" you can use the /SV flag. The /STW flag results in a wave that is designed to be used internally; it is intentionally undocumented as it contains information that is used to reconstruct the internal structures that are built during the triangulation process; it does not have direct vertex information.
Below I have pasted complete code for generating the triangles and their normals for a 2D matrix wave. This is not necessarily a very efficient way of computing this but it should get the job done.
I hope this helps,
AG
July 17, 2012 at 10:32 am - Permalink
Sorry for taking so long to get back. Thank you for posting your code, it was very helpful. I do need the triangle mesh with normals, so being able to do the calculations is important. My surface is relatively simple in that it can be described by a 2D array; it is clear where the outside of the surface lies.
I have also been able to write files for the stereo lithography and have had models created. This has worked out very well.
Thanks, again,
Jeff
July 27, 2012 at 08:45 am - Permalink