
Export to EPS for LaTeX
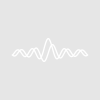
JMurray
// GUI for ExportEPS. Supports everything ExportEPS does, except it doesn't do sub-windows. Function ExportEPS_GUI() NewDataFolder/O root:Utilities NewDataFolder/O root:Utilities:ExportEPS // Load defaults Variable WidthCM = NumVarOrDefault("root:Utilities:ExportEPS:V_WidthCM",NaN) Variable HeightCM = NumVarOrDefault("root:Utilities:ExportEPS:V_HeightCM",NaN) String GraphName = StrVarOrDefault("root:Utilities:ExportEPS:S_GraphName","") String WdwList = "_TOP GRAPH or LAYOUT_;"+SortList(WinList("*",";","WIN:1"),";",16) + SortList(WinList("*",";","WIN:4"),";",16) Variable WhichGraph =WhichListItem(GraphName,WdwList) if (WhichGraph<0) WhichGraph = 0 endif Prompt WhichGraph,"Graph or Layout to Export",popup,WdwList Prompt WidthCM,"Exported width (cm) (NaN to preserve aspect ratio and set height)" Prompt HeightCM,"Exported height (cm) (NaN to preserve aspect ratio and set width)" WhichGraph+=1 DoPrompt "Export Graph to EPS",WhichGraph,WidthCM,HeightCM WhichGraph-=1 if (V_Flag) return 1 // user canceled endif // Set defaults GraphName = StringFromList(WhichGraph,WdwList) String/G root:Utilities:ExportEPS:S_GraphName = GraphName Variable/G root:Utilities:ExportEPS:V_WidthCM = WidthCM Variable/G root:Utilities:ExportEPS:V_HeightCM = HeightCM if (WhichGraph==0) // top graph GraphName = "" endif return ExportEPS(GraphName,WidthCM=WidthCM,HeightCM=HeightCM) End // Exports a graph or layout into an EPS file that LaTeX can use. // // Set GraphName to "" to select the top graph or layout. // Subwindows are also supported (but not sub-subwindows). // // If both WidthCM and/or HeightCM are specified and valid, they will be used to set // the width and height of the exported figure. (And if only one is given and valid, the // other will be calculated so as to preserve the aspect ratio.) // If neither is specified (and valid), the graph is used to set the size. // // If sub-windows are available but unspecified, the user will be prompted whether to // choose a sub-window or the host window -- unless DisableSubWinPrompt is true. Function ExportEPS(GraphName,[WidthCM,HeightCM,DisableSubWinPrompt]) String GraphName Variable WidthCM,HeightCM // in centimeters Variable DisableSubWinPrompt // option do disable prompt for sub-window (occurs if child windows present) if (ParamIsDefault(DisableSubWinPrompt)) DisableSubWinPrompt = 0 endif Variable SpecifiedWidth = ( !ParamIsDefault(WidthCM) && WidthCM>0 ) Variable SpecifiedHeight = ( !ParamIsDefault(HeightCM) && HeightCM>0 ) Variable SizeSpecified = SpecifiedWidth || SpecifiedHeight // Pick graph String GraphList = SortList(WinList("*",";","WIN:1"),";",16) String LayoutList = SortList(WinList("*",";","WIN:4"),";",16) String WdwList = GraphList+LayoutList if (strlen(GraphName)==0) // top graph or layout GraphName = WinName(0,5) if (strlen(GraphName)==0) return 2 // no graphs / layouts present endif else Variable PoundLoc = strsearch(GraphName,"#",0) String HostName = SelectString( PoundLoc >=0,GraphName,GraphName[0,PoundLoc-1]) Variable PromptForGraph = 0 if (WhichListItem(HostName,WdwList)<0) // graph doesn't exist! PromptForGraph = 1 elseif (PoundLoc >=0) // sub-window specified.... does it exist? String SubWindow = GraphName[PoundLoc+1,strlen(GraphName)-1] String ChildList = ChildWindowList(HostName) if (WhichListItem(SubWindow,ChildList)<0) PromptForGraph = 2 // DisableSubWinPrompt doesn't apply here, because user attempted to specify a sub-window that doesn't exist. endif endif if (PromptForGraph>0) // graph or subwindow doesn't exist String PopupList = SelectString(PromptForGraph==1,ChildList,WdwList) String PromptMsg = "Select "+SelectString(PromptForGraph==1,"subwindow of "+HostName,"graph or layout")+" to export" Variable WhichGraph = 0 Prompt WhichGraph,PromptMsg,popup, PopupList WhichGraph+=1 DoPrompt "Export EPS", WhichGraph WhichGraph-=1 if (V_Flag) return 1 // user canceled endif GraphName = SelectString(PromptForGraph==1,HostName+"#","")+StringFromList(WhichGraph,PopupList) if (strlen(GraphName)==0) // no graphs return 2 endif endif endif // If GraphName doesn't specify a sub-window and there are sub-windows available, ask // (but only if PromptForGraph!=2, in which case user didn't already select a subwindow, // and only if DisableSubWinPrompt is false) if (PromptForGraph!=2 && !DisableSubWinPrompt) PoundLoc = strsearch(GraphName,"#",0) if (PoundLoc<0) // no sub-window specified ChildList = SortList(ChildWindowList(HostName),";",16) if (strlen(ChildList)>0) // has subwindows available WhichGraph = 1 Prompt WhichGraph,"Select main window or sub-window to export",popup,"HOST WINDOW;"+ChildList DoPrompt "Export EPS", WhichGraph WhichGraph-=1 if (V_Flag) return 1 // user canceled endif GraphName += SelectString(WhichGraph==0,"#"+StringFromList(WhichGraph-1,ChildList),"") endif endif endif String FileName = GraphName+".eps" // Ask for file name / path Variable refNum Open/D/T=".eps"/M="Export graph to EPS figure" refNum as FileName String OutputFilePath = S_fileName if (strlen(OutputFilePath)==0) return 1 // user canceled endif // Set height or width according to aspect ratio, if only one quantity is specified if (SizeSpecified && !(SpecifiedWidth && SpecifiedHeight)) GetWindow $GraphName, gsize // works for host or sub-windows Variable AspectRatio = (V_Right - V_Left) / (V_bottom - V_Top) // width / height if (SpecifiedWidth) HeightCM = WidthCM / AspectRatio else WidthCM = HeightCM * AspectRatio endif endif // ---- Write to temporary file, then read contents ---- // (Code from Howard Rodstein 3/7/13) String pathToTemp = SpecialDirPath("Temporary", 0, 0, 0) String fullPath = pathToTemp + "TempEPSForLatexWork.eps" if (SizeSpecified) SavePICT/O/EF=1/E=-3/M/W=(0,0,WidthCM,HeightCM)/WIN=$GraphName as fullPath else SavePICT/O/EF=1/E=-3/WIN=$GraphName as fullPath endif // Get binary file contents Open/R refNum as fullPath FStatus refNum Variable numBytes = V_logEOF String EPScontents = PadString("", numBytes, 32) FBinRead refNum, EPScontents Close refNum DeleteFile /Z fullPath // -------------------------------------------------------------------------- // Remove stuff before "%%BoundingBox" Variable iLoc = strsearch(EPScontents,"%%BoundingBox",0) if (iLoc>=0) EPScontents = EPScontents[iLoc-1,strlen(EPScontents)-1] else DoAlert 0, "Export EPS error: Location of \"%%BoundingBox\" not found." return 3 endif // Write the file Open refNum as OutputFilePath FBinWrite refNum, EPScontents // write the EPS file Close refNum String sWdwType = SelectString (WinType(GraphName)==1,"Layout","Graph") print sWdwType+" \""+GraphName+"\" written to "+OutputFilePath return 0 // no error End
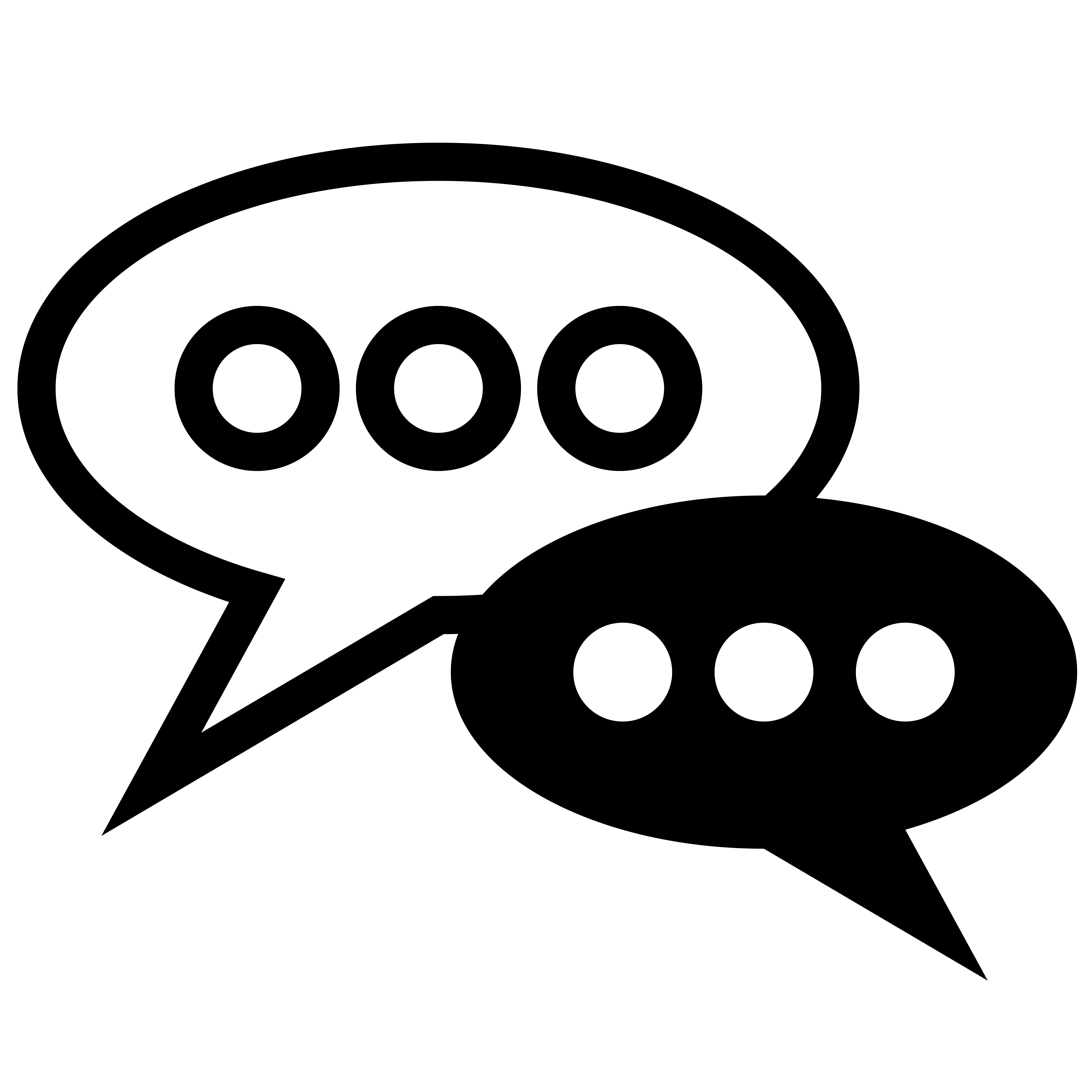
Forum
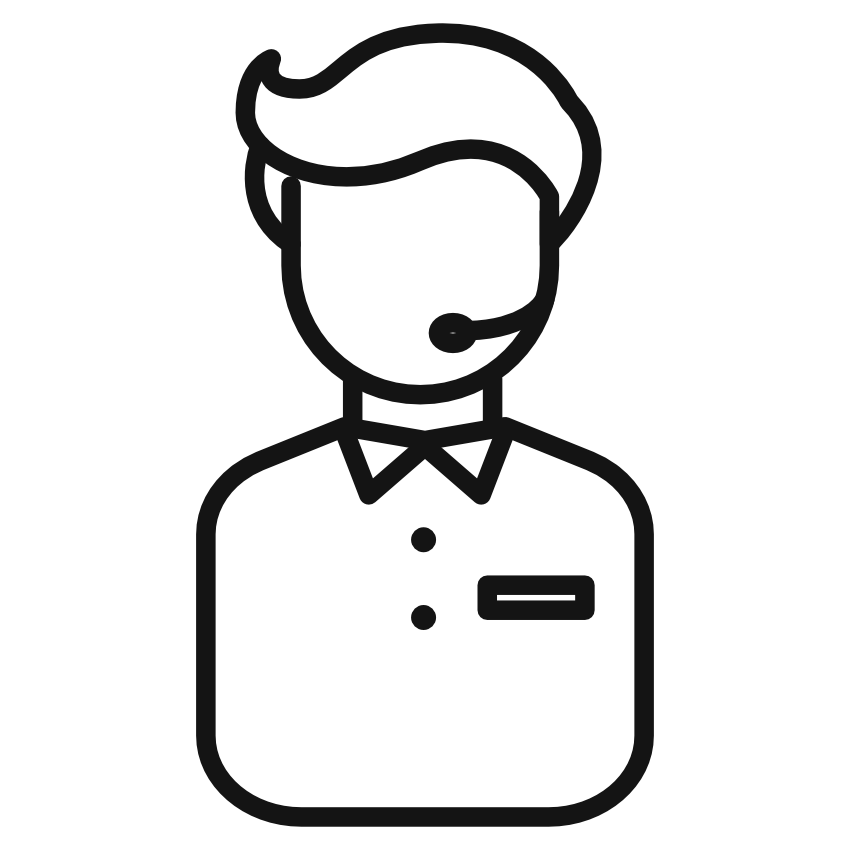
Support
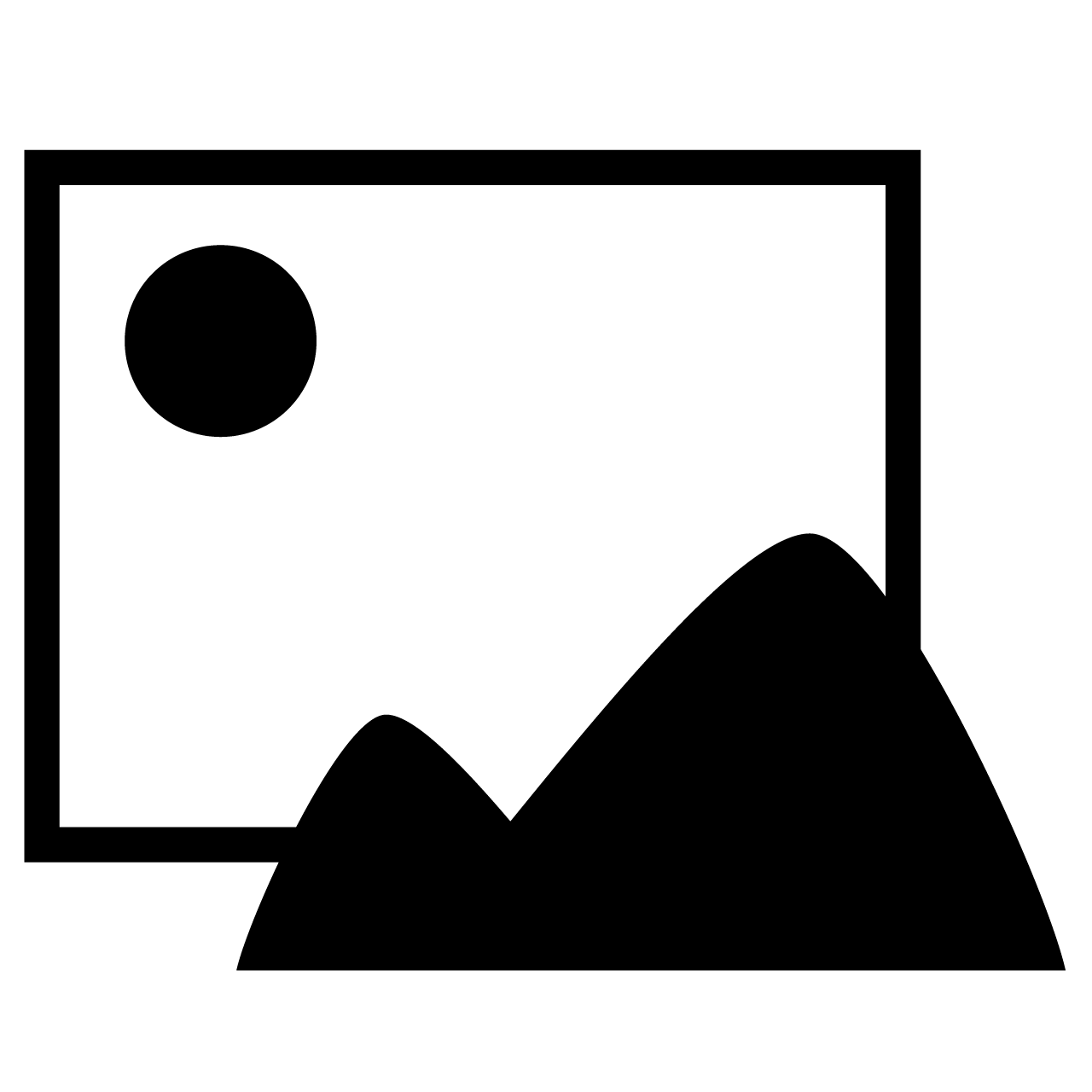
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
March 14, 2013 at 11:09 am - Permalink