
linear programming
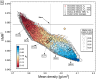
ChrLie
http://en.wikipedia.org/wiki/Linear_programming
There is code out there:
http://lpsolve.sourceforge.net/5.5/
and I wonder if there were already attempts to make use of that for Igor and if not what would it take to do it?
Cheers
C
The link you give is to code licensed under LGPL. Our understanding of this license (which is difficult to understand, and causes long threads on any developer forum where the topic comes up) is that we can use such code, but only with some difficulty. Another option, since you don't have the restrictions resulting from publishing a for-profit application, is to write an XOP that wraps up the API in that library.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
April 17, 2013 at 08:52 am - Permalink
I somehow feared that answer because currently writing an XOP is beyond my skills (I never looked into that and I don't have any experience in C). On the other hand, in my case the number of calls to lp_solve won't be excessive and a work-around for me could be to use Igor to write an input file which can then be processed simply from the command line of the operating system (on Mac in my case). Is there any way of executing a command from the system terminal via Igor and read out whatever lp_solve puts out? I guess that is in a very simplified manner what an XOP does, but would that be feasible?
April 17, 2013 at 01:05 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
April 17, 2013 at 02:23 pm - Permalink
* create a shell script that will run what you need when given two inputs, the input file and the output file
* put that shell script in your $PATH
* call that shell script from Igor using ExecuteScript
ExecuteScript "mylpsolver 'input file name' 'output file name'"
* wait for the return from the ExecuteScript command
* read the output file from Igor
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
April 17, 2013 at 03:03 pm - Permalink
It seems to use the MIT license.
April 17, 2013 at 11:53 pm - Permalink
I have not done this with AppleScript, but using Igor's ExecuteScriptText to run a Windows DOS BAT file. Igor was used to create the input file, call the BAT file (which ran a FORTRAN executable), wait for output file read access, read, analyze, and display the results. A bit kludgy, but should work with any executable whose input and output file formats are known.
April 18, 2013 at 07:08 am - Permalink
print DirList("/Users/jjw") --> prints directory listing
Suppose the shell level command in your $PATH is lpsolver with flags -r (recursive). You want to run lpsolver on file /Users/jjw/Documents/Data Files/April 23, 2012/first set/run002.dat. You want the output in the same directory as lp_run002.dat. Here is an example off the top of my head that should work.
MyLPSolver("/Users/jjw/Documents/Data Files/April 23, 2012/first set","run002.dat","r")
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
April 18, 2013 at 08:05 am - Permalink
John, would be great if linear programming and even a solver for non-linear constrained optimisation problems could be added in the future but I understand version 7 has priority now.
Thanks everyone!
C
April 18, 2013 at 08:15 am - Permalink
MMmmm... that looks interesting. The list of features includes "Easy to use graphical interface (GUI)". We would need, of course, to extract just the analysis code to use in Igor.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
April 18, 2013 at 09:17 am - Permalink
An input file looks like, e.g.
and S_value:
" Value of objective function: -0.06350000 Actual values of the variables: x0 0.1 x1 0.3 x2 0"
The bottleneck here is obviously the writing-to-disk part.
April 19, 2013 at 02:12 am - Permalink