
matrix math: finding random solution
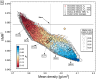
ChrLie
function foo (AA, cc) wave AA, cc Duplicate/FREE/O AA, frac frac = AA[p][q] *cc[p] MatrixOP/O bb_calc = (SumCols(frac))^t end
bb_calc and bb are identical. A constraint is that all elements in cc must be > 0.
E.g., if AA is:
aa[0][0]= {1,2,2,0,0,1,0,0,0,1}
aa[0][1]= {0,0,0,1,2,1,1,0,0,0}
aa[0][2]= {0,0,1,0,0,0,1,1,2,1}
and bb:
bb[0]= {2,1,1}
a possible solution would be:
cc[0]= {0.5,0.15,0.5,0.5,0.15,0.1,0.1,0.05,0.125,0.1}
I'd be grateful about ideas and suggestions!
Your function foo() looks like simple matrix multiplication of the transpose of AA so you can replace it with:
Now that you cast it into this form, it appears that you are looking for a solution of a system of the form:
MB=MA x CC
with MA (3x10 matrix) and CC (10,1).
If you were to use MatrixLLS on MA and MB you would get a minimum norm solution -- not one that restricts all elements of CC to be positive.
Clearly you could throw this into some optimize or root finding code but I'd be curious if there are other properties of the matrix AA that one could use. In particular, if AA is not random, I'd start by looking at its SVD.
AG
June 19, 2013 at 11:02 am - Permalink
Hi AG,
yes, of course! I ignored that there is "x" and "*" in MatrixOP! D'oh! I thought about MatrixLLS, but that doesn't solve the non-negative, non-zero issue.
This is the background to the question: I want to calculate chemical equilibrium using a Gibbs free energy minimisation technique, which however requires an initial guess for the abundance of the different species in the system. For simple systems this is easily done by hand, but of course an auto-guess is desirable. This guess must satisfy the mass balance constraint. AA is in this case a composition matrix with species in rows (e.g. CO, CO2, H2O, CH4, O2 ...) and number of atoms per species in columns (e.g. C, O, H), and bb is the total number of C, O, H in the system. cc represents the moles of each species.
Is there any way to tweak MatrixLLS, such that it produces different valid solutions?
June 20, 2013 at 01:36 am - Permalink
I think what you're asking for is what AG suggested: the Singular Value Decomposition. If you call MatrixSVD to operate on the transpose of AA, then the matrix V can be interpreted as a coordinate transformation of c so that the first three (assuming matrix AA has rank 3, as in your example) rows of V^t give exactly the linear combinations of elements of c needed to solve the system, and the remaining seven rows give linear combinations of elements of c that do not affect the solution. So you can pick a number, multiply one of the last 7 rows of V^t by that number and add that to the solution to produce a different valid solution.
June 20, 2013 at 06:20 am - Permalink
Thanks for pointing me in the right direction. My matrix math is a bit rusty!
June 21, 2013 at 01:17 am - Permalink
June 21, 2013 at 01:13 pm - Permalink