
Create your own terminal window
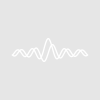
andyfaff
Normally one uses the Igor command line to call user defined functions and Igor+XOP Operations and functions. However, there is no way (AFAIK) of having a user defined operation from the command line. i.e. the user defined function must always be of the form "myfunc(arg1, arg2)" instead of "myop arg1 arg1".
Where this becomes interesting is when you are talking to something like a network server, or an acquisition device. Normally they have their own command set. One would wish to issue that command directly from a terminal instead of wrapping it up into a user function, i.e. why can't I write:
>drive ss2vg 10
instead of always having to write:
>sendmymessage("drive ss2vg 10")
The following code snippet implements a really simple terminal window. In this you type commands into the notebook window, on pressing return the commands are executed. If you wanted to make this act as a terminal for a device instead of the Execute/z/q line you could substitute the code that deals with your device, i.e. sendmymessage(S_Selection). All you have to do then is intercept the incoming messages and display them in the notebook. I leave that as an exercise for the reader (which is what people always say when they don't want to deal with that).
#pragma rtGlobals=1 // Use modern global access method. Menu "Macros" "Terminal Window/2", TerminalWIndow() End Function TerminalCmdHook(s) STRUCT WMWinHookStruct &s Variable statusCode = 0 String platform= UpperStr(igorinfo(2)) string cmdChar, temp if(stringmatch(platform,"MACINTOSH")) cmdChar = num2char(-91) else cmdChar = num2char(-107) endif strswitch(s.winname) case "Terminal#TerminalNB": switch(s.eventCode) case 11: // Keyboard switch (s.keycode) case 30: notebook $s.winname, findtext={cmdChar, 2^4+2^0} notebook $s.winname, selection={startOfParagraph, endOfParagraph} statuscode=1 break case 31: notebook $s.winname, findtext={cmdChar, 2^0} notebook $s.winname, selection={startOfParagraph, endOfParagraph} statuscode=1 break case 13: notebook $s.winname, selection={startOfParagraph, endOfParagraph} notebook $s.winname, textRGB=(0, 0, 65535), fstyle=1, fsize=8 getselection notebook, $s.winname, 2 execute/q/z S_Selection if(strlen(S_Selection) > 0 && (!stringmatch(S_Selection, "\r")) ) temp = "" notebook $s.winname, selection={startofparagraph, endofparagraph} if(!Stringmatch(S_Selection[0], cmdchar)) temp = cmdChar endif temp += S_Selection if(!Stringmatch(S_Selection[strlen(S_Selection)-1],"\r")) temp +="\r" endif notebook $s.winname, text = temp endif // if(strlen(S_Selection)>0) // notebookaction/w=$s.winname commands=S_Selection, frame=1,ignoreerrors=1, title=S_Selection // endif notebook $s.winname, selection={endofparagraph,startofnextparagraph} notebook $s.winname, textRGB=(0, 0, 0) statuscode=1 break endswitch endswitch break endswitch return statusCode // 0 if nothing done, else 1 End Function TerminalWindow() DoWindow/F Terminal if (V_flag != 0) return -1 endif NewPanel /W=(68, 50, 360, 500) /K=1 /N=Terminal SetWindow kwTopWin, hook(Hook1)=TerminalCmdHook NewNotebook /F=1 /N=TerminalNB/W=(10, 27, 290, 490) /HOST=# Notebook kwTopWin, defaultTab=20, statusWidth=0, autoSave=1, showRuler=0, rulerUnits=1 SetActiveSubwindow ## return 0 End
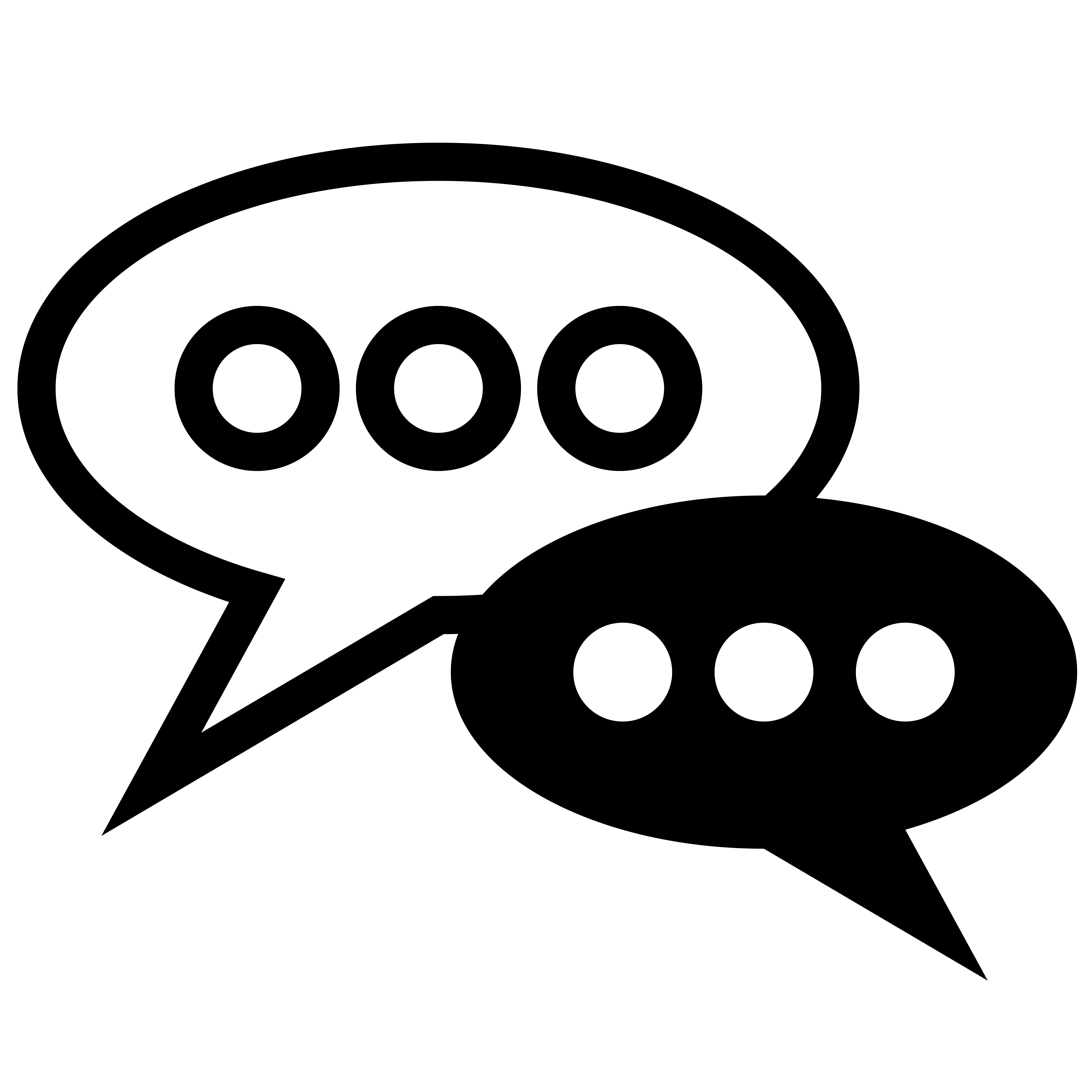
Forum
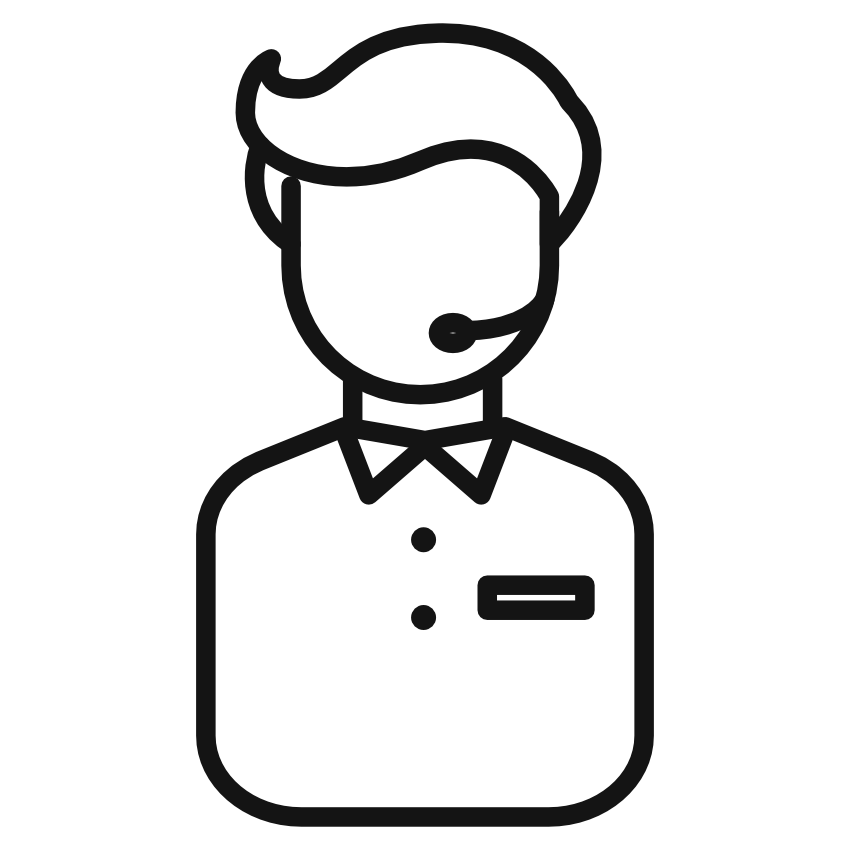
Support
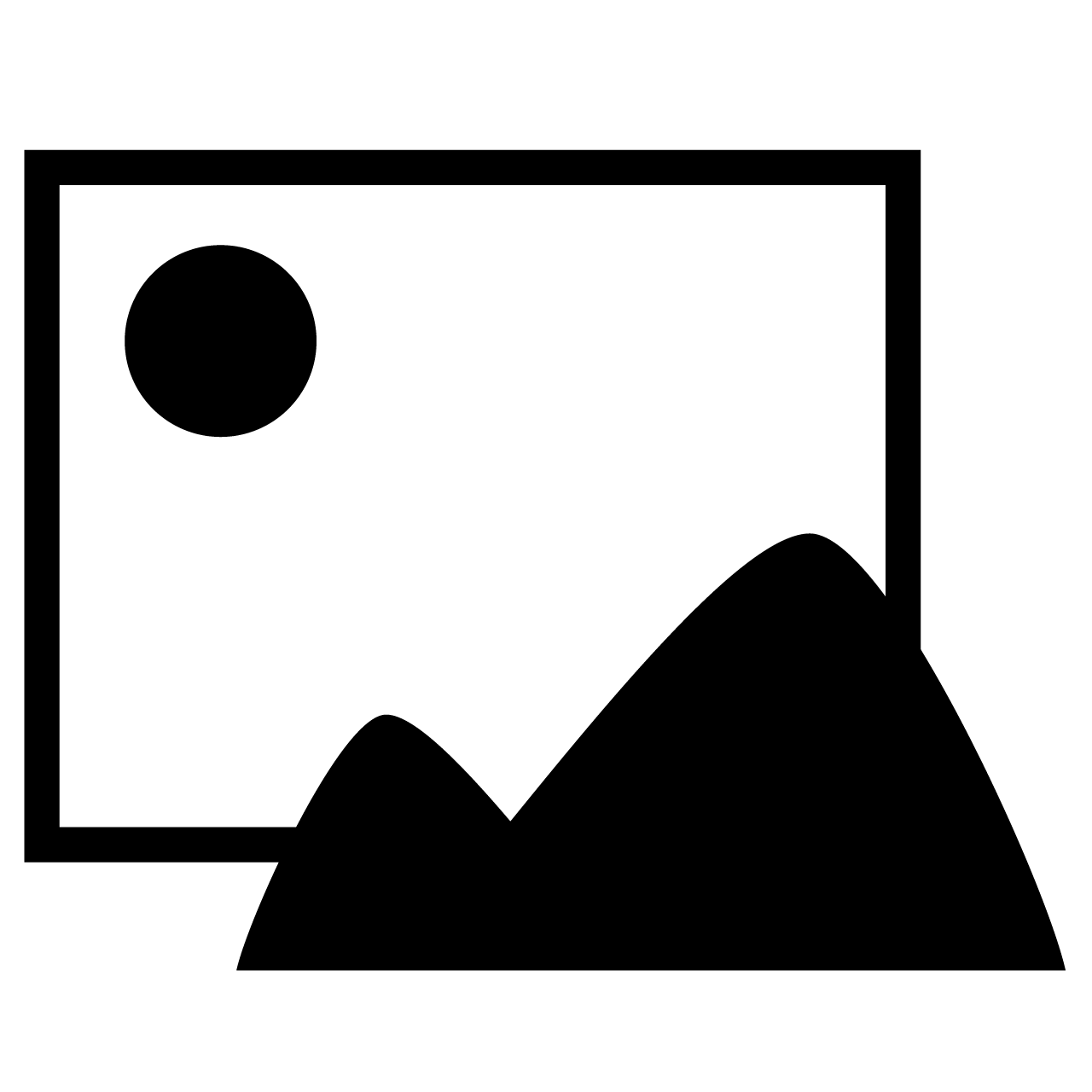
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
Wolfgang Harneit
March 10, 2009 at 12:28 pm - Permalink