
Combining waves
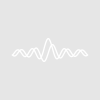
tkessler
Wave "A"
4
5
7
9
Wave "B"
1
3
4
8
Wave "C"
7
8
9
What I need to do is combine these waves, so the numbers from them are sequential, and not duplicated. So for instance, the result of the above three waves would be the following:
1
3
4
5
7
8
9
Is there some matrix/array math operation that can do this in Igor, or some built-in function? If not, then is there some programming technique that can accomplish this without resorting to a number of nested loops and conditionals? Ideally I'd like to be able to take an arbitrary number of such waves in a list string (ie, "WaveA;WaveB;WaveC;WaveD") as the input to a function, and have this function output a single wave combined in the method stated above.
It seems simple, but every time I wrap my head around it I get stuck on some impasse. Currently I can create a function that does this comparison for two waves, and then simply loop it in another function to compare its result to additional waves, but I'm hoping there is a more elegant solution (kind of like the "prime" notation in Matlab to transpose a matrix, instead of having to create a new matrix, then loop through the first and assign values to the second, etc.).
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
December 16, 2013 at 04:15 pm - Permalink
Yes, there is a way in two lines of code. Assuming wave0 contains the concatenated and sorted data, then:
Hope this helps,
Kurt
December 16, 2013 at 11:21 pm - Permalink
December 17, 2013 at 10:55 am - Permalink
what is [p] here?
--Now let's all stay positive, and do some science.
March 18, 2014 at 05:49 pm - Permalink
In a wave assignment statement p takes on the value of the row number. In the statement example given, there is an implicit loop such that it takes on each row number in turn from the first row (row 0) to the penultimate row (row N-2, where N is the total number of rows in the wave - note the last row in the wave is row N-1).
Type the following in the command line for more details:
March 18, 2014 at 11:56 pm - Permalink
March 19, 2014 at 09:29 am - Permalink