
Problem to check whether all elements of a text wave are equal
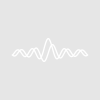
PeterR
I have tried to construct a procedure which returns whether all strings in a 1D-textwave are equal.
This is what I did:
// Handy function to check whether two string variables are equal Function AreEqual_str(str1,str2) String str1, str2 if(cmpstr(str1,str2)==0) return 1 else return 0 endif End // Handy function to check whether all elements of a text wave are equal // returns "0" if not all strings in the text wave are equal Function TextWaveEqual(Textwave) Wave /T Textwave Variable NOP = numpnts(TextWave) Variable counter, result String Str1,Str2 for(counter=0;(counter+1)<NOP;counter+=1) Str1 = TextWave [counter] Str2 = TextWave [counter+1] if(AreEqual_str(Str1,Str2)==0) Break return 0 endif endfor End
The problem now is, that the function always only returns NaN, and I can't figure out why...
Any help would be highly appreciated :)
Regards,
Peter
return 1
just before the end of the function.In case it matters,
CmpStr
does a case insensitive comparison by default.August 4, 2014 at 05:40 am - Permalink
The function returns Nan in any case, whether all elements are equal or not...
I tried return 1, but it overwrites the return 0, so that the function always returns 1...
Maybe I could return 1 by default, and only return 0, when the loop breaks...
August 4, 2014 at 05:44 am - Permalink
Thanks for the input, aclight!
August 4, 2014 at 05:50 am - Permalink
Unless I am missing something, your function will return 1 any time two places in the text wave are the same. Perhaps this is what you mean ...
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
August 4, 2014 at 11:35 am - Permalink
Someone at Wavemetrics (AG) may offer a MatrixOp to compete in speed :-)
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
August 4, 2014 at 11:50 am - Permalink
August 4, 2014 at 02:33 pm - Permalink
Since you asked:
MatrixOP is strictly numeric which suggests that you might do better converting the text wave entries into unique numerical representation and then using MatrixOP. StringCRC does not appear to be very efficient.
A.G.
WaveMetrics, Inc.
August 4, 2014 at 03:05 pm - Permalink
A.
August 4, 2014 at 05:27 pm - Permalink
Oh. I see now why.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
August 4, 2014 at 05:52 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 7, 2014 at 01:55 pm - Permalink
August 12, 2014 at 09:38 am - Permalink