
Calculating elapsed time from existing text
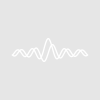
Dear all
I have two text waves containing date/time in the following format:
YYYY-MM-DD HH:MM:SS.XXXXXX (a real life example: 2022-11-18 09:06:55.370769)
I would like to calculate the time between the two entries. In a simple approach I can ignore the YYYY-MM-DD, but there are times when measurements starts one day and end the other, so I need to take this into account.
Does anyone have a function that calculates the amount of time between two entries of the above format (preferably in seconds)? I guess it is possible to write myself, but with leap years etc. etc. it would be great if there is something built-in (I tried to look for it but could not find it) or written by someone else...
Best,
Johan S
I solved it myself, not so beautiful but anyway - here is the solution...
Edit: The string "s_Current_DF" must be set by you in your code in case you want to use this - this is just an example of how I solved the problem with text-waves...
January 11, 2023 at 10:31 am - Permalink
The question that comes immediately to my mind is- why are the times in text waves? Seems like that times are in a reasonable format, and it should be possible to load the time/date data into a numeric wave, which would make the computation trivial.
January 11, 2023 at 11:13 am - Permalink
In reply to The question that comes… by johnweeks
Dear John
I load a large dataset from an HDF5 file and these waves "end up" as text - probably due to how they are saved by another program. So I have to adjust to this... The code-snippet above solves this.
January 11, 2023 at 11:20 pm - Permalink
You could alternatively use sscanf:
January 12, 2023 at 03:24 am - Permalink
In reply to You could alternatively use… by tony
Thank you, this was much more elegant...
January 12, 2023 at 04:35 am - Permalink
I don't have any real experience with HDF5, but our documentation doesn't mention date/time data. Surely there is a better way to load that data? All you HDF5 folks out there?
January 12, 2023 at 09:56 am - Permalink