
Can't get rid of "ambiguous wave point number" error
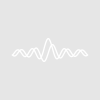
I've seen a couple of examples on the forum, but I couldn't translate their solutions to my own problem. I've inherited the following code from a former labmate. The first function makes a wave. The second function sends that wave to an instrument.
// : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : // Convert command string to ascii-code buffer // : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : Function/WAVE ConvertStr2Buff(commandString) String commandString Make/O/N=(strlen(commandString))/B buffWav Variable i for(i=0;i<=strlen(commandString);i+=1) buffWav[i-1]=char2num(commandString[i-1]) endfor return buffWav End // : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : // Send string as GPIB command // : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : : Function SendGPIBString(address,commandString) Variable address String commandString Variable L=strlen(commandString) Make/O/N=(L)/B bufferWave=ConvertStr2Buff(commandString) NI4882 Send={0,address,bufferWave,L,0x02} End
However, I get an "ambiguous wave point number" error for the third-to-last line:
Make/O/N=(L)/B bufferWave = ConvertStr2Buff(commandString)
I'm confused by this, because the error is suggesting that Igor is not expecting a wave, but the left hand of the equality is a Make function.
It might be important to note that the first function, when I first opened the code, did not contain the "/WAVE" required for it to properly return the wave buffWav. I assume that older versions of Igor did not have the "/WAVE" requirement, but I'm not sure.
Thank you for any help!,
Roy
HI,
Played around a bit and this compiled.
July 29, 2021 at 07:58 pm - Permalink
In reply to HI, Played around a bit and… by hegedus
Hi Andy,
Thank you for the help! I'll go into the lab tomorrow and see if it works. Can I ask what [p] does and when I should use it? I've been using Igor for using and have never seen it.
Roy
July 29, 2021 at 11:08 pm - Permalink
[p] will use the destination wave's (the one on the left side) point counter for accessing the source data range. In other words by using [p] each destination point gets assigned the respective source data. A simple example would be wave1_out = wave2_in[p], which successively assigns each point of wave2_in to a point p of wave1_out (this only works without errors if both waves have the same number of points, of course). But you can also omit it in this specific case. In above example, I guess the compiler has a bit of a difficulty to decide what type the output of ConvertStr2Buff() is without the [p] and how the assignment for each point of bufferWave should be handled. You can read more about all this stuff here:
DisplayHelpTopic "Waveform Arithmetic and Assignment"
EDIT: On closer inspection it seems to me that the problematic line makes no sense anyway. Since buffWav is already created inside ConvertStr2Buff() in the right format, it is useless to create yet another buffereWave with the same contents. Also, ConvertStr2Buff() is called for every point, which does the whole process over and over again. Just having this should be fine:
Wave bufferWave=ConvertStr2Buff(commandString)
July 29, 2021 at 11:18 pm - Permalink
chozo is right that you shouldn't be using Make here. I would rewrite your function like this:
Note that if you happen to be using Igor Pro 9.00B08 or later, you can use the new built-in StringToUnsignedByteWave function instead of your ConvertStr2Buff function(*). Here's how your SendGPIBString function would look in that case:
(*): Your ConvertStr2Buff function looks wrong to me on this line:
buffWav[i-1]=char2num(commandString[i-1])
Since i starts at 0, the first iteration of your loop will try to read from commandString[-1] and write to buffWave[-1], both of which are invalid. I would rewrite it as:
I would also add the /FREE flag to the make command there but depending on what you're doing in your calling function that might not be desirable.
July 30, 2021 at 08:28 am - Permalink