
Exception catching in loop -- Index Out of Range
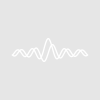
aperson
In the database I might have a collection of waves something like: Basename0, Basename1, Basename3. So, when Basename2 is used, the loop fails.
here's the code:
function AreaU()
variable numWave=40
variable index = 0
variable timeBegin = 1
variable timeEnd = 10
Make/O/N = (numWave) AreaWave
do
AreaWave[index] = area ($("Basename"+num2istr(index)) , timeBegin, timeEnd)
index += 1
while (index <= numWave)
end
Thanks!
waveexists
function.--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
March 30, 2018 at 04:00 pm - Permalink
But now you have an infinite loop. How about a slightly different approach:
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
March 30, 2018 at 05:03 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
March 30, 2018 at 05:08 pm - Permalink
April 2, 2018 at 09:03 am - Permalink