
Help on coding time evolution of spectrum
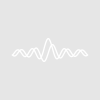
TRK
I am writing code to display a series of energy spectrum that changes with time. Basically the intensity of the curve decreases at a certain energy value while at other energy value the intensity decreases with time. Since I know how my spectrum are from the data I have, I wrote an equation to fit the spectrum and fixed all the parameters except the intensities at two different energy points. The fitting curve is good. Now I am trying to include a time variable in the equation to get a series of spectrum evolving with time. However keeping time variable in the coding displays 'expected assignment error'. It does not let me put the time in the equation. Since I am learning step by step how to code, any help would be great.
Below is my coding and also I have attached the igor file for clarity.
#pragma rtGlobals=3 // Use modern global access method and strict wave access. Function MySpectrum(ww) WAVE ww //Variable xx Variable w1=0, w2=0, w3=0, w4=0, w5=0, w6=0, w7=0, w8=0, i, t=0, qq // local variable make/O /N=46 xx=1.64+0.04*p //x axis energy values; start:step:end = 1.64:0.04:3.24 for fit // Body code for(i=0;i<40;i+=1) t=1 //time step w1 = exp(0.5*(0.055/0.27)^2-(xx-1.905)/0.27) w2 = erfc(0.707*(0.055/0.27-(xx-1.905)/0.055)) w3 = ww[0]*exp(-3.70*i*t) //ww[0]=intensity of first peak which decreases with time w4 = 0.5*w3/0.27 w5 = exp(0.5*(0.1/0.105)^2-(xx-2.19)/0.105) w6 = erfc(0.707*(0.1/0.105-(xx-2.19)/0.1)) w7 = ww[1]*exp(1-3.70*i*t) // ww[0]/ww[1] = 1.5, where ww[1]=intensity that increases slowly with time w8 = 0.5*w7/0.105 qq(i*t) = (w4*w1)*w2+(w8*w5)*w6 endfor return qq Display qq(i*t)
Perhaps you meant it to be a wave. Then you need to create it using Make, not Variable.
Another issue which I see in Igor7 but not in Igor 6.37 is that xx is a wave but you are treating it like variable. For example:
You need to specify which point of xx you want to use in the assignment to the variable. Perhaps xx[i] is what you want.
You have an extraneous statement at the end, after the return statement.
October 25, 2017 at 05:25 pm - Permalink
TRK
Thanks for the suggestion. The return function qq[i*t] is a wave which changes with time. I used (i*t) to display the wave for a particular time. As suggested, I used make command to create an array of 41 points for qq.
xx is a wave which I created using make command and I want every value of xx to subtract a fixed assigned number i.e, 1.905 and 2.19 so I am not sure if I need to write xx[i] but I tried with both.
And I removed the display function at the end but still the problem exists in code compiling
October 25, 2017 at 06:44 pm - Permalink
TRK
Hi
This is a follow up to my previous post. I was able to program how spectrum evolves with time though it did not match perfectly to my data. Hence I want to use the actual population which I have saved in data folder named as peak1 and peak2. Taking help from the igor help, I wrote a code to call the waves peak1 and peak2 in the main function but there occurs compiling error saying unkonwn/appropriate name or symbol. How do we call a wave from the data folder to a user defined function ? I would appreciate any help/comments to resolve this. I have attached the igor file.
Here is the code
November 16, 2017 at 01:52 pm - Permalink
displayhelptopic "User-Defined Functions"
Try to write a smaller and simpler function. Once you can get that to work, build up to the calculation you're trying to achieve.
November 16, 2017 at 02:46 pm - Permalink
TRK
Thanks Tony, I have been looking at data folder references subtopic of of programming help file and had tried my best before posting the problem. Any example on how the wave in a data folder can be applied to a user defined function would be appreciated.
November 16, 2017 at 03:14 pm - Permalink
You can declare a wave in a function like this:
wave wave1=w1
wave1 will be a wave reference in your function for the wave w1 in the current data folder.
displayhelptopic "wave"
you can also pass wave references, variables, etc to the function, but you cannot return the wave because it already exists outside of the function.
execute
displayhelptopic "User-Defined Functions"
and look at the third example function.November 16, 2017 at 04:10 pm - Permalink
TRK
Thank you so much Tony ! Works perfectly and looks so simple now. I am still wondering if the data folder references could be used to call a wave in the user defined function.
November 16, 2017 at 05:09 pm - Permalink
By "call" do you mean to work on a wave in your function? If so, then what Tony showed you was most of the story but you may need to read up on data folders in IgorPro. The basic idea is that if you are trying to reference a wave that is not in the current data folder, you have to specify a full path when declaring the wave reference.
So if your function is working in
root:
but the wave you want to work on is in e.g.root:folder1
then you would want to make that declaration as follows:WAVE wave1 = root:folder1:w1
Once you have that reference all set up, you can then code with wave1 without worrying about giving the full path, because IgorPro now knows where to find that specific wave each time you want to use it for something.
November 25, 2017 at 07:44 am - Permalink
TRK
Thanks Yanick! This is exactly what I am doing. Since the subtopic 'data folder references' under topic 'programming' in help menu also talks about calling wave, I was just curious how to apply this in a user defined function.
November 25, 2017 at 03:41 pm - Permalink