
How to simulate a loaded dice in Igor Pro?
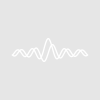
Hi. I am looking for a way to generate random states with non-equal probabilities. A simple example to illustrate this would be a loaded dice, where outcome of 1 is 2p/3, the probability of obtaining 2, 3, 4 or 5 is p each, and the probability of obtaining 6 is 3p/2. My approach so far has been to pick random numbers from a wave that would have 6x100 points with the first 66 points equal 1, next 100 equal 2, and so on, with the last 137 points equal to 6. This code shows how a loaded coin would work:
Function return_prob(Probability) Variable Probability Make/O/N=100/FREE ProbW=1*(x<Probability*100)+0*(x>=Probability*100) Return ProbW[50+enoise(50)] End
However this is computationally very expensive and takes too long to be practical, as I need to generate large sets of data. Also has low resolution, as sometimes the probabilities are at the 7th digit, and making a wave with Nx10^7 only makes things worse.
Is there a function in Igor Pro similar to the one in R that can do this faster/in one shot? This is how that code would look like:
myprobs <- c(2/3 * p, p, p, p, p, 3/2*p) x <- sample(1:6, size = 100, replace = TRUE, prob = myprobs)
how about
March 10, 2021 at 12:39 pm - Permalink
A roll of the die is floor(enoise(3) + 4), so for p(6) > 1/6, try this:
March 10, 2021 at 11:10 pm - Permalink
for p(6) = 1/4, as per your example:
March 10, 2021 at 11:48 pm - Permalink
In your example, IVP, the probabilities of the loaded dice do not add up to 100%. Is that intentional? If the probability of rolling a 6 is 3/2*1/6 I would expect the probability of rolling a 1 to be 1/2*1/6. This is assuming that the only effect of the loaded dice is to convert a given fraction of 1s into 6s.
To make that clear, I would write the code like this:
March 11, 2021 at 03:04 am - Permalink
or do you need something more general where you have a list of events, each with different probabilities?
event 1 0.10
event 2 0.20
event 3 0.20
event 4 0.40
event 5 0.90
etc...
March 11, 2021 at 03:15 am - Permalink
This is how I would handle the more generalized case. I haven't tested the histogram. You'll want to make sure to do that before you use the code.
March 11, 2021 at 04:02 am - Permalink
In reply to In your example, IVP, the… by olelytken
Hi Olelytken,
It was unintentional... I picked that example from the internet... Probabilities should add up to 1.
Thanks
March 11, 2021 at 06:16 am - Permalink