
Moving several data folders
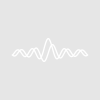
tooprock
I am trying to move (or regroup) several data folder into pre-defined data folder. My goal is regrouping them to use another function to concetenate them into single waves. Here is an example which shows the data folder structure in my case:
-> root
+ DataFolder1_0000
+ DataFolder1_0001
+ DataFolder1_0002
+ DataFolder1_0003
+ DataFolder2_0000
+ DataFolder2_0001
I would like to create two new data folders (DataFoldersGroup1 & DataFoldersGroup2) and move the data folders ending with "_0000" into DataFoldersGroup1 and all other data folders into DataFoldersGroup2. Does anybody have an idea?
Thanks,
Emre
February 27, 2013 at 10:53 am - Permalink
Thank you so much. It is now clear to me. I will test it and post the results.
Cheers,
Emre
February 28, 2013 at 04:56 am - Permalink
One part is working great but have trouble with second part.
Best,
Emre
March 1, 2013 at 04:33 pm - Permalink
I was wondering why do we need to do all in reverse order? When I move data folders they are in reverse order and this causes some problems for the next step. I tried to change it. However it doesn't work entirely. What am I doing wrong? First function works correctly but the second function moves only the first data folder and skips the second one (when I have two data folders ending with _0000 and two ending with 0001 and 0002).
Best,
Emre
March 1, 2013 at 04:36 pm - Permalink
Because otherwise index and numDataFolders will be wrong.
You can probably fix this by decrementing index and numDataFolders after calling MoveDataFolder.
March 1, 2013 at 05:04 pm - Permalink
March 2, 2013 at 10:10 am - Permalink
Assuming you are moving data folders in forward order, when you move a data folder out of the parent data folder, numDataFolders is 1 fewer than when you started so you must adjust its value by subtracting 1 from it.
Similarly index is affected by the fact that you moved the data folder out of the parent and you must adjust its value by subtracting 1 from it.
March 2, 2013 at 10:26 am - Permalink
March 2, 2013 at 12:32 pm - Permalink