
Observations using drawing tools
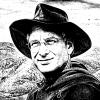
I am setting up a programming to use drawing tools on an image window. I noticed some behaviors where I have questions.
* The only way to determine whether drawing tools are already active programmatically is by parsing the WinRecreation. Could we have a method with GetWindow active where v_value = 2 designates the graph as active with the drawing tools active?
* I have a string winrstr = WinRecreation(...) that has ShowTools in it. To find the string "ShowTools", I must use WhichListItem("ShowTools",winrstr,"\r\t"). The function WhichListItem("ShowTools",winrstr,"\r\") will return -1 (string not found). Interestingly, StringFromList(...) does not care whether "\r" or "\r\t" is used as the separator. Perhaps this difference should be included in the help examples for WinRecreation.
* When I set the drawing tools via ShowTools, I cannot set the initial color or line style for the objects that are being actively drawn. The active drawings are always black, solid lines with thickness = 1. Can I set this initial active drawing line style programmatically? If so, how? If not, perhaps SetDrawEnvironment should allow a way to do this.
On your second question, you have to realize that any text that isn't in the separator has to be in the list item. This works:
Note that the tab character is at the beginning of the item string, not in the separator string. And the recreation command includes the /A flag.
May 12, 2023 at 11:58 am - Permalink
Third question:
When I then manually draw a line using the tool selected by the code, I get a red line.
That's how it works: SetDrawEnv by itself like that preps for the next thing that happens, including using a specific drawing tool.
May 12, 2023 at 12:03 pm - Permalink
EDIT -- Strike this first one -- I had a mistake in parsing StringFromList. The command below does report a tab as the first character to the second item.
1) So, by reference, StringfromList should not return "ShowTools/A" from WinRecreation, it should return "\tShowTools"? My note is only to point out the distinction where WhichListItem requires the tab (either as part of the delimiter or within the string item) while StringFromList does not return the tab when using only "\r" as the delimiter.
3) I am doing this.
The result still draws during the drag operation using a black line at thickness = 1. Only after I release the drag do I get a white line at thickness = 2.
May 12, 2023 at 01:58 pm - Permalink
In reply to 1) So, by reference,… by jjweimer
You may not have noticed, then, that *any* dragged/selected/resizing drawing object is drawn in a simplified way (which we refer to as "outline mode"). Once the dragging/selection/resizing stops, then the "presentation" appearance is used to draw the object(s).
May 13, 2023 at 03:07 pm - Permalink
Thanks for the clarification.
I am including the ability to use drawing tools in the image tools package. I initially expose the tools with the arrow selected to allow a user to choose the drawing tool. The outline drawing with a black line gets lost on images that are grayscale with mostly black backgrounds. It would help to have a way to programmatically preset the color of line in outline mode, even if only between black and white (e.g. SetDrawEnv outline=0/1).
Alternatively, I can imagine eventually wanting to have the option to be able to change the shape of an existing drawing, for example using the context menu and/or the shape dialog box itself. I could then pre-populate an image with a fixed type of drawing shape (e.g. a square in the image center) that has a selectable line color and simply presume that a user would change its shape as needed.
May 14, 2023 at 07:16 am - Permalink