
Update a graph using a slider
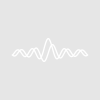
igornoob12
I am trying to update a graph by choosing the initial and final indices of the data wave using sliders.
I have something like this:
Window graph() : Graph Variable start,stop PauseUpdate; Silent 1 // building window... start = 0 stop = DimSize(pos,0) display vel[start,stop] vs pos[start,stop] ControlBar 42 Slider slider0 vert=0,proc=SliderProc,limits={0,DimSize(pos,0),0} EndMacro Function SliderProc(ctrlName,sliderValue,event) : SliderControl String ctrlName Variable sliderValue Variable event // bit field: bit 0: value set, 1: mouse down, 2: mouse up, 3: mouse moved if(event %& 0x1) // bit 0, value set endif return 0 End
How do I proceed to make the graph update based on the values of start, stop?
Thanks
duplicate /O /R(Start,Stop) datawave root:displaywave
within your if clause.(Usually I have a fixed frame size and only use an assignment, but with dynamic ranges overwriting might be better than redefining)
Other opinions?
HJ
July 9, 2015 at 03:32 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 9, 2015 at 04:11 pm - Permalink
If not, you can look at that code to see how a slider can change a graph's axis range.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
July 9, 2015 at 04:49 pm - Permalink
Unless I misunderstood your solution I don't want to change the axis range but the number of wave data points that are displayed.
July 10, 2015 at 10:56 am - Permalink
Thank you for the suggestion.
Here is what I got:
However, both sliders become coupled for some reason. i.e changing one also changes the other at the same time.
EDIT:
Nevermind, I figured it out. CmpStr returns "False" if the strings are equal.
July 10, 2015 at 11:53 am - Permalink
Technically, CmpStr returns 0 (not "false") if the strings are equal.
It also returns -1 if the first string is lexically "less than" the second, and it returns +1 if greater.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
July 10, 2015 at 04:04 pm - Permalink
StringMatch
has the expected behavior in the above code. The matching criteria is a bit loose, as case sensitivity and such is not included. But by using wildcards one can also write case switches for groups of controls.July 13, 2015 at 12:20 am - Permalink