
why do wave assignments for zero-length wave sometimes give indexing error?
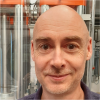
I was surprised to discover that functions in a wave assignment for a zero-length wave are executed with a NaN or null string. Is there a reason this should be so?
From the command line, a wave assignment for a zero-length wave always generates an indexing error.
Within a function, an assignment like wave0=1
doesn't generate an error, but wave0=abs(wave0)
generates an 'index out of range' error. Seems to me that it's Igor's choice to look for an out of range value when he doesn't have to? If I think of the wave assignment as an implicit loop, it's like writing for(i=0;i<0;i+=1)
and finding that I enter the loop with i=NaN and generate a run-time error.
If I write wave0=f(wave0)
for zero-length numeric waves in a function I get an out-of-range error, but for text waves a null string is passed to the string function f
without an out-of-range error, so if my function checks for null strings I can get away with it. That seems inconsistent.
This implies that if my code has a possibility of redimensioning a wave I must check for non-zero wave length before an assignment statement, or for a text wave consider the possibility of a string function executing with a null string.
Thoughts?
[I posted this to the old IgorExchange site, but both my post and Thomas Braun's followup to ask which Igor version I'm using were lost in the transition. The old site resurfaced and I noticed my post still sitting there.]
Igor 8, mac
Well I'm all on your side!
I've poured your post into code. This uses my unit-testing-framework.
I would expect that this generates no errors.
To replicate:
- Install UTF
- Put code into main procedure window
- Save Experiment and execute `RunTest("Procedure")`
August 30, 2018 at 01:14 pm - Permalink