
Loop causing inexlicably "Index out of range for wave CU_AA"-error
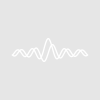
PeterR
I have encountered a strange problem that I can not figure out:
The following code should be used to compare a List of amino acids with a user-defined input sequence. The found values shall be written to a wave CU_AA, which should be enlarged by 1 whenever a new matching amino acid is found.
for(c=0;c<(numpnts(AA_OLC));c+=1) TransientActiveAA = AA_OLC [c] // Compare Transient active AA and active AA: if(cmpstr(TransientActiveAA,ActiveAA)==0) // If equal Wave /T CU_AA if(waveexists(CU_AA)==0) Make /O /T /N=1 CU_AA CU_AA [0] = Codons [c] else Redimension /N=(numpnts(CU_AA)+1) CU_AA Variable test = numpnts(CU_AA) CU_AA [test] = TheValue endif endif endfor
On the second run of the loop, the program stops - error code: "Index out of range for wave CU_AA"
This I cannot understand, since the index normally changes from 1 (
Make command
) to 2 (Redimension command
).Where is the bug?
Thanks in advance for any help!
Regards,
Peter
It should be:
A neater way to do it is:
October 3, 2014 at 06:52 pm - Permalink
October 4, 2014 at 01:50 am - Permalink
Redimension
in the code, I would add some quadratic growth strategy to the code.E.g:
October 4, 2014 at 09:27 am - Permalink