
Extracting contents of table's target cell?
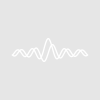
As part of a programming project using Igor Pro v.8, I want to make sure that numeric data my user enters into a table (itself a subwindow within a panel), is properly bounded. I've created hook functions for the parent window and for the table subwindow and plan to detect when the user moves away from the target cell (ex. Enter or Return, Arrow Keys, etc). At that time, I want to read the content of the target cell and limit the entered value to within my [min, max] range.
In this circumstance, how can my code determine the contents of the target cell? In some circumstances it seems sufficient to use the value of the corresponding point in the wave represented by the column in the table, but in other circumstances, that scheme doesn't seem to work. For example: move to a cell already containing a value; enter a new numeric value; press Enter. In this case, the point in the wave does not yet (in the hook function), contain the new numeric value -- instead it contains the previous point.
I would've thought that GetSelection table, ... would be approp. function to read the data directly from the table itself, rather than the 'backing wave', but I haven't yet found a way to get the value of that target cell.
Any suggestions?
Thanks,
Bruce
I recommend that you take a different approach. Check all of the entered data regardless of what the target cell is.
That said, here is a first-crack attempt at doing what you asked for. Note the caveats explained in the comments and that I have tested this very briefly.
(However, by the time your hook is called, that target cell may have already been changed.)
August 16, 2018 at 01:26 pm - Permalink
Hi, and thanks for your feedback w/ sample code.
If I understand correctly, you're recommending that the code perform a bounds-check on each element in the wave, each time the return key is pressed. While that would be too slow for long waves, it could work for my application where the waves are likely to be short.
However, that approach wouldn't solve the problem that seems to arise when the user presses 'Enter' immediately after editing the value of a cell. In that case, my (quick) experiments suggest that the wave still contains the old (pre-editing) value, while the table's cell contains the new (post-editing) value. Thus checking the data in the wave appears unreliable as a scheme to immediately catch users' errors.
Please let me know if I should hook a different event to catch such changes, or if I've misunderstood something.
August 20, 2018 at 05:20 pm - Permalink
In reply to Hi, and thanks for your… by BRUCE
I would need to know exactly what you are doing in order to comment. If you will post a minimal, self-contained example that I can run and debug, I will look into this.
August 21, 2018 at 07:15 am - Permalink
That suggests that you are using a window hook function, and perhaps you are using the mouse-down event to catch the edited value. Try the mouse-up event.
August 21, 2018 at 09:44 am - Permalink
I don't see how a table hook helps with this. Below is a simple self-contained example. I get no event when I edit the value of a table cell.
August 21, 2018 at 10:24 am - Permalink
Here is an example using a dependency. It works but I don't know how to get the dependency to run just once when the wave is modified instead of once for every point.
August 21, 2018 at 10:25 am - Permalink
Right. My comment wasn't well thought out; I was thinking of something else.
@BRUCE Are you using a window hook function? If so, better post some code because we can't figure out what you're doing :)
August 21, 2018 at 11:03 am - Permalink
Hi All,
Thanks for the feedback, and sorry for my slow return to this thread.
I've attached a .pxp file that I think will demonstrate the puzzle. Please see the comments at the top of the procedure window for more details.
Looking forward to your feedback,
bp
August 31, 2018 at 11:03 am - Permalink
Ah, I see the problem. You're right- when the Enter key event comes in, the wave hasn't actually been updated. Which is what you told us before :). But it took some digging to uncover the fact that you are using a window hook. Window hooks can be very tricky; in this case I think it simply doesn't give you what you need. Perhaps in a future version?
I think you can, with effort, achieve what you need using a Listbox control. It is difficult because a Listbox deals only in text, not numbers, so you have to prepare a text wave with the contents of the wave you want to edit translated into text.
On the other hand, Listbox controls are made for editing and it's not hard to find out the selection and the new text. Then you can vet the new text before finalizing the value to put into the real numeric wave.
August 31, 2018 at 04:45 pm - Permalink
You might want to look at ModifyTable entryMode. There are commands to check whether values are being edited and not (yet) accepted.
There isn't a way to get the text of the current entry line in Igor until Igor 8.02: ModifyTable entryMode sets S_Value beginning with Igor 8.02.
August 31, 2018 at 06:15 pm - Permalink
In reply to You might want to look at… by JimProuty
You could do it with a dependency if you create a one point dummy dependency wave:
September 1, 2018 at 09:17 am - Permalink
In Igor 8.02, I added two things to help you: Event codes sent when the table entry area is completed or rejected, and a ModifyTable entryMode=num that always sets S_Value to the text in the table entry area.
You can use the event like this:
And you can use the ModifyTable entry mode like this:
September 11, 2018 at 01:02 pm - Permalink
Thanks for the suggestions, and the refinements in Igor v.8.02.
Hope to get back to this question later this week, after which I can give more specific feedback.
bp
September 11, 2018 at 02:59 pm - Permalink
The hints and revisions in v.8.02 seem to solve the puzzle. The attached .ipf file shows the current versions of the table's hook function & the bounds checker (filter).
The new 'tableEntryAccepted' event lets me easily pick up when the user clicks away from the cell they were editing. By detecting that event, the mouse-down event, and a few keydown events, I can now call the filter no matter how the user terminates their edit. The new 'tableEntryCancelled' event doesn't seem necessary (at least for now).
The new assignment of the cell's contents to S_Value lets my filter constrain the user's inputs to permitted values. I'm not sure if there's a way to write the filtered value directly back into the table, but writing the filtered value directly into the wave has seemed adequate so far.
Thanks again for the help,
bp
September 13, 2018 at 04:06 pm - Permalink
Writing a value into a wave displayed in a table is the same thing as writing "directly into the table". If your code does other things after changing the wave, and it's important for the table to immediately show the change in value, you may need to call DoUpdate.
September 13, 2018 at 04:33 pm - Permalink
Will keep that in mind. Thx.
September 13, 2018 at 04:55 pm - Permalink
Here's a very compact example of getting information about an edited table cell using a table hook and the new ModifyTable query:
April 24, 2023 at 02:04 pm - Permalink
Thanks Jim -- that's some customer support!
Hoping to get back to this someday,
Bruce
May 4, 2023 at 01:00 pm - Permalink