
Replace loop over image stack calculations?
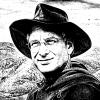
jjweimer
What is the best way to do the calculations below without a for loop?
// assume these two waves exist already make/N=(100,100,200) imgwave= // ... (filled with some image data) make/N=(200)/D ifrac // how do I handle this part without a for loop? variable ic for (ic = 0;ic<200;ic+=1) WaveStats/Q/M=1 imgwave[][][ic] ifrac[ic] = V_sum/(V_npnts*V_max) endfor
For reference, I am computing the fraction of white level in a threshold image going through each plane in a stack.
How about this:
April 4, 2020 at 01:31 am - Permalink
I guess I could have done it without the redimension, but I initially tried to make it work with MatrixOP, and for that the redimension was convenient.
April 4, 2020 at 01:36 am - Permalink
just as example to use MatrixOP without Redimension:
April 4, 2020 at 03:23 am - Permalink
Neat. Thanks!!!
April 4, 2020 at 05:40 am - Permalink