
Add additional submenus
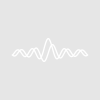
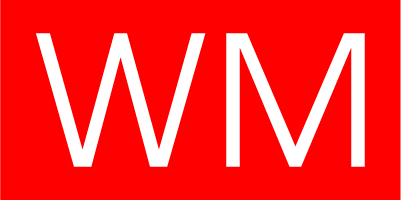
Ben Murphy-Baum
There are many times when I've wanted to programmatically create new submenus within a PopUpContextualMenu, and to allocate items to different submenus. This doesn't seem to be possible right now -- although you can dynamically adjust Menu items, the submenus themselves must be hard-coded into the Menu definition from the start. Instead, I'd like the end user to be able to add submenus to an existing menu definition on the fly using something like AddSubMenu(submenuName,addToMenuName,itemListString).
They can already do this by writing their own menu definition using the same menu name.
Here's an example:
January 26, 2021 at 04:03 pm - Permalink
Hi Jim,
That's definitely useful, but for this case I'm looking more for something where I can have a pre-written program dynamically resolve how many submenus to create, what they should be called, and what items to put into each one.
For my purpose, I'm allowing the user to write their own functions that can be tagged with a submenu assignment in a commented line below the function definition (shown below). I can extract this code as a string using ProcedureText, and I want to resolve the submenu they wish to assign that function to, and then build it into a contextual pop up menu. The idea is to have a menu drop down with all of the user's functions organized as they please into various submenus. Every time a user writes a new function, that's all they have to do--the GUI will automatically find it and parse the functions into their appropriate submenus. Thanks for your help.
January 26, 2021 at 04:29 pm - Permalink
In reply to Hi Jim, That's definitely… by Ben Murphy-Baum
In Jim's example, the menu with the 'dynamic' keyword is built on-the-fly. Instead of Wavelist you can supply an arbitrarily complex string function, with the caveat that adding many menus or using slow text processing will make things sluggish.
But, for what you describe, I don't think the menu needs to be dynamic. Building the menu at compile time will catch any new functions.
For global user functions that take no parameters, this works:
You could supply a wrapper function that executes the selected user function if you need to collect parameters or something.
Use GetLastUserMenuInfo to retrieve the menu selection:
January 27, 2021 at 12:30 am - Permalink
... but I don't think there's an option for programmatically adding or removing submenus, unfortunately.
January 27, 2021 at 05:28 am - Permalink
Give this a try:
If you can live with writing your "ForContext" menu definition to contain a maximum number of submenus (in the example, I made up to 10 possible) then I think you can do what you want.
In your situation you would modify SubmenuStr to handle all of the parsed submenus found in the code.
Note that this works because our menu code will remove any menu items that are dividers if they are not followed by a non-divider menu item. Returning "-" from SubmenuStr makes that submenu a divider.
January 27, 2021 at 06:19 am - Permalink
In reply to Give this a try: Menu … by aclight
Ahh thanks Adam. I had actually tried something like this, but was trying to return an empty string to the submenu definition, which it wasn't allowing. That should solve it!
January 27, 2021 at 09:49 am - Permalink