
V_maxloc with matrixop
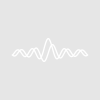
IVP
Hi. Is there a way to get the location of the maximum using matrixop command? I use "maxval" to get the value, but can't find anywhere in the help a way to get the location of this value inside the wave.
Thanks
Here, try this convoluted thing, which should give you the position of maxval in your input wave:
... prints 3 in this test case.
March 26, 2021 at 07:50 am - Permalink
Hi Chozo,
Your code only works for a 1D wave. One can find a convoluted way to get this, for sure. It's just surprising to me that the Igor developers did not include a direct way to find the maxloc/minlod with MatrixOP and was wondering if I am missing it in the help. If this lacks, seems quite a major omission on their part.
In my case I have a matrix of (1e6,10) which correspond (data points, states) and need to know the state with the max value, as well as the overall max. My convoluted way of doing this is:
I broke it into 3 parts, to be easier to follow. However, this takes a lot of time, and need to do this as part of a fitting procedure, which makes things even more complicated.
March 26, 2021 at 09:14 am - Permalink
Hi,
Is there a reason to constrain to matrixop? For example in wavestats, there are V_maxRowLoc, V_maxColLoc,V_maxLayerLoc that will give the location of point.
Andy
March 26, 2021 at 09:24 am - Permalink
In reply to Hi, Is there a reason to… by hegedus
Could you suggest a way using "wavestats" to get a 1D wave with the same number of rows and the values = position of the max in that row. I am trying to avoid "for" loops.
Thanks.
March 26, 2021 at 09:36 am - Permalink
Finding the location of an extrema is not a typical matrix operation; it is supported by WaveStats and ImageStats.
In general, the V_ variables are used in operations that produce unambiguous results. A MatrixOP expression may have arbitrary complexity so the only unambiguous V_ that MatrixOP supports is V_flag which is used to indicate an error.
The case of V_maxRowLoc is a perfect example where the notion of returning a single value is inadequate. Consider, e.g.,
Make/N=(rows,cols) ddd=sin(pi*x/M)
For some values of the constants this will produce multiple maxima and here the various stats operations have to choose one of these values to report back as the location of a maximum.
[... and for advanced users who consider multithreading such code: if two threads return the same maximum value but obviously different max location, which location do you choose?]
In some applications this approach may be good enough but as a general tool it leaves much to be desired. A more appropriate approach consists of two steps:
1. Determine the maximum value.
2. Identify all the locations in the data that contain that value.
Now leave it to the user to pick any subset of these locations for further analysis.
At this point MatrixOP supports maxVal(w), minVal(w) and equal(a,b) so the example above becomes:
MatrixOP/O aa=equal(w,maxVal(w))
A.G.
March 26, 2021 at 10:00 am - Permalink
Dear A.G.,
I am porting a code from Matlab, which has a function: [M,I] = max(A) returns M - the maximum elements of an array A and I is the linear index into A that corresponds to the maximum value in A. I see your point when multithreading, but I am sure you could overcome the rare case when there is more than one maximum if you decide to incorporate this in Igor 9, for example by picking the one with the lowest indexes.
Based on your suggestion, I optimized a bit the code, which now reads:
March 26, 2021 at 11:05 am - Permalink
I'm not a Matlab user so I looked at online documentation for this function. I do not see where/if they specify the returned index in the case of multi-valued maxima.
I am not a fan of the way this is implemented in Igor in stats operations/functions. The difficulty is that we have to keep old code working the same way. In IP9 WaveStats the multi-threaded execution Igor returns the smallest index for which a min/max are found.
The problem with your code is that indexCols() can return a zero or equal() can return a zero. Also, I'd be inclined to not use zero in this way; use NaN instead and zap them to leave only valid indices.
March 26, 2021 at 11:31 am - Permalink