
Insert control action procedure template
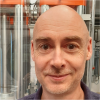
tony
Perhaps there is already a way to do this, but I haven't found it.
menu "Procedure" submenu "Insert control function" "Popup;SetVariable;CheckBox;Button;Listbox;TabControl;Slider;CustomControl", /Q, InsertControlActionCode() end end function /S InsertControlActionCode() GetLastUserMenuInfo string strSav = GetScrapText() string strFunc = "" sprintf strFunc, "function %sProc(STRUCT WM%sAction &s) : %sControl\r\t\r\treturn 0\rend", s_value, s_value, s_value strFunc = ReplaceString("PopupControl",strFunc, "PopupMenuControl") PutScrapText strFunc DoIgorMenu "Edit" "Paste" PutScrapText strSav end
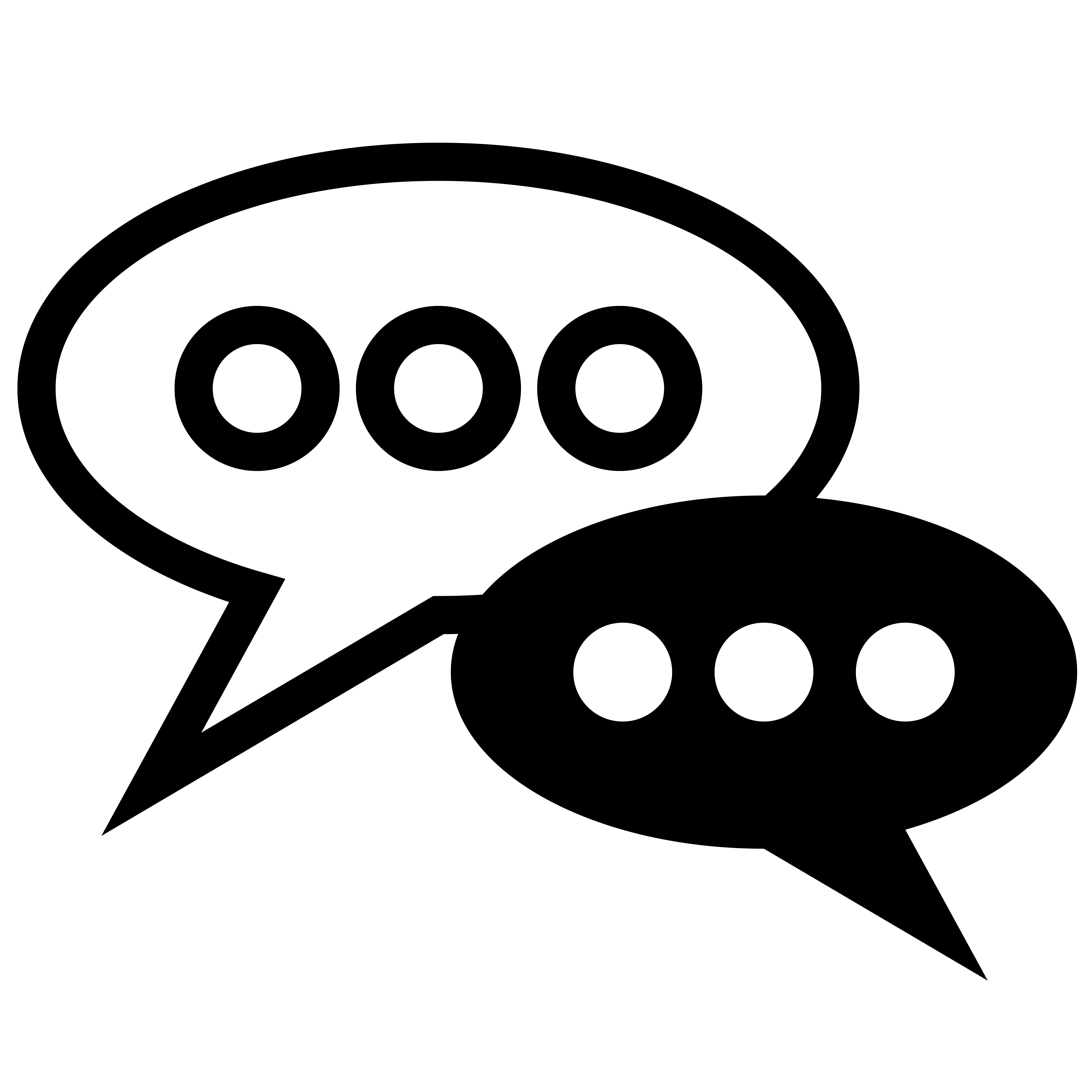
Forum
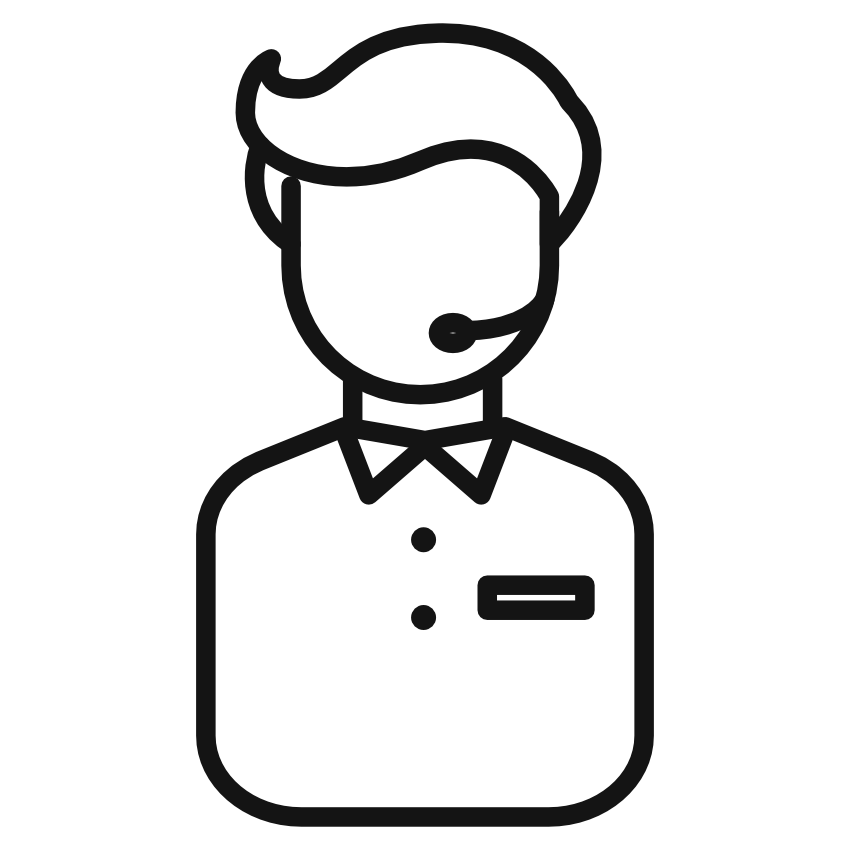
Support
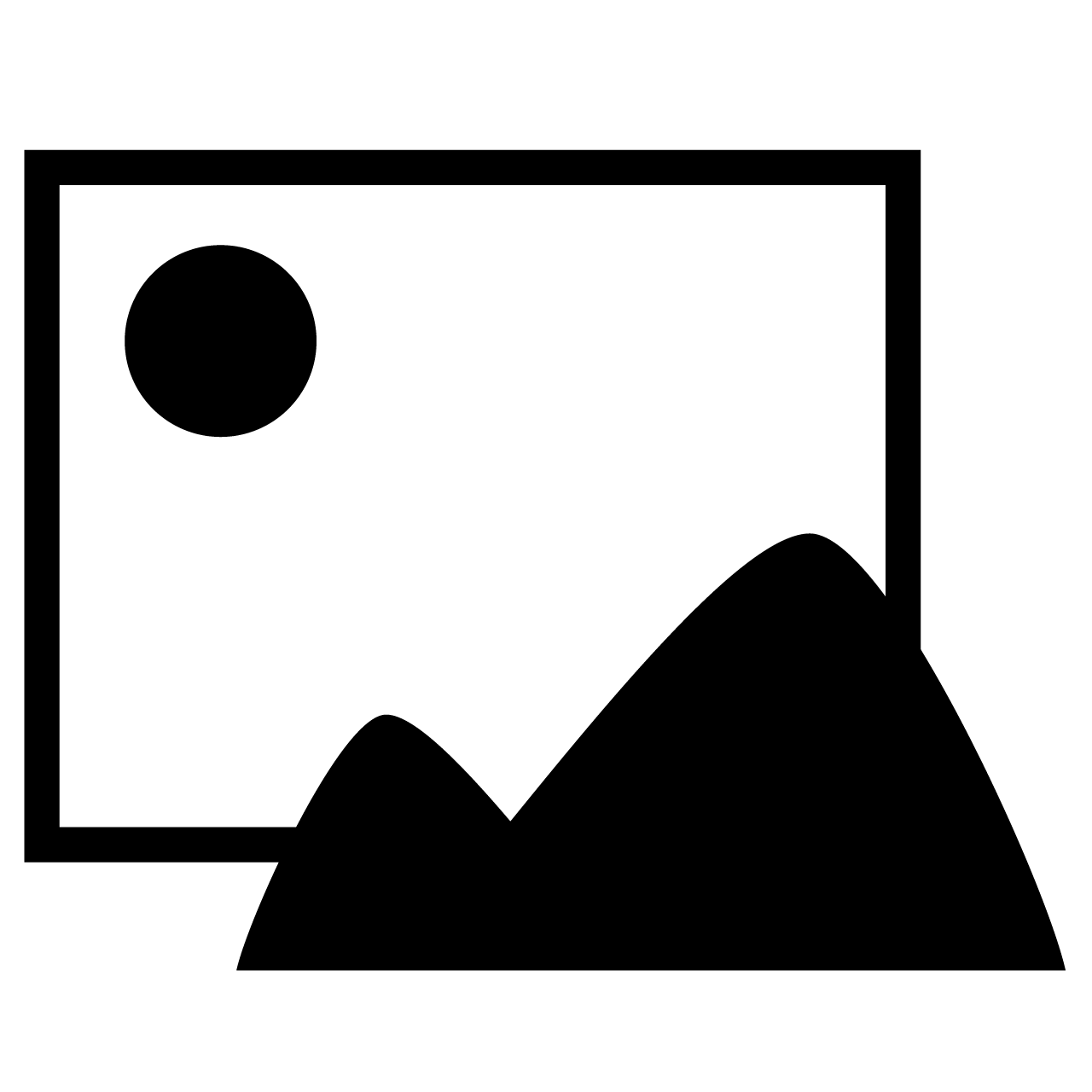
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
Nice, Tony.
I think the ": xxxControl" keyword is missing here. How about this:
Note that the first entry needs "PopupMenu" for the control keyword, hence the replace command.
What really would be awesome and would make this 10x more useful for me would be to add a comment section at the beginning explaining the parameters of the WMxxxAction structure. I constantly have to go to the manual for this to check (I cannot remember the details even after all these years). Do you happen to know how to get the text contents of help files into a string? Then it would be just a matter of filtering out the struct definition at the beginning of each explanation. An alternative approach would be to have a long helper function which would serve and drop in the respective comment. Something like this (I did just the example for the popup menu, since I am lazy today: (EDIT: adjusted the help text a bit.)
If you think this would be useful, then I may complete above function when I have nothing better to do, like on the weekend. ;)
April 23, 2021 at 02:18 am - Permalink
The : xxxControl part is optional, and because I don't usually bother with it I didn't put it in the template. But you're right, I should have included them so that the functions are selectable in the control editing dialogs.
Whenever I write one of these functions I too have to look up the help for the action structure. I have a key mapped to command help - outside of Igor - so that I can place the cursor on the name of the structure and summons the help. Within Igor the keystroke is cmd-option-F1, which I find thoroughly inconvenient.
April 23, 2021 at 03:39 am - Permalink
I should also note that hovering over the structure name will show the structure definition. But the thing I always need is the list of event codes.
April 23, 2021 at 04:18 am - Permalink
Oh, I didn't know that hovering over structures will show their definition, at least for built-in ones (would be cool if this would work for user-defined structures as well). This indeed reduces the problem to mostly eventCodes (and maybe eventMod descriptions). This could be solved by serving comments via above function. I might compile something at post it here later if that is useful.
Yes, the cmd-option-F1 (or here ctrl-alt-F1) keystroke is only something for professional piano players. On top of that I rather read the description within the help browser. I get easily confused with many help windows open.
April 23, 2021 at 05:14 am - Permalink
OK, here is the eventCode / eventMod helper function. Tony, if you find this useful, then would it be a good idea to merge all this into the Text Editing Tools?
April 24, 2021 at 06:45 am - Permalink
Looks good, chozo. I'll wrap this into the Text Edit Tools. I have a few other things I'd like to edit at the same time.
Once cmd-option-fn-F1 (yes, on a macbook pro it takes four keys) has been mapped to a single key, for me it becomes really useful.
April 25, 2021 at 07:51 am - Permalink