
Date time format in delimited text files
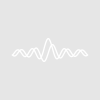
Hello:
Working with a data file from a mil-std-1553 databus monitor application, Alta View. The files are saved as binary files, .cdp extension, but can also be exported to .csv files. Sample of some data from a .csv file is below.
My main challenge time and date formatting. The timestamp is formatted using the year in brackets, julian date in parentheses, followed by system time.
The files can be set to output the data words in hex or decimal, but date/time format doesn't seem to have any other options.
Going back to my request from a few years ago;
https://www.wavemetrics.com/forum/general/help-formatting-datetime-stri…
I'm not sure how much things have changed since Igor 6. The date-time format from those files was a lot different as well.
The format is [year](julian day)hh:mm:ss.ss.??? Not sure what the "extra" decimals are on the ends of the time
Example data:
TimeStamp TimeHigh TimeLow(20nsLSB) IMGap(100nsLSB) CMD1 DATA01
[2020](236)19:35:11.981.685.000 0x0073BBFE 0xDB6BBEDA 0x00000107 0xDCA0 0xF7E3
Some sequential timestamp data:
[2020](236)19:35:11.981.685.000 |
[2020](236)19:35:12.081.805.200 |
[2020](236)19:35:12.181.805.400 |
[2020](236)19:35:12.281.813.480 |
[2020](236)19:35:12.381.689.680 |
[2020](236)19:35:12.481.675.360 |
"[2020](236)19:35:11.981.685.000" is a messy format.
If the file is tab-delimited, then Igor will load "[2020](236)19:35:11.981.685.000" as a text wave and load the remaining columns (0x0073BBFE, 0xDB6BBEDA, 0x00000107, 0xDCA0, 0xF7E3) as numeric. You will then have to parse the text wave using sscanf or Grep.
Here is a function that parses the date/time using sscanf:
May 4, 2021 at 10:26 am - Permalink
you can use sscanf to extract the numbers from the date string:
May 4, 2021 at 10:27 am - Permalink
In reply to you can use sscanf to… by tony
oops, a bit too slow :)
May 4, 2021 at 10:27 am - Permalink