
plotting 2 datasets in one image
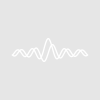
mas1126
Hi all,
I'm looking for a way to plot an image that uses values from two different waves to determine the color output at each x,y point. Specifically, I have two 2D waves- one containing magnitudes, and one containing phase information. I'd like to display them in one image so that the phase determines the hue of the pixel, but the magnitude determines the brightness.
Does anyone have any pointers for accomplishing this?
Make a real-valued 2-D RGB matrix with Make/U/B/O/N=(rows,cols,3) and set the first layer to red, (0-255), second to green, third to blue.
Write a routine to decide what RGB you get from the corresponding point in your 2D complex wave.
As expressed, you need a way to convert from hue and brightness to RGB (constant saturation?)
There are useful routines in #include <colorSpaceConversions>, especially HSL2RGB().
October 4, 2021 at 04:35 pm - Permalink
In reply to Make a real-valued 2-D RGB… by JimProuty
I should look more carefully at the WM utility procedures. I wasted a bit of time rolling my own for several of these functions.
Incidentally, I don't see an RGB2HSL function in colorSpaceConversions. Here's the one I have used. There may be some reason that this is incorrect, perhaps something to do with which colors can be mapped between color spaces in this direction? It worked for my needs.
Also, the RGB2XYZ and RGB2LAB functions are for linearized RGB values, not sRGB. Here is my function with an extra step to 'linearize' standard RGB values.
October 5, 2021 at 03:24 am - Permalink
@Tony:
rgb2hsl is a keyword in ImageTransform that also supports a number of other conversions.
@mas1126:
I'd use Gizmo. Display the first image as a surface object and then use the second wave to create a color wave for the surface object.
Here is a quick example of creating some default surface and then using a function to map the colors.
AG
October 5, 2021 at 12:49 pm - Permalink
Tony brings up a good point: many of our conversions presume a linear RGB color space (because our routines were implemented before sRGB was a thing).
sRGB is a "gamma-compressed" color space:
https://en.wikipedia.org/wiki/SRGB
October 5, 2021 at 04:41 pm - Permalink
yes, colorSpaceConversions.ipf is not self consistent, some functions are for sRGB and some for linear RGB.
Reading this makes me realize that I should post related functions to quantify color difference as a code snippet.
October 6, 2021 at 05:07 am - Permalink