
synchronize time base for multiple waves
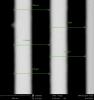
wieliczkad
A recent experiment was run where two datasets were obtained from two different instruments. The instruments ran independently and saved the measured data to separate files. I now would like to plot the output of one dataset versus the values from the second. Since both datasets were one different "clocks" and sampling rates, does anyone have a routine to place both datasets under the same time scale. This would allow me to plot the measured values against each other rather than only versus time.
Hi,
If each data set has regular sampling frequency, you can set the wave scaling for the start and time between each point. Then you can graph the data sets as normal and the scaling will adjust their positions as appropriate.
If the sampling is less regular and you have the time at each data point, then plot the xy pair as appropriate.
If you need to get values for the same time interval from each data set, then I would create a base wave with the time points of interest and interpolate the values from each data set.
Andy
October 6, 2021 at 09:46 am - Permalink
I would like to point out a possible fundamental problem with regard to the two separate instruments. If each reports times derived from its own clock (or sampling base), their clock frequencies may not agree, even if the nominal frequencies are the same. Depending on the sampling rates and your desired accuracy, this could be a problem. In a past data acquisition experiment using Igor Pro, I had to synchronize two PCI cards with a common external clock.
October 6, 2021 at 10:10 am - Permalink
I constantly have to deal with this very problem - time is the worst invention IMHO - my strategy is to create a regular reference time base and expand all the errant data onto that time base.
For example say in your example you have insturent 1 sampling at nominally 1hz but drifting around that value, instrument 2 samples at 5 hz probably also drifting around a bit. Decide what data rate you eventually want your data at - say try 1 hz. You can average data on an uneven time base using this peice of (inefficient) code. Beware a free wave is returned , you could modify it to use a pass-by-refference or however you like:
I probably wouldn't bother averaging your 1hz data, but if there is also no harm in it.
You can then expand the data onto an even time base by first getting the data limits:
Make the even time base, I'm assuminmg the instrument time data is already in Igor time:
Expand out the data onto the regular time wave:
delta would be 0.5 in the case of 1hz data
t1 is the instrument time wave
t2 is the reference regular time wave
w1 is the data to be expanded
Again a free wave is returned, but you could modify that
October 6, 2021 at 12:36 pm - Permalink
In reply to Hi, If each data set has… by hegedus
Andy:
Thank you for the input. I used your idea for interpolation and created new datasets at the same time intervals. This produced the two waves required to plot one wave versus the other.
October 6, 2021 at 01:51 pm - Permalink
In reply to I constantly have to deal… by cpr
Thank you for the code, it will be helpful with the full experiment as I will have multiple waves to apply the routine to. The interpolation routine worked but required redundant effort. Running a procedure multiple times is more straightforward.
October 6, 2021 at 01:55 pm - Permalink