
Thermocouples: temperature to emf (or vice verca)
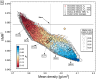
ChrLie
#pragma rtGlobals=1 // Use modern global access method. //The function emf2T converts a thermocouple electromotive force (emf, in milli volts to temperature (in Celsius) or vice verca; //currently for B, S and C-Type thermocouples. All coefficients taken from ASTM E-230. // //syntax: emf2T(TC_string, temp, emf) //Parameters: TC_string is a string indicating the thermocouple type, here B, S or C // tmp is temperature in Celsius // emf is the emf in mV // The value that needs to be calculated must be entered as NaN // //To convert a temperature into an emf value use e.g. print emf2T("B", 1400, nan) //To convert emf to temperature use e.g. print emf2T("B", nan, 8.956) function emf2T(type, Tmp, emf) string type variable Tmp, emf variable c0, c1,c2,c3,c4,c5,c6,c7,c8, c9 c1 = 0 //if not assigned later make sure they are all zero c2 = 0 c3 = 0 c4 = 0 c5 = 0 c6 = 0 c7 = 0 c8 = 0 c9 = 0 // ********************************************************************************************* // *********************** B- Type thermocouples Pt6%Rh / Pt30%Rh ************************* // ********************************************************************************************* strswitch(type) case "B": if (numtype(Tmp) == 0 && numtype(emf) == 2) // this is a temperature to EMF conversion if (tmp >= 0 && tmp <= 630.615) c0 = 0.000000000000E+00 c1 =-0.246508183460E-03 c2 = 0.590404211710E-05 c3 =-0.132579316360E-08 c4 = 0.156682919010E-11 c5 =-0.169445292400E-14 c6 = 0.629903470940E-18 c7 = 0 c8 = 0 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 +c7*tmp^7 +c8*tmp^8 endif if (tmp > 630.615 && tmp <= 1820) c0 = -0.389381686210E+01 c1 = 0.285717474700E-01 c2 =-0.848851047850E-04 c3 = 0.157852801640E-06 c4 =-0.168353448640E-09 c5 = 0.111097940130E-12 c6 =-0.445154310330E-16 c7 = 0.989756408210E-20 c8 =-0.937913302890E-24 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 +c7*tmp^7 +c8*tmp^8 endif endif if (numtype(Tmp) == 2 && numtype(emf) == 0) // this is a EMF to temperature conversion if (emf >= 0.291 && emf <= 2.431) c0 = 9.8423321E+01 c1 = 6.9971500E+02 c2 = -8.4765304E+02 c3 = 1.0052644E+03 c4 = -8.3345952E+02 c5 = 4.5508542E+02 c6 = -1.5523037E+02 c7 = 2.9886750E+01 c8 = -2.4742860E+00 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 endif if (emf > 2.431 && emf <= 13.82) c0 = 2.1315071E+02 c1 = 2.8510504E+02 c2 = -5.2742887E+01 c3 = 9.9160804E+00 c4 = -1.2965303E+00 c5 = 1.1195870E-01 c6 = -6.0625199E-03 c7 = 1.8661696E-04 c8 = -2.4878585E-06 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 endif endif break // ********************************************************************************************* // *********************** S- Type thermocouples Pt / Pt10%Rh ****************************** // ********************************************************************************************* case "S": if (numtype(Tmp) == 0 && numtype(emf) == 2) // this is a temperature to EMF conversion if (tmp >= -50 && tmp <= 1064.18) c0 = 0.000000000000E+00 c1 =0.540313308631E-02 c2 = 0.125934289740E-04 c3 =-0.232477968689E-07 c4 = 0.322028823036E-10 c5 =-0.331465196389E-13 c6 = 0.255744251786E-16 c7 =-0.125068871393E-19 c8 = 0.271443176145E-23 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 +c7*tmp^7 +c8*tmp^8 endif if (tmp > 1064.18 && tmp <= 1664.5) c0 = 0.132900444085E+01 c1 = 0.334509311344E-02 c2 = 0.654805192818E-05 c3 = -0.164856259209E-08 c4 = 0.129989605174E-13 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 +c7*tmp^7 +c8*tmp^8 endif if (tmp > 1664.5 && tmp <= 1768.1) c0 = 0.146628232636E+03 c1 = -0.258430516752E+00 c2 = 0.163693574641E-03 c3 = -0.330439046987E-07 c4 = -0.943223690612E-14 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 +c7*tmp^7 +c8*tmp^8 endif endif if (numtype(Tmp) == 2 && numtype(emf) == 0) // this is a EMF to temperature conversion if (emf >= -0.235 && emf <= 1.874) c0 = 0 c1 = 1.84949460E+02 c2 = -8.00504062E+01 c3 = 1.02237430E+02 c4 = -1.52248592E+02 c5 = 1.88821343E+02 c6 = -1.59085941E+02 c7 = 8.23027880E+01 c8 = -2.34181944E+01 c9 = 2.79786260E+00 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 +c9*emf^9 endif if (emf > 1.874 && emf <=11.950) c0 = 1.291507177E+01 c1 = 1.466298863E+02 c2 = -1.534713402E+01 c3 = 3.145945973 c4 = -4.163257839E-01 c5 = 3.187963771E-02 c6 = -1.291637500E-03 c7 = 2.183475087E-05 c8 = -1.447379511E-07 c9 = 8.211272125E-09 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 +c9*emf^9 endif if (emf > 11.950 && emf <= 17.536) c0 = -8.087801117E+01 c1 = 1.621573104E+02 c2 = -8.536869453E+00 c3 = 4.719686976E-01 c4 = -1.441693666E-02 c5 = 2.081618890E-04 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 endif if (emf > 17.536 && emf <= 18.693) c0 = 5.333875126E+04 c1 = -1.235892298E+04 c2 = 1.092657613E+03 c3 = -4.265693686E+01 c4 = 6.247205420E-01 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 endif endif break // ********************************************************************************************* // *********************** C- Type thermocouples W5%Re / W26%Re ***************************** // ********************************************************************************************* case "C": if (numtype(Tmp) == 0 && numtype(emf) == 2) // this is a temperature to EMF conversion if (tmp >= 0 && tmp <= 630.615) c0 = 0.0000000 c1 = 1.34060323e-2 c2 = 1.19249923e-5 c3 = -7.98063543e-9 c4 = -5.07875153e-12 c5 = 1.31641973e-14 c6 = -7.91973323e-18 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 endif if (tmp > 630.615 && tmp <= 2315) c0 = 4.05288233e-1 c1 = 1.15093553e-2 c2 = 1.56964533e-5 c3 = -1.37044123e-8 c4 = 5.22908733e-12 c5 = -9.20827583e-16 c6 = 4.52451123e-20 return c0*tmp^0 + c1*tmp^1 +c2*tmp^2 +c3*tmp^3 +c4*tmp^4 +c5*tmp^5 +c6*tmp^6 endif endif if (numtype(Tmp) == 2 && numtype(emf) == 0) // this is a EMF to temperature conversion c0 = 0.0 c1 = 7.4124732e1 c2 = -4.28082813 c3 = 5.21138920e-1 c4 = -4.57487201e-2 c5 = 2.80578284e-3 c6 = -1.13145137e-4 c7 = 2.85489684e-6 c8 = -4.07643828e-8 c9 = 2.51358071e-10 return c0 + c1*emf^1 +c2*emf^2 +c3*emf^3 +c4*emf^4 +c5*emf^5 +c6*emf^6 +c7*emf^7 +c8*emf^8 +c9*emf^9 endif break default: break endswitch return NaN // if non of the other if-statements are entered, return NaN end
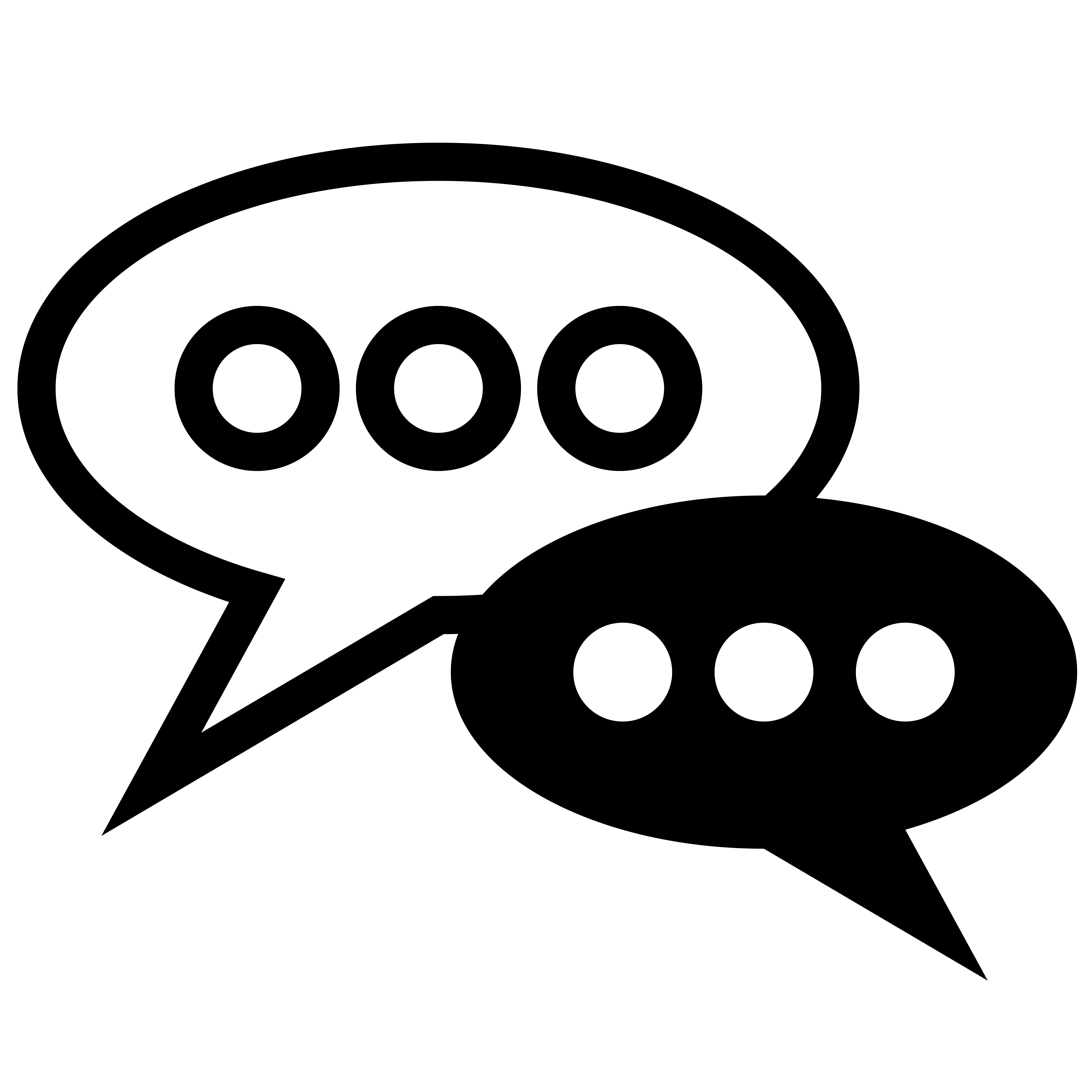
Forum
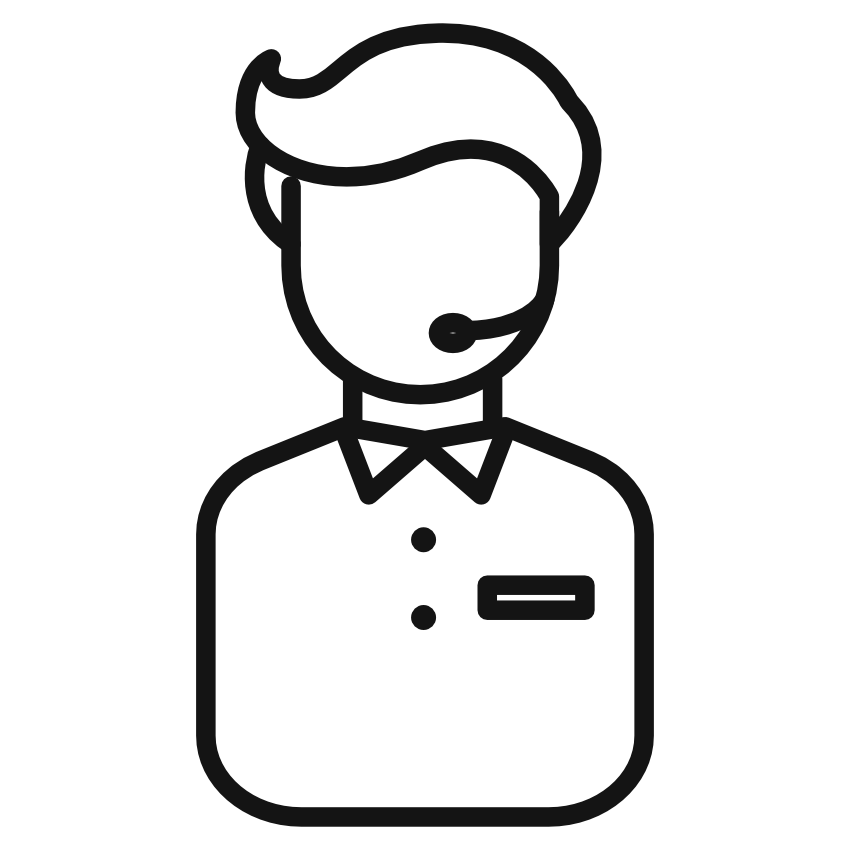
Support
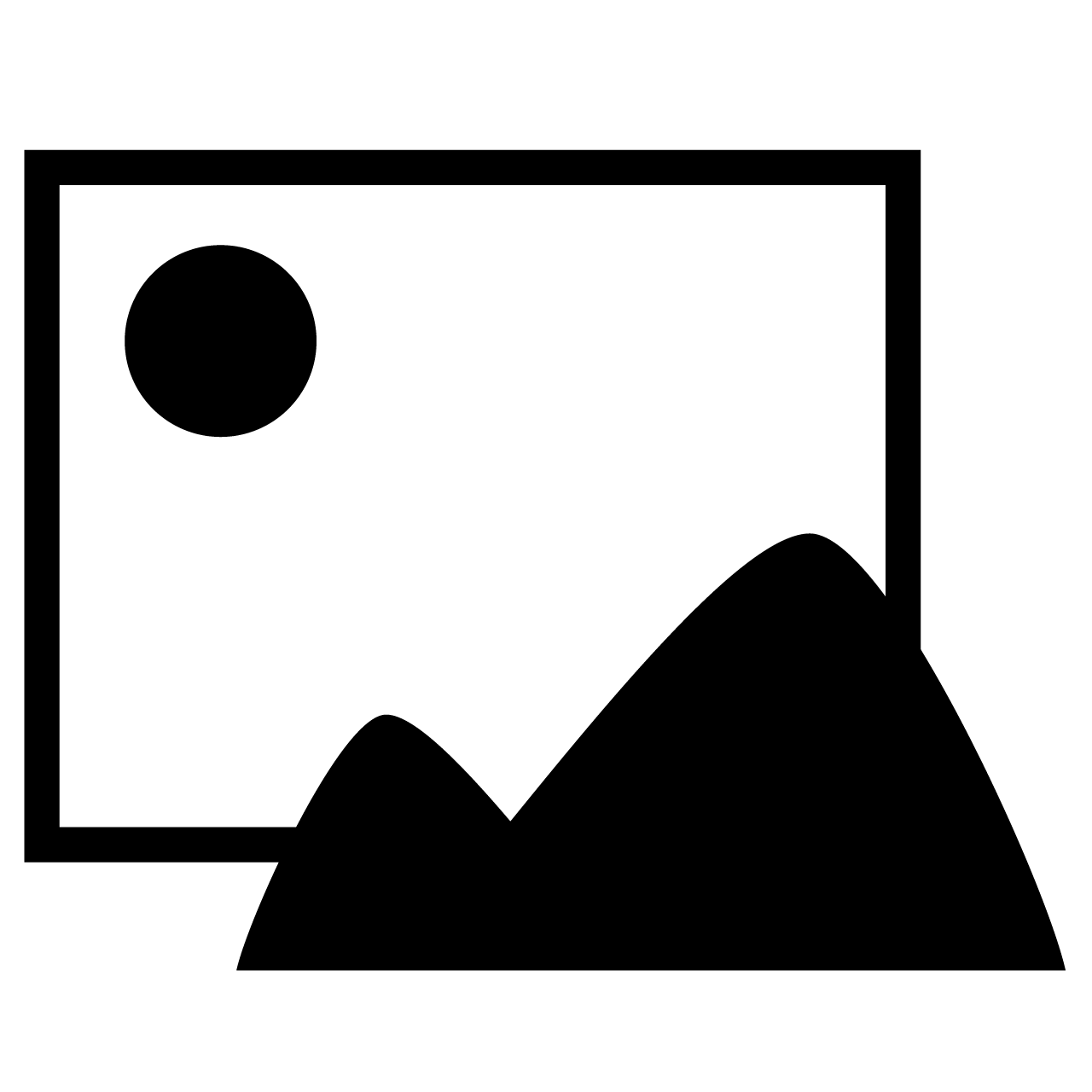
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
I would comment that the straightforward method of evaluating a polynomial has potential problems caused by floating-point truncation errors. A better way to do it is outlined in Numerical Recipes. This method is followed by the Igor poly() function, so I would recommend poly(). To use it, you need to create a wave with the coefficients, something like this fragment that implements just one part of your function:
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
June 1, 2012 at 08:55 am - Permalink
There's a GUI for thermocouple emf calculations here: https://www.wavemetrics.com/node/21411
It does S, B, R, K, T, E, J, N, C, and D types, and includes cold junction compensation. It should convert between emf and temperature in either direction, depending on which setvariable you adjust.
June 27, 2012 at 09:43 am - Permalink
June 29, 2012 at 11:17 am - Permalink
(I haven't been looking here for a while)
yes, indeed! It is all your fault! ;-)
C
July 5, 2012 at 03:06 am - Permalink
August 21, 2012 at 02:31 pm - Permalink
After many years, I have released my thermocouple calulator GUI as an IgorExchange project. I'll update the link in this thread, in case anyone out there is interested in such a thing.
September 24, 2020 at 02:26 am - Permalink