
how to handle changing wave reference in typical commands
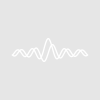
Hello,
Based on my inputs of start and end date of performing data analysis my code extracts the data for individual batches, naming them as batch0, batch1 etc... The number of batches that are extracted depends entirely on the time range I chose to analyze. Therefore, number of individual batches vary every time. Now, I want to do some mathematical operation on the data of each batch but couldn't find a way to automate that using, say for loop. Is there a way to do that?
The way I am extracting data for individual batch is using duplicate function for the parent wave between start and end points and saving them in different waves referenced like this - $nameofthewave(wve) + num2str(i). I am not sure if I could use the same referencing method to say add all positive outcomes in individual batches for all batches.
Any help would be much appreciated!!
Thank you.
Here is an example that may illustrate what you want. It returns a string list of the waves created.
March 14, 2024 at 02:40 pm - Permalink
In reply to Here is an example that may… by jjweimer
I always have trouble to perform arithmetic operations with the waves that are created through some kind of automated process (in this case using a loop).
So, for example, if I would like to add swave_00[5] + swave_03[7] + swave_04[6] + ... what kind of command I use to make it that happen.March 16, 2024 at 12:59 am - Permalink
> ... what kind of command ...?
I would need to see the specifics in your processing to help with anything beyond this.
March 16, 2024 at 07:36 am - Permalink
In reply to > ... what kind of command … by jjweimer
March 16, 2024 at 07:50 am - Permalink
Updating then on my previous post, you might use this as an example ...
March 16, 2024 at 08:41 am - Permalink
I think your question is how to create an arbitrary number of wave references.
Maybe the function that creates the waves can return a wave of type WAVE that contains wave references. As each wave is created you add a reference to the wave of references.
March 18, 2024 at 05:23 am - Permalink
Thank you so much.. I'll try to work on it and let you know...
March 19, 2024 at 08:03 am - Permalink
I tried to work on it but challenged to find a way to do things. Here is the code that I used to create arbitrary number of waves based on the start and endpoints.
By running the code above, I created waves that are named as delta_VOC0, delta_VOC1, delta_VOC2, delta_VOC3..... Now, I would like to check each value in delta_VOC0, delta_VOC1, delta_VOC2, delta_VOC3..... if it is positive or negative. And add positive values and negative values separately for individual wave. I thought to use a for loop to do that, but then depending upon the time range I choose, I might have 100s of delta_VOCs (delta_VOC1, delta_VOC2, delta_VOC3, delta_VOC4, delta_VOC5, delta_VOC6.....), So, I can't do it in a simple way of referencing each delta_VOC wave. I believe I need another for loop where I can change the wave reference (delta_VOC0 then delta_VOC1 then delta_VOC2 then ......) until I cover all delta_VOC waves.
And when I use this automatic referencing method, how to obtain each value or element of the wave, like in normal circumstances, for example, we may use delta_VOC0[7] to read the value of 8th point, but when I have automatic referencing, then what syntax should I use to do that?
March 29, 2024 at 07:21 am - Permalink
In reply to I tried to work on it but… by vpratap397
It's not clear to me what you need here. Could you maybe give a more explicit example of how you would process a pair of time and delta_VOC waves? Do these waves all need to be saved, or are they an intermediate step in some calculation?
Any wave reference that you create is no different to the 'normal' case.
March 31, 2024 at 02:22 am - Permalink
It may be worthwhile reading the help about wave references. Copy these commands, paste them into Igor's command line and press Enter:
DisplayHelpTopic "Wave References"
DisplayHelpTopic "Accessing Waves In Functions"
April 1, 2024 at 09:39 am - Permalink