
Multiplying or dividing a wave by user inputted number
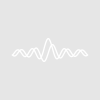
akeyte
Hi, I'm new to writing functions for Igor (and in general). I'd like to be able to multiply and/or divide all the points in a wave by a certain number that I choose when the function is called. For example, I'd like to divide all the points in a wave by 513.
Here is a function I attempted. Ideally I'd like create a new wave without changing the original, but I didn't know how to do that. This function compiles, but doesn't do anything to the wave.
Function DoDivide(inwave,x)
wave inwave
Variable x
inwave /= x
End
Thank you, and I appreciate your help!
Just tried it and it works as anticipated.
0 0
1 0.5
2 1
3 1.5
4 2
5 2.5
6 3
7 3.5
Andy
July 1, 2020 at 08:07 am - Permalink
In reply to Just tried it and it works… by hegedus
Thanks Andy for checking it! I just tried again and yes, it does work.
I'm pleasantly surprised. I spent way too long trying to figure out why it wasn't working!
July 1, 2020 at 08:13 am - Permalink
Have a look at the help for:
NameOfWave, to get the name of the input wave
CheckName and UniqueName, to figure out a good name for the new wave
Duplicate, to make a copy of a wave
and
DisplayHelpTopic "Converting a String into a Reference Using $"
July 1, 2020 at 09:41 am - Permalink
I would like to make a comment on style- since you are still in the process of learning, perhaps I can influence your style! Igor permits you to make a variable called "x" but it is not good practice because x has special meaning in Igor. We must bear much of the blame for this, as for years we published procedure code doing this. But really it's much better to avoid it. You can use a descriptive name like "divisor" and your code becomes self-documenting.
Same goes for the point version of x, "p", and the multidimensional equivalents y,z,t and q,r,s. Sorry for usurping useful letters :)
OK. Down off my pulpit.
July 1, 2020 at 09:49 am - Permalink
EDIT: Ooops, too slow comment, but I hope this one will be of use as well!
For your example you could also write the last line of your function directly into the command line and it would work as well:
test /= 2
Now, if you want to keep the old wave then the Duplicate command is your friend:
This will create a wave named 'result' which then contains the divided values. But it will always be just this one result wave which gets overwritten upon the next call (the /O flag is for this purpose). If you rather want to have a new wave which is similarly named as your original wave then you could do this:
Here a new name for the result wave is defined by a string, which consists of the original wave name and the ending '_div'. This string is then fed into the duplicate and wave assignments. You should read more about the usage of the $ character early on by executing:
DisplayHelpTopic "Converting a String into a Reference Using $"
You will use this a lot in the future! I hope this helps.
July 1, 2020 at 11:48 am - Permalink
In reply to Have a look at the help for:… by tony
Thank you all for your help! I'll have a go at implementing CheckName and UniqueName, but otherwise I'm so happy to have my first function working.
And all comments and feedback on style are definitely helpful. It's good to know!
One thing I'm confused about is the /O flag. For example in Duplicate/O. What does it mean? I haven't been able to find out from the manual.
July 2, 2020 at 07:58 am - Permalink
It means overwrite. If a wave with the name you are using as the new one exists, it will be overwritten with the duplicated wave. Without the /O flag if a pre-existing wave exists, the duplicate operation will throw an error as such.
and from manual:
Duplicate [/O/R=(startX,endX )[(startY, endY )...]] [typeFlags ] srcWaveName, destWaveName [, destWaveName ]...
The Duplicate operation creates new waves; the names of which are specified by destWaveNames and the contents, data type and scaling of which are identical to srcWaveName.
Parameters
srcWaveName must be the name of an existing wave.
The destWaveNames should be wave names not currently in use unless the /O flag is used to overwrite existing waves.
Flags
/FREE Creates a free wave (see Free Waves).
/FREE is allowed only in functions and only if a simple name or wave reference structure field is specified as the destination wave name.
/O Existing waves with the same name as a destWaveName are overwritten.
Andy
July 2, 2020 at 08:26 am - Permalink
In reply to It means overwrite. If a… by hegedus
Thanks Andy for explaining /O so clearly.
I’m completely blown away by how responsive and helpful people are on this forum ?
July 2, 2020 at 09:23 am - Permalink
To see an explanation for all the flags just press F1 and go to the Command Help tab inside the help window. Here you can find a function quickly by typing in the name with quick key strokes while the command list is selected (just typing 'du' should bring you to the Duplicate command). Or you use the right-click menu with the command selected in the procedure window.
July 2, 2020 at 09:40 am - Permalink
It's probably also worthwhile to check this out: DisplayHelpTopic "Operations and Functions Syntax"
It explains that somewhat opaque way we present the command syntax at the top of the reference for a given command.
In general, it is simply a good idea to work through as much of the help (or the PDF manual, which presents the same information) as you can. Especially the Using Igor part of the manual we have tried to present topics in a logical order. One great attribute of the Igor manual: if you read in bed, you will never suffer from insomnia!
July 2, 2020 at 01:15 pm - Permalink
If you're interested in a user interface for dividing waves by numbers, I attach one here.
I made a user interface for this seemingly trivial application because I make mistakes when I type numbers from my lab book on the commandline. A UI helps me to check that I'm entering the right numbers and records the divisor in the wavenote so that I can easily see how a wave has been manipulated during data processing, even if it's loaded into a different Igor experiment.
July 3, 2020 at 01:37 am - Permalink
Thank you chozo for explaining how to find explanations for operations and function. And thanks johnweeks, I'm reading through that section of the manual, and I'll take your suggestion to read the entire thing in bed!
Thank you Tony for sharing your user interface. There's a lot in there that doesn't make sense to me, but that just provides more motivation for me to read the manual :)
July 3, 2020 at 07:54 am - Permalink
Another possibility has occurred to me- we ship with Igor a (rather complicated) procedure file that provides a UI for quite a few different manipulations involving arithmetic on waves. It's called "Wave Arithmetic". There is a demo experiment file that will introduce you to its use. Select File->Example Experiments->Analysis->Wave Arithmetic Panel Demo.
Tony is a very smart fellow- his procedure file may be a better choice.
July 6, 2020 at 10:44 am - Permalink