
Using loop to go through several waves and change the points on those waves with a formula.
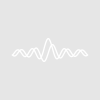
sbrijal90l
I have around 400 waves, wave0, wave1, wave2..., wave400. I want to use a formula to change all the points in those waves.
Since this is my first ever programming in Igor Pro, I began with a small step first. I created a sample wave0, and tried to square the points in that wave 2 times using loop.
Function forloop()
variable i
for (i=0;i<2;i+=1)
wave0=wave0^2
endfor
end
I get the error shown in the image while compiling.
I feel like I am not getting permission to access the wave0. But the same formula works outside the loop.
Please help me with this.
Thanks
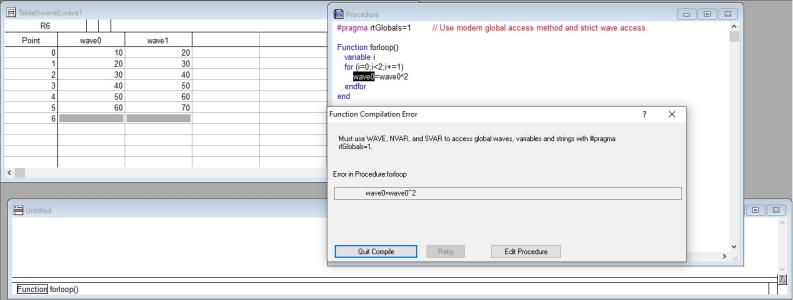
Hi,
If you want to use data (waves, numeric variables, or strings) that lie outside of your function, you need to declare them so Igor knows what specific data you mean to use.
for your simple function, you need to include a declaration of the wave
That step tells Igor to use wave0. For future reference you could also have given it a new name in the declaration. such as
the wave labeled new name only exists within the function and is only a naming device.
Andy
October 27, 2020 at 10:48 am - Permalink
The whole business with WAVE, NVAR and SVAR can be really confusing. It is required because Igor's compiler needs to know what sort of object the name "wave0" refers to in order to compile the right kind of code for it. The compiler runs at a time when wave0 may not exist yet.
To learn more about this, execute this command: DisplayHelpTopic "Accessing Global Variables And Waves"
Just a bit above that help topic is this one: DisplayHelpTopic "Converting a String into a Reference Using $" If you start reading here, you will get to the first one pretty quickly.
Reading the Programming help file is helpful... and if you read it at bed time, it cures insomnia :)
October 27, 2020 at 11:20 am - Permalink
Considering that you want to operate on many different waves, referencing the wave within the function is the thing that allows you to do this, by moving the wave declaration into the loop:
October 28, 2020 at 01:13 am - Permalink
this might be a bit much at the beginning, but here is another way to do the job that doesn't need a loop:
The snippet makes a string list of wave names starting with "wave", generates a matrix from the items in this list, squares it, and then splits the matrix back to individual waves.
Edit: oh, the above assumes that all waves are of equal length!
October 28, 2020 at 02:15 am - Permalink
And we come back to the usual question whether
is faster than
MatrixOP M_AllWaves = magsqr(M_AllWaves)
magSqr(w) Returns a real value wave containing the square of a real w or the squared magnitude of complex w.
October 28, 2020 at 08:03 am - Permalink
@sbrijal90l It should be clear to you by now that you have hit one of the hot topics in Igor programming :)
October 28, 2020 at 10:32 am - Permalink
In reply to And we come back to the… by s.r.chinn
Don't forget WaveTransform :)
October 28, 2020 at 11:30 am - Permalink
In reply to Hi, If you want to use data… by hegedus
Thanks, yes, I missed that and including the declaration compiles it successfully.
October 30, 2020 at 08:48 am - Permalink
In reply to Considering that you want to… by tony
October 30, 2020 at 08:51 am - Permalink
The most common error in a case like this would be that the waves aren't in the current data folder. The best way to look for such errors is using Igor's debugger: DisplayHelpTopic "The Debugger"
And enable debugging on errors: right-click in any procedure window and select Enable Debugger. Right-click again and make sure that Debug on Error and WAVE, NVAR, SVAR Checking is also enabled. Now, you should automatically break into the debugger when such an error occurs, right at the spot in your code that is going wrong.
October 30, 2020 at 10:14 am - Permalink
Oh, and you may want to change #pragma rtglobals=1 to #pragma rtglobals=3, if you are using a sufficiently recent Igor version.
October 30, 2020 at 10:15 am - Permalink
The error you are getting here comes from trying to write 'Function' in the command line. You instead should only write the function's name with () at the end to execute it. The command line does not know what to do with 'Function' here.
October 31, 2020 at 01:53 am - Permalink
In reply to The error you are getting… by chozo
This is just awesome. Thank you so much for pointing out that "MAJOR" flaw with my program learning. I really appreciate that and thanks to everyone helping me solve this.
November 4, 2020 at 10:16 am - Permalink