
how to reverse values of every other column in a big matrix wave ?
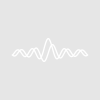
Amo
Hello forum,
I want to reverse values of every other column in waveA, a matrix with 298 columns and 200 rows. Something like in attached image
I know for a single wave, I can use reverse command or use sort /R, but for 298x200 matrix and doing it manually for each column takes forever. So I was wondering if there is an easy way to solve this problem?
Thank you for your help.
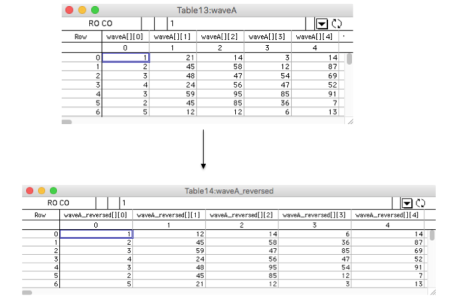
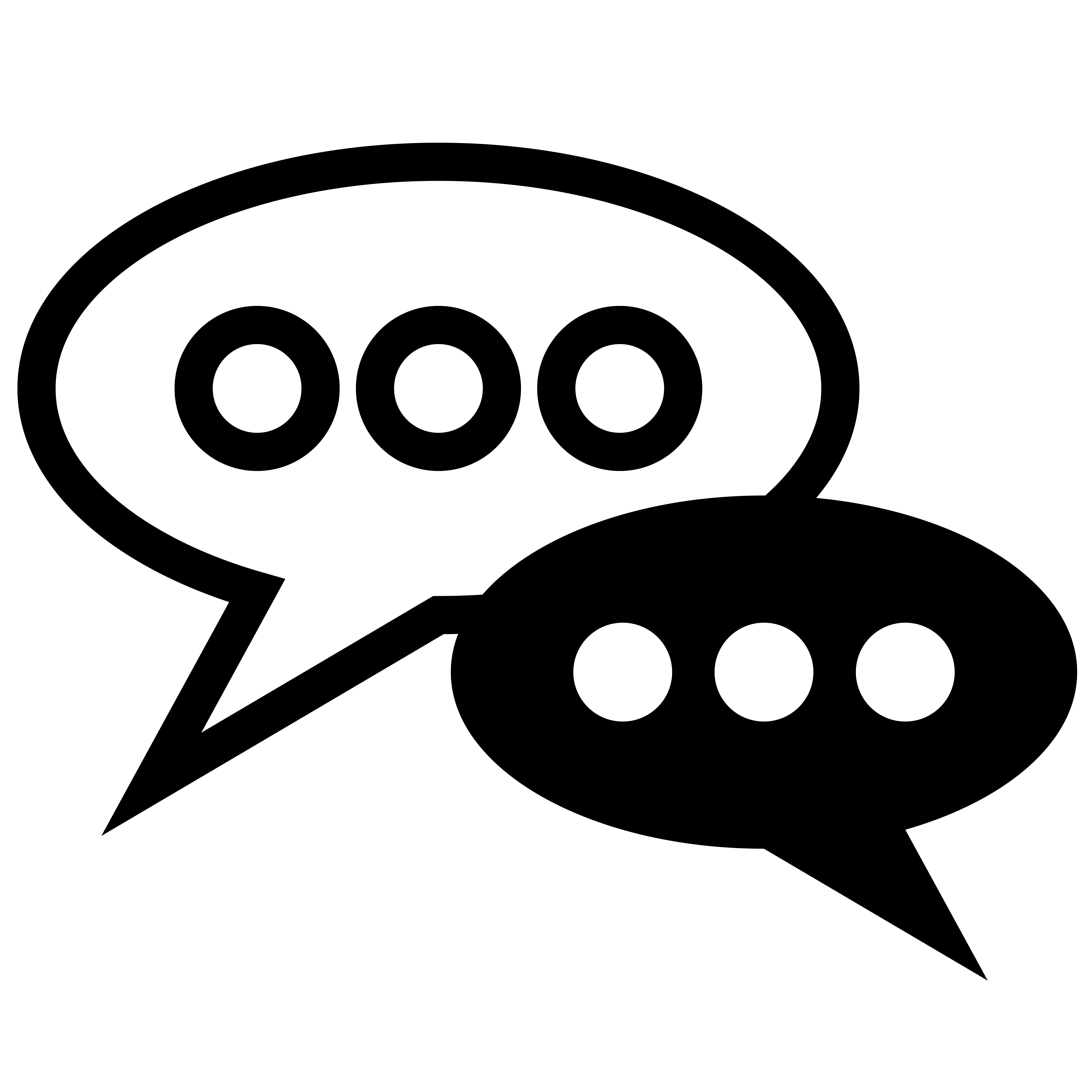
Forum
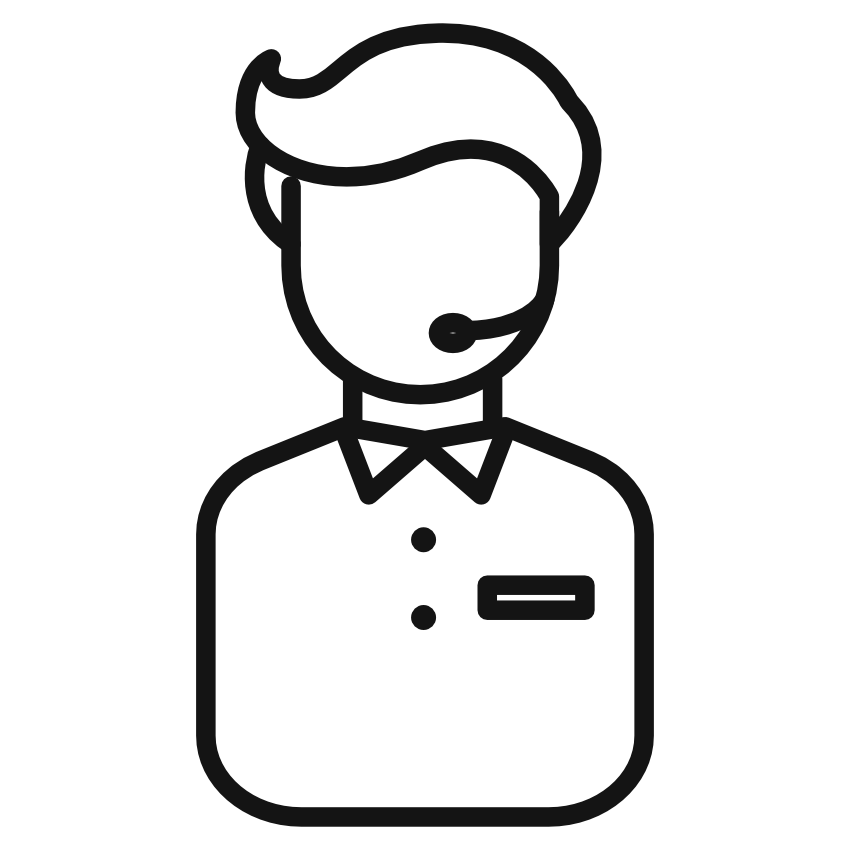
Support
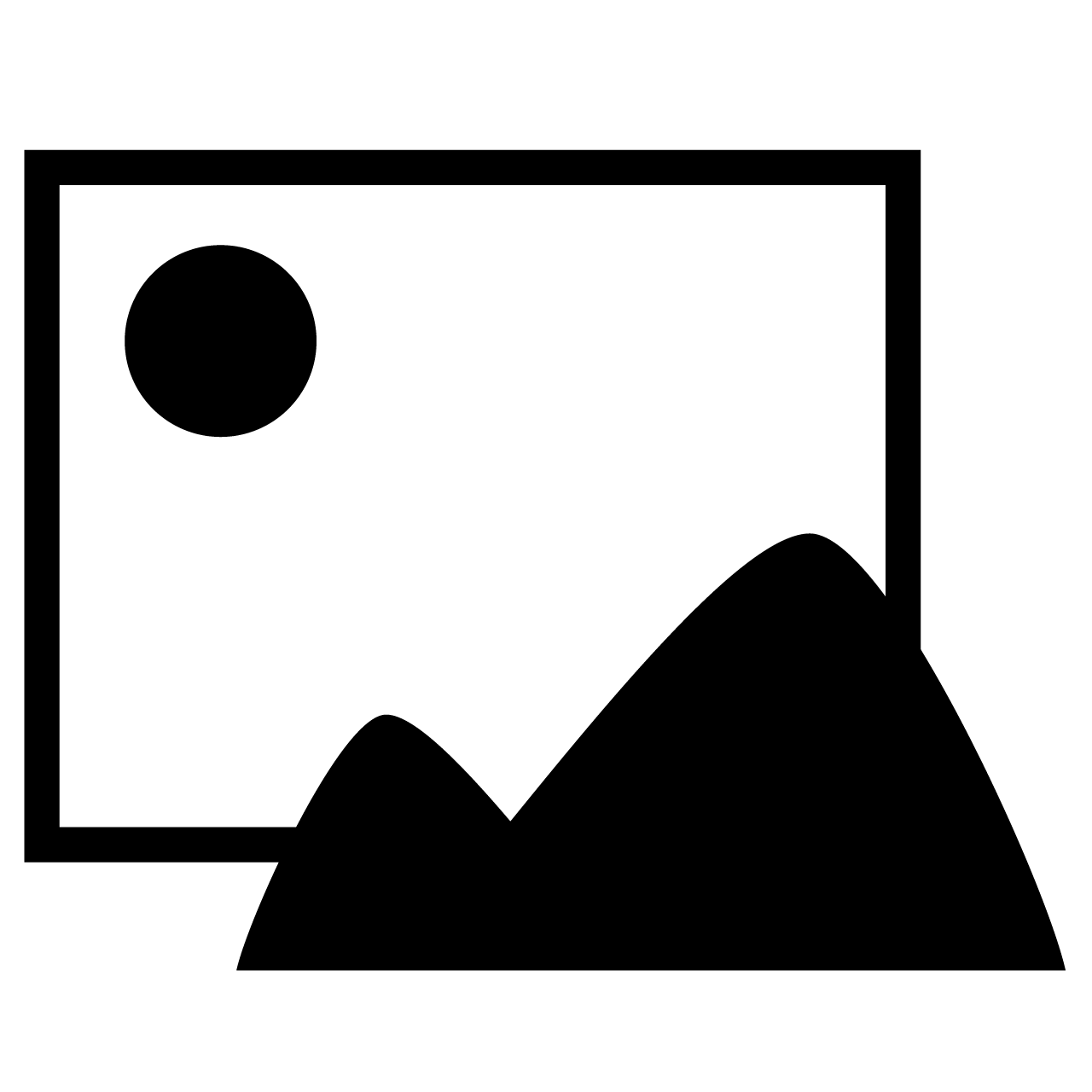
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
Here is a quick solution based on 200 rows, i.e. p goes from 0 to 199.
Duplicate oldwave, newwave
NewWave = mod(q,2)? oldwave[199-p][q] : oldwave[p][q]
Logic: checks to see if column is odd, modulus=1. and then reverses the row assignment. If even, modulus = 0, then just copies.
Andy
April 22, 2019 at 04:32 pm - Permalink
Here is a less-quick solution:
April 22, 2019 at 04:46 pm - Permalink
In reply to Here is a quick solution… by hegedus
this is cool thanks, what does "?" mark in command mean?
April 23, 2019 at 10:10 am - Permalink
In reply to Here is a less-quick… by JimProuty
Really cool , thanks a lot
April 23, 2019 at 10:10 am - Permalink
In reply to this is cool thanks, what… by Amo
From manual
? :
? : is the conditional operator, which is a shorthand form of an if-else-endif statement. It is of the form:
<expression> ? <TRUE> : <FALSE>
When expression is nonzero, it evaluates the TRUE part, otherwise it evaluates the FALSE part. The ":" character in the conditional operator must always be separated with spaces from the two adjacent operands. The operands must be numeric; for strings, you must use the SelectString function.
See Operators for a list of all operators in order of precedence.
Examples
Replace zero values with blanks:
Wave w = wave0
w = w[p]==0 ? NaN : w[p]
Andy
April 23, 2019 at 10:20 am - Permalink
Here is a more-quick solution:
April 25, 2019 at 05:02 am - Permalink
Wow, Steve- you're getting to be almost as creative with MatrixOP as AG is!
April 25, 2019 at 09:17 am - Permalink
Thanks, John. I am but Grasshopper at the feet of the Master :)
Sometimes the effort of Parsing MatrixOP pays off. For the (200 row, 298 col) case cited above, the point-index method gives Profiling result for 1000 iterations:
and identically-looped MatrixOP method gives
April 25, 2019 at 10:06 am - Permalink
In reply to Thanks, John. I am but… by s.r.chinn
Try adding the MultiThread keyword in front of the wave assignment statement in RevOddColsP. Depending on your processor, you might find that to be faster than the MatrixOP version. I did, with a 4 core/8 thread processor.
April 25, 2019 at 11:24 am - Permalink
This would be my approach. I used redimension to separate odd and even columns. I didn't check the speed.
April 25, 2019 at 12:16 pm - Permalink
gives
So it basically depends how many cores which solution is the best. Here I'm having 12.
April 25, 2019 at 02:25 pm - Permalink
In reply to This would be my approach. I… by olelytken
A quick test on my 10-year-old computer gives 0.00014 seconds
April 25, 2019 at 11:29 pm - Permalink
Just to close the loop with Adam's suggesting using MultiThread (in my 4-core cpu), 1000 iterations (divide times by 1000)
and with a more efficient MatrixOp modification
Your mileage may vary (with number of cores, matrix dimensions, etc.)
April 30, 2019 at 03:21 am - Permalink