
Hide characters in a string prompt dialog for secure password entry
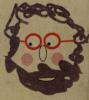
john.geisz
I am prompting the user for a password with a doprompt dialog, but it shows the password in clear text. This is not very secure, so I would like to hide the characters as is commonly done with passwords. I thought of creating a panel with a setvariable control with valueColor the same as valueBackColor, but even that shows the entered characters until the user hits return. Does anyone have any ideas how to prompt the user for a password string while hiding the string from view?
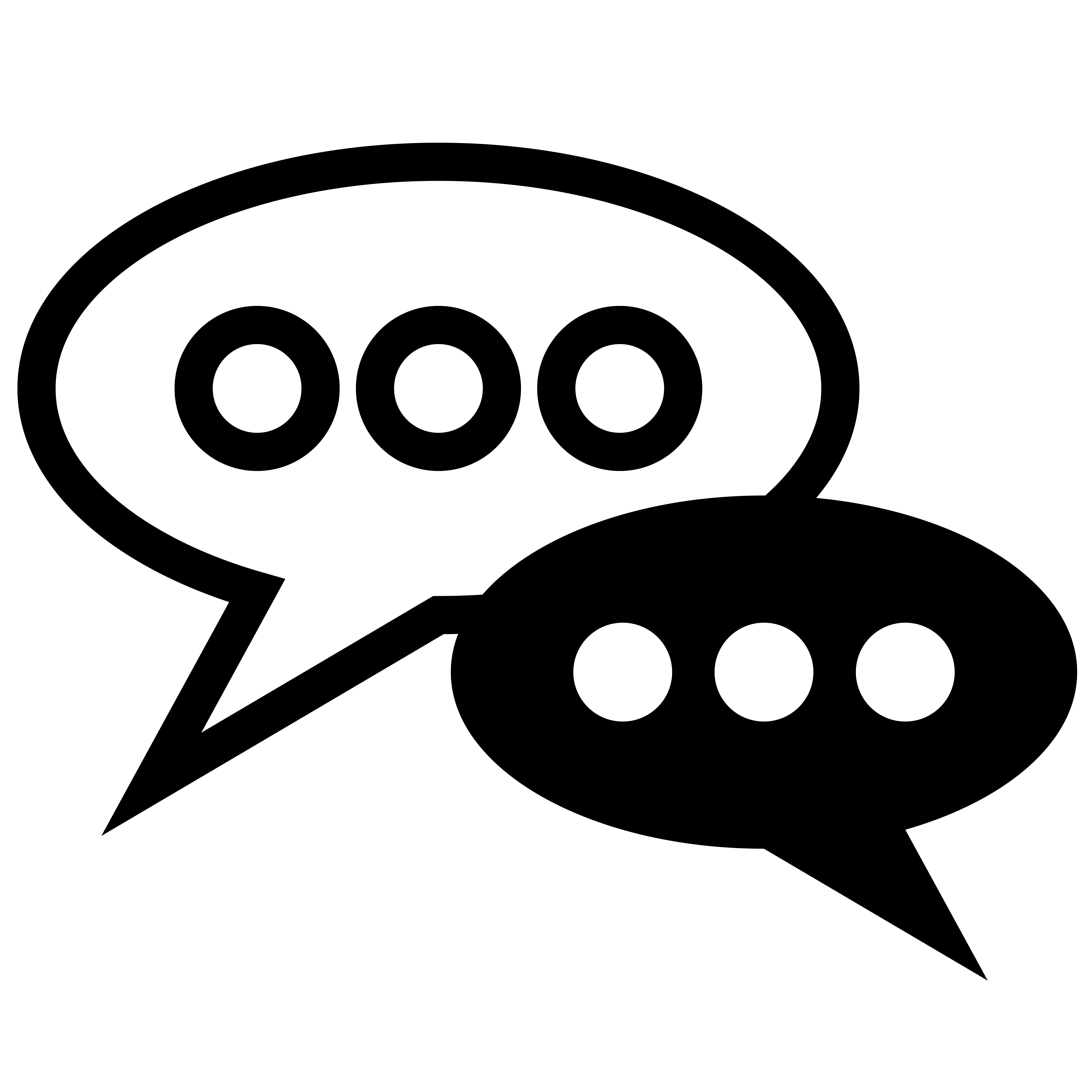
Forum
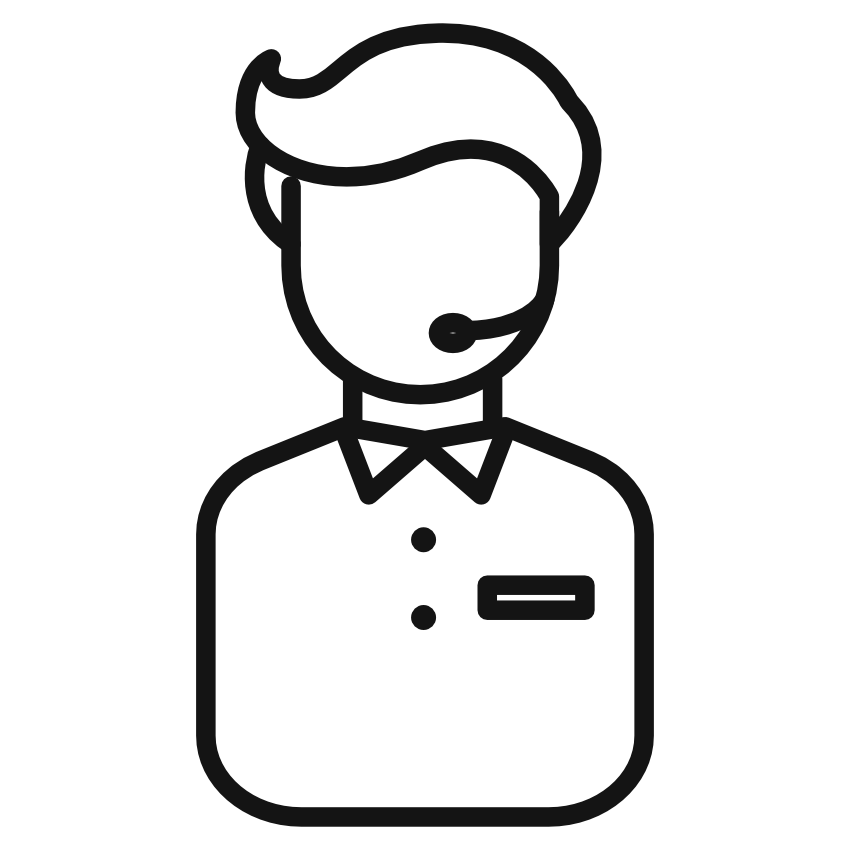
Support
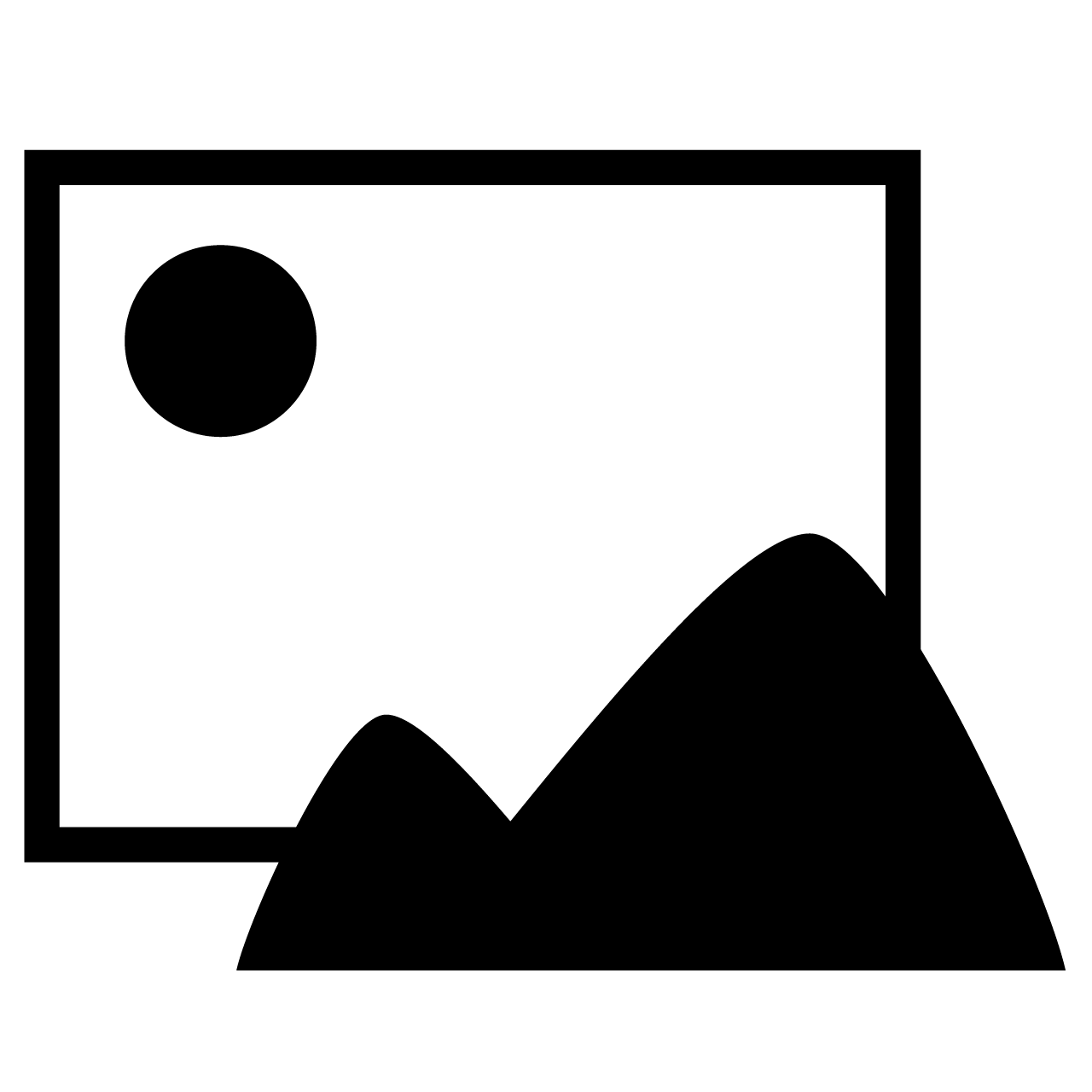
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
specify a font that is all bullets, like PasswordDotsFont:
https://fontstruct.com/fontstructions/show/1106896/password_dots_2
May 2, 2019 at 04:32 pm - Permalink
In reply to specify a font that is all… by JimProuty
That is a good idea, but it creates more problems:
1) How can I change the font of the value of the setvariable control separately from the title?
2) How can I distribute a non-standard font to the users of my procedure easily?
May 2, 2019 at 09:37 pm - Permalink
In reply to That is a good idea, but it… by john.geisz
You could do it with a notebook subwindow. Use a Hook function to process keystrokes in the notebook, grab the typed character for your own use and insert an asterisk in its place.
May 3, 2019 at 02:14 am - Permalink
Here's a code snippet that creates a notebook subwindow and processes user input with a hook function. It doesn't do what you need but could be adapted.
May 3, 2019 at 02:24 am - Permalink
One other method is to capture every keystroke in the SetVariable procedure, store each of them sequentially in an internal string (wave), and only return * (asterisk) to the panel display for any character input. When the keystroke is a return, then process the function call further using the string (wave).
May 3, 2019 at 05:24 am - Permalink
In reply to One other method is to… by jjweimer
Note that there's no eventCode for keyboard events in the WMSetVariableAction structure.
May 3, 2019 at 06:22 am - Permalink
In reply to jjweimer wrote: One… by tony
I believe that I have done this in the past by capturing the sva.sval parameter during an eventCode 3 (live update) and/or eventCode 8 (begin edit Igor Pro 7 or later) and then forcing the control to change back to something else at the event. It is not an easy process to manage but it is a doable process if I recall.
Feature Request: This could be handled by the equivalent of an fstyle=8: password mode for setvariable controls.
May 3, 2019 at 07:15 am - Permalink
Thanks all for the good comments.
I followed Tony's notebook with hook idea.
Here is the code using a dot from the Wingding font.
BEWARE: this example code prints the resulting password (which is hidden as the notebook userdata) as plain text in the history.
May 5, 2019 at 01:08 pm - Permalink
I used a similar approach but used a panel instead of a notebook. I simply hid the SetVariable input by disabling it and putting it off-screen, then temporarily used a global string to pass the password rather than the userdata approach.
May 7, 2019 at 09:02 am - Permalink
In reply to I used a similar approach… by ajleenheer
This is a very nice bit of code!
I suggest changing this line of Passwordpanel():
into:
So that if the user clicks in the control's edit field it doesn't show the typed characters as Wingdings glpyhs:
May 19, 2019 at 07:58 pm - Permalink